Leetcode 2598: Smallest Missing Non-negative Integer After Operations
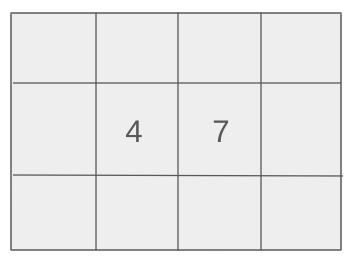
You are given a 0-indexed integer array
nums
and a positive integer value
. In one operation, you can either add or subtract the integer value
from any element of the array nums
. The MEX (Minimum Excluded Value) of an array is the smallest non-negative integer that does not appear in the array. Your task is to determine the maximum possible MEX of the array nums
after performing the mentioned operations any number of times.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `nums` and an integer `value`.
Example: For `nums = [3, -10, 9, 15, 8]` and `value = 4`.
Constraints:
• 1 <= nums.length, value <= 10^5
• -10^9 <= nums[i] <= 10^9
Output: Return the maximum possible MEX of the array `nums` after applying the allowed operations.
Example: For `nums = [3, -10, 9, 15, 8]` and `value = 4`, the output should be `5`.
Constraints:
• The result will be a non-negative integer.
Goal: The goal is to apply the allowed operations to maximize the MEX of the array `nums`.
Steps:
• 1. For each element in `nums`, calculate the possible new values after adding or subtracting `value` any number of times.
• 2. Track the occurrences of all numbers after the operations.
• 3. Find the smallest non-negative integer not present in the array to determine the MEX.
Goal: The constraints ensure the problem is feasible to solve within the given time and space limits.
Steps:
• 1 <= nums.length, value <= 10^5
• -10^9 <= nums[i] <= 10^9
Assumptions:
• The array `nums` contains integers between -10^9 and 10^9.
• The value of `value` is always a positive integer.
• Input: For `nums = [3, -10, 9, 15, 8]` and `value = 4`
• Explanation: By applying the operations as described, you can achieve a maximum MEX of 5.
• Input: For `nums = [1, -15, 5, 14, 7]` and `value = 5`
• Explanation: After applying the allowed operations, the maximum MEX of the array is 3.
Approach: The approach involves tracking the possible new values after applying the allowed operations and determining the MEX of the array.
Observations:
• The operation can add or subtract `value` multiple times.
• The number of possible distinct numbers in the array will be limited by the size of the array and the value of `value`.
• Efficiently track which numbers are present in the modified array.
Steps:
• 1. For each element in `nums`, compute the possible remainders after adding or subtracting `value` multiple times.
• 2. Track the frequency of all possible remainders.
• 3. Find the smallest non-negative integer not represented in the array to determine the MEX.
Empty Inputs:
• The array will always contain at least one element as per the problem constraints.
Large Inputs:
• The solution should handle large arrays efficiently, with `nums.length` up to 10^5.
Special Values:
• If `value` is large relative to the elements in `nums`, fewer operations may be needed.
Constraints:
• Handle negative and large positive values in `nums` efficiently.
int findSmallestInteger(vector<int>& nums, int value) {
map<int, int> mp;
int n = nums.size();
for(int i = 0; i < n; i++) {
mp[(nums[i] < 0? (nums[i] % value + value)%value: nums[i]%value)]++;
}
int idx = 0, mn = INT_MAX;
for(int i = 0; i < value; i++) {
if(mp[i] < mn) {
idx = i;
mn = mp[i];
}
}
return mn * value + idx;
}
1 : Function Definition
int findSmallestInteger(vector<int>& nums, int value) {
Defines the function 'findSmallestInteger' that takes an array of integers 'nums' and an integer 'value' as inputs.
2 : Variable Initialization
map<int, int> mp;
Initializes a map 'mp' that will be used to count the occurrences of the modular results of the elements in 'nums'.
3 : Size Calculation
int n = nums.size();
Calculates the size of the 'nums' vector and stores it in the variable 'n'.
4 : Loop to Populate Map
for(int i = 0; i < n; i++) {
Iterates over each element in the 'nums' vector.
5 : Map Update
mp[(nums[i] < 0? (nums[i] % value + value)%value: nums[i]%value)]++;
Calculates the modular value of each element in 'nums' (adjusting for negative values) and increments its count in the map 'mp'.
6 : Variable Initialization
int idx = 0, mn = INT_MAX;
Initializes two variables, 'idx' to 0 and 'mn' to the maximum possible integer value, to track the smallest count and its index.
7 : Loop to Find Minimum
for(int i = 0; i < value; i++) {
Iterates over the possible modular values (from 0 to value-1).
8 : Condition to Update Min
if(mp[i] < mn) {
Checks if the count for the current modular value is less than the current minimum count.
9 : Update Min Index
idx = i;
Updates the index 'idx' to the current modular value if it has a smaller count.
10 : Update Min Count
mn = mp[i];
Updates the minimum count 'mn' to the count of the current modular value.
11 : Return Result
return mn * value + idx;
Returns the smallest integer formed by multiplying the minimum count 'mn' by the 'value' and adding the index 'idx'.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear in terms of the size of the array `nums`.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is proportional to the number of elements in `nums`.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus