Leetcode 2601: Prime Subtraction Operation
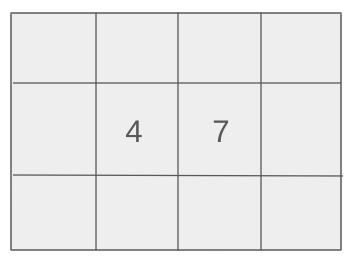
You are given a 0-indexed integer array nums of length n. You can perform the following operation as many times as needed: pick an index i that has not been previously selected, and choose a prime number p such that p < nums[i]. Then, subtract p from nums[i]. Your task is to determine if it is possible to make the array strictly increasing by performing the operation described.
Problem
Approach
Steps
Complexity
Input: The input consists of a list of integers nums, where each element nums[i] represents the value at index i.
Example: nums = [8, 12, 7, 15]
Constraints:
• 1 <= nums.length <= 1000
• 1 <= nums[i] <= 1000
Output: Return true if it is possible to make nums strictly increasing using the described operations; otherwise, return false.
Example: true
Constraints:
Goal: To determine if it's possible to make the array strictly increasing using the prime subtraction operation.
Steps:
• Iterate through the array starting from the second-to-last element.
• For each element nums[i], check if it is greater than or equal to the next element nums[i + 1].
• If nums[i] >= nums[i + 1], find the largest prime number p such that p < nums[i] and subtract it from nums[i].
• Check if the updated nums[i] is strictly less than nums[i + 1]. If not, return false.
• If the array is processed successfully, return true.
Goal: The problem constraints specify that nums will have at least one element and that the values of each element will be between 1 and 1000, inclusive.
Steps:
• 1 <= nums.length <= 1000
• 1 <= nums[i] <= 1000
Assumptions:
• The array can have duplicate elements.
• The prime subtraction operation can be performed any number of times on different elements.
• Input: nums = [8, 12, 7, 15]
• Explanation: We can subtract prime numbers 5 from 8, and 7 from 12 to make the array strictly increasing.
• Input: nums = [5, 6, 10, 12]
• Explanation: The array is already strictly increasing, no operation needed.
• Input: nums = [4, 8, 3]
• Explanation: It is not possible to perform any operation to make this array strictly increasing.
Approach: The solution involves iterating through the array and performing prime subtraction to make the array strictly increasing.
Observations:
• The array must be strictly increasing at every step.
• Prime numbers less than nums[i] can be used to decrease nums[i].
• We need to iterate from the second-to-last element to the first element to perform operations efficiently.
Steps:
• Start with the second-to-last element in the array.
• If the current element is greater than or equal to the next element, subtract the largest prime number smaller than the current element.
• If after subtraction, the current element is still not smaller than the next element, return false.
• Repeat the process for each element in the array.
• Return true if all elements are processed successfully.
Empty Inputs:
• An empty input array should return false as there are no elements to operate on.
Large Inputs:
• The solution should handle input arrays of size up to 1000 efficiently.
Special Values:
• Consider edge cases where nums[i] is 1, and no prime can be subtracted.
Constraints:
• The solution must respect the constraints of 1 <= nums.length <= 1000.
bool primeSubOperation(vector<int>& nums) {
vector<int> arr = { 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71,
73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173,
179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281,
283, 293, 307, 311, 313, 317, 331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409,
419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541,
547, 557, 563, 569, 571, 577, 587, 593, 599, 601, 607, 613, 617, 619, 631, 641, 643, 647, 653, 659,
661, 673, 677, 683, 691, 701, 709, 719, 727, 733, 739, 743, 751, 757, 761, 769, 773, 787, 797, 809,
811, 821, 823, 827, 829, 839, 853, 857, 859, 863, 877, 881, 883, 887, 907, 911, 919, 929, 937, 941,
947, 953, 967, 971, 977, 983, 991, 997 };
int n = nums.size();
for(int i = n - 2; i >= 0; i--) {
int j = 0, tmp = nums[i];
if(nums[i] >= nums[i + 1]) {
int diff = nums[i] - nums[i + 1] + 1;
auto it = lower_bound(arr.begin(), arr.end(), diff);
if(it == arr.end() || *it >= nums[i]) return false;
nums[i] -= *it;
}
if(nums[i] >= nums[i + 1]) return false;
}
return true;
}
1 : Function Definition
bool primeSubOperation(vector<int>& nums) {
Define the function 'primeSubOperation', which takes a reference to a vector of integers and returns a boolean value.
2 : Prime Numbers Initialization
vector<int> arr = { 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317, 331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541, 547, 557, 563, 569, 571, 577, 587, 593, 599, 601, 607, 613, 617, 619, 631, 641, 643, 647, 653, 659, 661, 673, 677, 683, 691, 701, 709, 719, 727, 733, 739, 743, 751, 757, 761, 769, 773, 787, 797, 809, 811, 821, 823, 827, 829, 839, 853, 857, 859, 863, 877, 881, 883, 887, 907, 911, 919, 929, 937, 941, 947, 953, 967, 971, 977, 983, 991, 997 };
Initialize a vector of prime numbers which will be used to compare and subtract from the elements in 'nums'.
3 : Determine List Size
int n = nums.size();
Get the size of the input vector 'nums'.
4 : Loop Initialization
for(int i = n - 2; i >= 0; i--) {
Start a loop that iterates through the vector from second-to-last element down to the first.
5 : Store Temporary Value
int j = 0, tmp = nums[i];
Store the current element in 'tmp' and initialize 'j'.
6 : Check Comparison Condition
if(nums[i] >= nums[i + 1]) {
Check if the current element is greater than or equal to the next element.
7 : Calculate Difference
int diff = nums[i] - nums[i + 1] + 1;
Calculate the difference between the current element and the next element, adding 1.
8 : Find Lower Bound Prime
auto it = lower_bound(arr.begin(), arr.end(), diff);
Find the smallest prime number that is greater than or equal to the calculated difference.
9 : Prime Validation
if(it == arr.end() || *it >= nums[i]) return false;
Check if the found prime number is valid. If not, return false.
10 : Subtract Prime
nums[i] -= *it;
Subtract the found prime from the current element.
11 : Condition Check
if(nums[i] >= nums[i + 1]) return false;
After modification, check again if the current element is greater than or equal to the next element.
12 : Return True
return true;
If the loop finishes successfully, return true.
Best Case: O(n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: In the worst case, for each element, we need to search for a prime number smaller than the element.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space needed for the prime number list and storing the modified array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus