Leetcode 2594: Minimum Time to Repair Cars
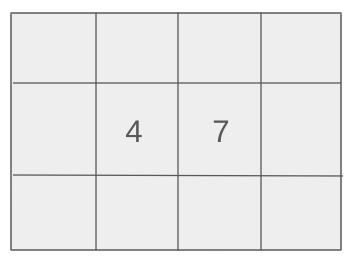
You are given an array
ranks
representing the ranks of some mechanics, where ranks[i]
is the rank of the i
-th mechanic. A mechanic with rank r
can repair n
cars in r * n^2
minutes. You are also given an integer cars
representing the total number of cars waiting to be repaired. Return the minimum time required to repair all the cars when all mechanics can work simultaneously.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `ranks` and an integer `cars`.
Example: For `ranks = [5, 2, 3, 1], cars = 12`.
Constraints:
• 1 <= ranks.length <= 10^5
• 1 <= ranks[i] <= 100
• 1 <= cars <= 10^6
Output: Return the minimum time required to repair all the cars.
Example: For `ranks = [5, 2, 3, 1], cars = 12`, the output is `15`.
Constraints:
• The output is an integer representing the minimum time.
Goal: The goal is to minimize the time taken to repair all the cars by distributing the work efficiently among the mechanics.
Steps:
• 1. Use binary search to find the minimum time required to repair all cars.
• 2. For each possible time, calculate how many cars can be repaired by each mechanic.
• 3. Sum up the number of cars repaired by all mechanics and check if it is sufficient to repair all the cars.
• 4. Adjust the search range to find the minimum time that allows all cars to be repaired.
Goal: The input array `ranks` will have a length between 1 and 10^5. The values in `ranks` will range from 1 to 100. The number of cars will be between 1 and 10^6.
Steps:
• 1 <= ranks.length <= 10^5
• 1 <= ranks[i] <= 100
• 1 <= cars <= 10^6
Assumptions:
• The number of cars is always at least 1.
• There will always be at least one mechanic.
• Input: For `ranks = [5, 2, 3, 1], cars = 12`
• Explanation: By distributing the work among the mechanics, we find the minimum time required is 15 minutes.
Approach: The solution uses binary search to find the minimum time required to repair all the cars by distributing the work efficiently among the mechanics.
Observations:
• The time complexity is dominated by the binary search and the car calculation for each mechanic, which can be optimized.
• The binary search will help narrow down the possible time range efficiently.
Steps:
• 1. Sort the `ranks` array to ensure we start from the mechanic with the lowest rank.
• 2. Apply binary search to find the minimum time required to repair all cars.
• 3. For each midpoint in the binary search, calculate how many cars can be repaired by each mechanic within that time.
• 4. Adjust the search range based on whether all cars can be repaired in the current time.
Empty Inputs:
• There will always be at least one mechanic and one car, so no empty inputs are possible.
Large Inputs:
• The solution should efficiently handle large inputs up to the constraint limits.
Special Values:
• If there is only one mechanic or one car, the solution should still work correctly.
Constraints:
• The solution must handle inputs with up to 100,000 mechanics and 1 million cars.
bool can(vector<int> &ranks, long long time, int cars) {
long long cnt = 0;
if(time == 0) return false;
for(int i = 0; i < ranks.size(); i++) {
cnt += (long long) sqrt(time/ranks[i]);
if(cnt >= cars) return true;
}
return cnt >= cars;
}
long long repairCars(vector<int>& ranks, int cars) {
sort(ranks.begin(), ranks.end());
int mx = ranks[ranks.size() - 1];
long long l = 0, r = LLONG_MAX - 100;
long long ans = r;
while(l <= r) {
long long mid = l + (r - l + 1) / 2;
if(can(ranks, mid, cars)) {
ans= mid;
r = mid - 1;
} else l = mid + 1;
}
return ans;
}
1 : Function Definition
bool can(vector<int> &ranks, long long time, int cars) {
This function checks if it is possible to repair a given number of cars within the specified time, based on the repair ranks provided.
2 : Variable Initialization
long long cnt = 0;
Initializes the counter 'cnt' to track the total number of cars that can be repaired within the given time.
3 : Condition Check
if(time == 0) return false;
If time is zero, no cars can be repaired, so the function returns false.
4 : Loop
for(int i = 0; i < ranks.size(); i++) {
Iterates through each rank to calculate the number of cars that can be repaired in the given time.
5 : Calculation
cnt += (long long) sqrt(time/ranks[i]);
For each rank, it calculates how many cars can be repaired in the given time and adds it to 'cnt'.
6 : Condition Check
if(cnt >= cars) return true;
If the counter reaches or exceeds the number of cars, it returns true, indicating that the repair is possible.
7 : Return Statement
return cnt >= cars;
Returns true if the number of cars repaired is greater than or equal to the target; otherwise, it returns false.
8 : Function Definition
long long repairCars(vector<int>& ranks, int cars) {
Defines the 'repairCars' function, which determines the minimum time required to repair the specified number of cars.
9 : Sorting
sort(ranks.begin(), ranks.end());
Sorts the repair ranks in ascending order to optimize the binary search process.
10 : Maximum Rank
int mx = ranks[ranks.size() - 1];
Determines the maximum repair rank from the sorted ranks.
11 : Variable Initialization
long long l = 0, r = LLONG_MAX - 100;
Initializes the binary search range, starting from 0 to a large value (LLONG_MAX - 100).
12 : Binary Search Setup
long long ans = r;
Sets up a variable 'ans' to store the minimum time required.
13 : Binary Search Loop
while(l <= r) {
Begins the binary search loop.
14 : Middle Point Calculation
long long mid = l + (r - l + 1) / 2;
Calculates the middle point of the current binary search range.
15 : Check Feasibility
if(can(ranks, mid, cars)) {
Calls the 'can' function to check if it's possible to repair the cars in the current time 'mid'.
16 : Update Answer
ans = mid;
If the 'can' function returns true, updates 'ans' to the current time.
17 : Adjust Search Range
r = mid - 1;
If repair is possible within 'mid' time, narrow the search range by adjusting 'r'.
18 : Adjust Search Range
} else l = mid + 1;
If repair is not possible within 'mid' time, adjust the lower bound 'l'.
19 : Return Minimum Time
return ans;
Returns the minimum time required to repair all cars.
Best Case: O(n log T)
Average Case: O(n log T)
Worst Case: O(n log T)
Description: The binary search takes log(T) iterations, and for each iteration, we check the repair capacity of all mechanics, which takes O(n).
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because we store the mechanics' ranks and perform operations on them.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus