Leetcode 2597: The Number of Beautiful Subsets
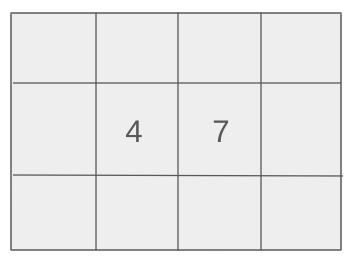
You are given an array of positive integers
nums
and a positive integer k
. A subset of nums
is considered beautiful if it does not contain any two integers whose absolute difference is equal to k
. Your task is to return the number of non-empty beautiful subsets of the array nums
. A subset is formed by deleting some (possibly none) elements from nums
, and two subsets are different if their selected indices are different.Problem
Approach
Steps
Complexity
Input: The input consists of an array `nums` and a positive integer `k`.
Example: For `nums = [3, 5, 7]` and `k = 2`.
Constraints:
• 1 <= nums.length <= 20
• 1 <= nums[i], k <= 1000
Output: Return the number of non-empty beautiful subsets of the array `nums`.
Example: For `nums = [3, 5, 7]` and `k = 2`, the output should be `4`.
Constraints:
• The output will be a positive integer.
Goal: The goal is to find all subsets of `nums` and check if they meet the condition that no two elements have an absolute difference of `k`.
Steps:
• 1. Generate all possible non-empty subsets of the array `nums`.
• 2. For each subset, check if it contains two elements with an absolute difference of `k`.
• 3. Count and return the subsets that do not contain any such pair.
Goal: The constraints ensure that the array size is small enough to allow checking all subsets.
Steps:
• 1 <= nums.length <= 20
• 1 <= nums[i], k <= 1000
Assumptions:
• The array `nums` will contain only positive integers.
• The value of `k` will be a positive integer.
• Input: For `nums = [3, 5, 7]` and `k = 2`
• Explanation: The beautiful subsets are `[3]`, `[5]`, `[7]`, and `[3, 7]`, because none of them contains a pair of numbers with an absolute difference of `2`.
• Input: For `nums = [1, 4, 6]` and `k = 3`
• Explanation: The beautiful subsets are `[1]`, `[4]`, `[6]`, `[1, 4]`, and `[4, 6]` because none contains two elements with an absolute difference of `3`.
Approach: The approach involves generating all non-empty subsets of the array `nums` and checking whether any subset contains two elements with an absolute difference of `k`. If a subset does not contain such a pair, it is counted as a beautiful subset.
Observations:
• The problem requires finding subsets without two elements having a specific difference.
• Given that the array length is small (up to 20), it is feasible to check all subsets.
• Using a recursive approach or dynamic programming could help generate subsets efficiently.
Steps:
• 1. Generate all possible non-empty subsets of `nums`.
• 2. For each subset, check if any two elements have an absolute difference of `k`.
• 3. Count the subsets that do not contain such pairs and return the count.
Empty Inputs:
• There will always be a non-empty input array as per the problem constraints.
Large Inputs:
• The maximum length of `nums` is 20, so the solution must handle up to 2^20 subsets.
Special Values:
• If `k` is greater than the largest difference between any two elements, all subsets will be beautiful.
Constraints:
• The array length is small enough (maximum 20), so checking all subsets is feasible.
vector<int> cnt, nums;
int dp(int idx, int k) {
if(idx == nums.size()) return 1;
int ans = 0;
if(nums[idx] - k >= 0 && (cnt[nums[idx] - k] > 0)) {
ans += dp(idx + 1, k);
} else {
ans += dp(idx + 1, k);
cnt[nums[idx]]++;
ans += dp(idx + 1, k);
cnt[nums[idx]]--;
}
return ans;
}
int beautifulSubsets(vector<int>& nums, int k) {
sort(nums.begin(), nums.end());
cnt.resize(1001, 0);
this->nums = nums;
return dp(0, k) - 1; // exluding none selected
}
1 : Variable Initialization
vector<int> cnt, nums;
Initialize two vectors: `cnt` for counting occurrences of numbers and `nums` for storing the input array.
2 : Function Definition
int dp(int idx, int k) {
Define the recursive function `dp` that takes an index `idx` and a difference `k` to calculate the number of valid subsets.
3 : Base Case
if(idx == nums.size()) return 1;
The base case of the recursion: if the index reaches the end of the array, return 1, indicating a valid subset.
4 : Variable Declaration
int ans = 0;
Declare a variable `ans` to store the count of valid subsets.
5 : Subset Validation
if(nums[idx] - k >= 0 && (cnt[nums[idx] - k] > 0)) {
Check if the current number minus `k` is valid and if the count of that number is greater than 0.
6 : Recursive Call
ans += dp(idx + 1, k);
If the condition is met, make a recursive call to check the next element and accumulate the result in `ans`.
7 : Else Case
} else {
If the condition isn't met, proceed with the following alternative logic.
8 : Recursive Call (No Selection)
ans += dp(idx + 1, k);
First, try the case where the current element is not selected in the subset and make a recursive call.
9 : Increment Count
cnt[nums[idx]]++;
Increment the count of the current element in the `cnt` vector.
10 : Recursive Call (With Selection)
ans += dp(idx + 1, k);
Make a recursive call to consider the current element as part of the subset.
11 : Decrement Count
cnt[nums[idx]]--;
After considering the element in the subset, decrement its count to backtrack.
12 : Return Statement
return ans;
Return the result `ans`, which represents the number of valid subsets found.
13 : Function Definition
int beautifulSubsets(vector<int>& nums, int k) {
Define the main function `beautifulSubsets` that sets up the initial values and calls the `dp` function.
14 : Sort Input
sort(nums.begin(), nums.end());
Sort the input array `nums` to make it easier to handle subsets.
15 : Resize Count Array
cnt.resize(1001, 0);
Resize the `cnt` array to store counts of numbers in the range 0 to 1000, initializing all counts to 0.
16 : Store Input Array
this->nums = nums;
Store the input array `nums` as a member variable of the class.
17 : Return Result
return dp(0, k) - 1; // exluding none selected
Call the `dp` function to compute the number of valid subsets, subtract 1 to exclude the empty subset.
Best Case: O(2^n)
Average Case: O(2^n)
Worst Case: O(2^n)
Description: The time complexity is O(2^n) because we need to check all subsets, where n is the size of the array `nums`.
Best Case: O(2^n)
Worst Case: O(2^n)
Description: The space complexity is O(2^n) because we store all possible subsets of the array `nums`.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus