Leetcode 2591: Distribute Money to Maximum Children
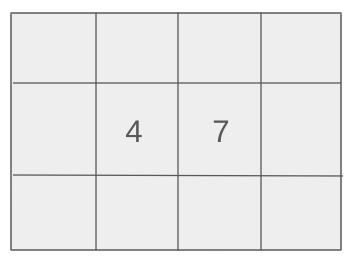
You are given an integer
money
and an integer children
. You need to distribute the money to the children such that everyone gets at least 1 dollar, nobody gets exactly 4 dollars, and you maximize the number of children receiving exactly 8 dollars. Return the maximum number of children who receive 8 dollars, or -1
if it’s not possible.Problem
Approach
Steps
Complexity
Input: The input consists of two integers: `money` (the total amount of money) and `children` (the number of children).
Example: For example, `money = 18, children = 3`.
Constraints:
• 1 <= money <= 200
• 2 <= children <= 30
Output: The output is an integer representing the maximum number of children who can receive exactly 8 dollars, or `-1` if it is not possible to distribute the money accordingly.
Example: For `money = 18, children = 3`, the output is `1`.
Constraints:
• The result will always be a valid integer.
Goal: The goal is to distribute the money optimally such that the maximum number of children receive 8 dollars, considering the constraints of the problem.
Steps:
• 1. Check if it is possible to distribute at least 1 dollar to each child.
• 2. Maximize the number of children who can receive 8 dollars by distributing the remaining money after giving each child 1 dollar.
• 3. Return the number of children who can receive exactly 8 dollars, or return `-1` if it is not possible.
Goal: The problem guarantees that there will be at least 2 children and no more than 30 children. The total money will be between 1 and 200 dollars.
Steps:
• 1 <= money <= 200
• 2 <= children <= 30
Assumptions:
• The input values are valid, and the money can be divided among children according to the given rules.
• Input: For `money = 18, children = 3`
• Explanation: In this example, we give 8 dollars to the first child, 6 dollars to the second child, and the remaining 4 dollars to the third child. Only one child can receive exactly 8 dollars.
Approach: We start by giving each child at least 1 dollar. Then, we maximize the number of children receiving exactly 8 dollars by distributing the remaining money accordingly.
Observations:
• To maximize the number of children receiving 8 dollars, the total money must be sufficiently large to distribute to the children in such a way.
• We need to consider the remainder after distributing the money and check how many children can receive 8 dollars.
Steps:
• 1. If `money` is less than `children`, return -1 as it is not possible to distribute the money.
• 2. Subtract `children` from `money` to ensure each child gets at least 1 dollar.
• 3. Try to give as many children 8 dollars as possible by dividing the remaining money.
Empty Inputs:
• The problem guarantees valid input values and there will be no empty inputs.
Large Inputs:
• The solution must handle cases where `money` is large (up to 200) and `children` is small (up to 30).
Special Values:
• If `money` is exactly equal to `children`, then every child can receive exactly 1 dollar.
Constraints:
• The problem constraints ensure that the number of children and the amount of money will always fall within the specified ranges.
int distMoney(int num, int kid) {
if(num < kid) return -1;
int avg = num / kid;
if(avg > 8) return kid - 1;
if(avg == 8) {
if(num % kid == 0) return kid;
return kid - 1;
}
if(avg > 4) {
num -= kid;
int sol = num / 7;
if(num % 7 == 3 && sol == kid - 1) sol--;
return sol;
}
if(avg == 4) {
num -= kid;
int sol = num / 7;
if(num % 7 == 3 && sol == kid - 1) sol--;
return sol;
}
if(avg < 4) {
num -= kid;
int sol = num / 7;
if(num % 7 == 3 && sol == kid - 1) sol--;
return sol;
}
return -1;
}
1 : Initialization
int distMoney(int num, int kid) {
Function definition for `distMoney`, taking `num` (total money) and `kid` (number of kids) as arguments.
2 : Conditional Check
if(num < kid) return -1;
Checks if there is less money than kids, returns -1 if true.
3 : Average Calculation
int avg = num / kid;
Calculates the average money each kid would get.
4 : Comparison
if(avg > 8) return kid - 1;
Checks if the average is greater than 8; if true, returns the number of kids minus one.
5 : Nested Condition
if(avg == 8) {
Checks if the average is exactly 8.
6 : Division Check
if(num % kid == 0) return kid;
If the money is exactly divisible by the number of kids, all kids receive 8 units.
7 : Else Case
return kid - 1;
If the money is not exactly divisible, return one less than the number of kids.
8 : Condition for Larger Averages
if(avg > 4) {
Checks if the average is greater than 4.
9 : Adjustment
num -= kid;
Reduces the total money by the number of kids, assuming each kid gets at least 1 unit.
10 : Solution Calculation
int sol = num / 7;
Calculates the number of 7 units that can be distributed to the remaining money.
11 : Edge Case Handling
if(num % 7 == 3 && sol == kid - 1) sol--;
Handles a special case when the remaining money modulo 7 equals 3 and the solution is one less than the total kids.
12 : Return Solution
return sol;
Returns the final calculated solution.
13 : Check for avg == 4
if(avg == 4) {
Checks if the average is exactly 4.
14 : Adjustment for avg == 4
num -= kid;
Reduces the total money by the number of kids for the case when the average is 4.
15 : Solution Calculation for avg == 4
int sol = num / 7;
Calculates the number of 7 units that can be distributed to the remaining money when the average is 4.
16 : Edge Case Handling for avg == 4
if(num % 7 == 3 && sol == kid - 1) sol--;
Handles the special case when the remaining money modulo 7 equals 3 and the solution is one less than the total kids.
17 : Return Solution for avg == 4
return sol;
Returns the calculated solution for when the average is 4.
18 : Check for avg < 4
if(avg < 4) {
Checks if the average is less than 4.
19 : Adjustment for avg < 4
num -= kid;
Reduces the total money by the number of kids for the case when the average is less than 4.
20 : Solution Calculation for avg < 4
int sol = num / 7;
Calculates the number of 7 units that can be distributed to the remaining money when the average is less than 4.
21 : Edge Case Handling for avg < 4
if(num % 7 == 3 && sol == kid - 1) sol--;
Handles the special case when the remaining money modulo 7 equals 3 and the solution is one less than the total kids.
22 : Return Solution for avg < 4
return sol;
Returns the calculated solution for when the average is less than 4.
23 : Final Return
return -1;
Returns -1 if no valid distribution is found.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The solution works in constant time as it only involves basic arithmetic operations.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant as no additional space is used apart from the input and output.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus