Leetcode 2611: Mice and Cheese
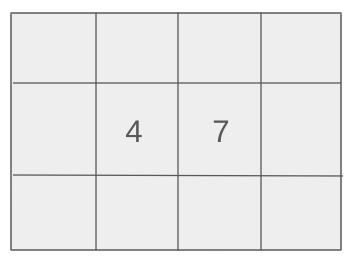
You have two mice and a set of n different types of cheese. Each type of cheese has a different point value when eaten by either mouse. The first mouse must eat exactly k types of cheese, while the second mouse eats the remaining cheese types. Your task is to maximize the total points the two mice can achieve by distributing the cheese types between the mice.
Problem
Approach
Steps
Complexity
Input: You are given two arrays, reward1 and reward2, each of length n, where reward1[i] represents the points the first mouse gets from eating the ith cheese type, and reward2[i] represents the points the second mouse gets from eating the ith cheese type. Additionally, you are given a non-negative integer k, which represents the number of cheese types the first mouse should eat.
Example: reward1 = [2, 5, 3, 4], reward2 = [1, 2, 6, 4], k = 3
Constraints:
• 1 <= n == reward1.length == reward2.length <= 10^5
• 1 <= reward1[i], reward2[i] <= 1000
• 0 <= k <= n
Output: Return the maximum points that the two mice can achieve, considering that the first mouse eats exactly k types of cheese.
Example: Output: 18
Constraints:
• The output will be a single integer representing the maximum points the mice can achieve.
Goal: The goal is to maximize the total points by distributing the cheeses between the two mice, ensuring that the first mouse eats exactly k types of cheese.
Steps:
• Step 1: Calculate the difference in reward between reward1[i] and reward2[i] for each cheese.
• Step 2: Sort the cheeses based on the difference in rewards, from largest to smallest.
• Step 3: Assign the first mouse the k cheeses that provide the highest rewards for it, and assign the remaining cheeses to the second mouse.
• Step 4: Calculate the total points and return it.
Goal: Consider edge cases such as when k is 0 or equal to n, and handle large inputs efficiently.
Steps:
• Arrays reward1 and reward2 will always have the same length.
• The number of cheese types n will always be between 1 and 10^5.
Assumptions:
• The rewards are non-negative integers.
• The first mouse is guaranteed to eat exactly k cheeses.
• Input: reward1 = [2, 5, 3, 4], reward2 = [1, 2, 6, 4], k = 3
• Explanation: The first mouse eats the 2nd, 3rd, and 4th cheeses, giving points 5, 3, and 4. The second mouse eats the 1st cheese, giving 2 points. The total points are 5 + 3 + 4 + 2 = 18.
• Input: reward1 = [1, 1], reward2 = [1, 1], k = 1
• Explanation: In this case, the first mouse eats one cheese, and the second mouse eats the other. The total points will be 1 + 1 = 2.
Approach: The approach focuses on maximizing the reward for the first mouse by sorting the cheese types based on the difference between the rewards of the two mice and assigning the cheeses accordingly.
Observations:
• We need to maximize the reward for the first mouse by selecting k types of cheese that yield the highest reward for it.
• It is important to prioritize the cheeses that give the first mouse the most points while considering the second mouse's reward for the remaining cheeses.
Steps:
• Step 1: Calculate the difference in reward between the two mice for each cheese.
• Step 2: Sort the cheeses based on the reward difference in descending order.
• Step 3: Assign the top k cheeses to the first mouse.
• Step 4: Assign the remaining cheeses to the second mouse.
• Step 5: Sum the points and return the result.
Empty Inputs:
• If reward1 or reward2 is empty, return 0 as no points can be earned.
Large Inputs:
• Ensure that the algorithm efficiently handles the maximum constraint of 10^5 cheese types.
Special Values:
• If k is 0, the first mouse does not eat any cheese, and the second mouse eats all the cheeses.
Constraints:
• Handle cases where all rewards are equal, where the two mice have identical reward values for each cheese.
int miceAndCheese(vector<int>& r1, vector<int>& r2, int k) {
int n = r1.size();
vector<vector<int>> ans;
for(int i = 0; i < n; i++) {
ans.push_back({r1[i] - r2[i], r1[i], r2[i]});
}
sort(ans.begin(), ans.end(), greater<vector<int>>());
int res = 0, i = 0;
while(k--) {
res += ans[i++][1];
}
while(i < n) res += ans[i++][2];
return res;
}
1 : Function Definition
int miceAndCheese(vector<int>& r1, vector<int>& r2, int k) {
Defines the function `miceAndCheese`, which takes two vectors `r1` and `r2` representing the number of cheeses for mice in two locations, and an integer `k` representing the number of mice to select from `r1`.
2 : Variable Declaration
int n = r1.size();
Initializes variable `n` to the size of the input vector `r1`, representing the number of mice.
3 : Vector Initialization
vector<vector<int>> ans;
Declares a 2D vector `ans` to store the difference between `r1[i]` and `r2[i]`, along with the values from `r1[i]` and `r2[i]`.
4 : Loop - Calculation
for(int i = 0; i < n; i++) {
Starts a loop to iterate through each mouse and calculate the difference between the cheese values in `r1[i]` and `r2[i]`.
5 : Push to Vector
ans.push_back({r1[i] - r2[i], r1[i], r2[i]});
Adds a new element to the `ans` vector containing the difference `r1[i] - r2[i]`, followed by `r1[i]` and `r2[i]`.
6 : Sorting
sort(ans.begin(), ans.end(), greater<vector<int>>());
Sorts the vector `ans` in descending order based on the first element of each sub-array (the difference `r1[i] - r2[i]`).
7 : Variable Initialization
int res = 0, i = 0;
Initializes two variables: `res` to accumulate the total cheese and `i` to keep track of the index.
8 : While Loop - Select `k` Mice
while(k--) {
Starts a loop that runs `k` times to add the best `k` mice from `r1` to the result.
9 : Add Cheese from `r1`
res += ans[i++][1];
Adds the cheese value from `r1[i]` to `res` for the current best selection, then increments `i`.
10 : While Loop - Add Remaining Mice
while(i < n) res += ans[i++][2];
Adds the remaining cheese values from `r2[i]` to `res` for the remaining mice.
11 : Return Statement
return res;
Returns the total amount of cheese accumulated after selecting `k` mice from `r1` and the rest from `r2`.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which is O(n log n), where n is the number of cheese types.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because we store the differences in rewards for each cheese type.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus