Leetcode 2587: Rearrange Array to Maximize Prefix Score
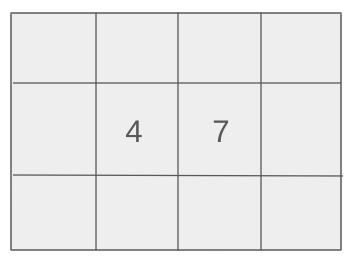
You are given a 0-indexed integer array
nums
. In one operation, you can choose two indices and subtract a power of two from both elements at those indices. A subarray is considered beautiful if you can make all of its elements equal to zero by applying the operation any number of times. Your task is to return the number of beautiful subarrays in nums
.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `nums`.
Example: For example, `nums = [5, 6, 1, 3, 5]`.
Constraints:
• 1 <= nums.length <= 10^5
• 0 <= nums[i] <= 10^6
Output: The output is an integer that represents the number of beautiful subarrays in the array `nums`.
Example: For `nums = [5, 6, 1, 3, 5]`, the output is `3`.
Constraints:
• The result will always be a valid integer.
Goal: The goal is to find the number of subarrays that can be made equal to 0 by applying the specified operations.
Steps:
• 1. Loop through all possible subarrays in `nums`.
• 2. For each subarray, check if it can be transformed into an array of zeros by applying the operations.
• 3. Count the number of such subarrays and return the count.
Goal: The array contains between 1 and 10^5 integers, and each integer is between 0 and 10^6.
Steps:
• 1 <= nums.length <= 10^5
• 0 <= nums[i] <= 10^6
Assumptions:
• The input array contains valid integers within the given range.
• Input: For `nums = [5, 6, 1, 3, 5]`
• Explanation: The beautiful subarrays are `[5, 6, 1]`, `[5, 6, 1, 3, 5]`, and `[6, 1, 3]` as each can become all zeros after applying the operations.
Approach: To solve this problem, we need to find the subarrays where it is possible to make all elements equal to zero by applying the operation any number of times. A strategy would be to check each subarray and verify if it satisfies the condition for becoming a beautiful subarray.
Observations:
• For each subarray, check if it can be made to have all elements equal to zero using the given operations.
• This problem seems to involve checking the binary representation of the numbers and ensuring we can make them equal.
Steps:
• 1. Iterate through each subarray in the given array `nums`.
• 2. For each subarray, attempt to apply the subtraction operation to check if all elements can be reduced to zero.
• 3. Count how many subarrays are beautiful and return the count.
Empty Inputs:
• The problem guarantees that the array will not be empty.
Large Inputs:
• The solution should be optimized to handle large inputs up to 10^5 elements.
Special Values:
• If all numbers are already zero, all subarrays are beautiful.
Constraints:
• The length of the array is guaranteed to be within the specified limits.
int maxScore(vector<int>& nums) {
sort(nums.begin(), nums.end(), greater<int>());
int n = nums.size();
if(n == 1) return nums[0] > 0;
int cnt = 0;
long sum = 0;
for(int i = 0; i < n; i++) {
sum += nums[i];
if(sum > 0) cnt++;
}
return cnt;
}
1 : Function Definition
int maxScore(vector<int>& nums) {
Defines the 'maxScore' function that accepts a vector of integers.
2 : Sorting
sort(nums.begin(), nums.end(), greater<int>());
Sorts the input vector in descending order using the 'greater<int>' comparison function.
3 : Variable Initialization
int n = nums.size();
Stores the size of the sorted vector 'nums' in variable 'n'.
4 : Condition
if(n == 1) return nums[0] > 0;
Checks if the vector has only one element and returns true if that element is positive.
5 : Variable Initialization
int cnt = 0;
Initializes the 'cnt' variable to count how many elements contribute positively to the sum.
6 : Variable Initialization
long sum = 0;
Initializes a long variable 'sum' to keep track of the cumulative sum.
7 : Loop
for(int i = 0; i < n; i++) {
Starts a loop that iterates through each element in the sorted vector 'nums'.
8 : Summing Elements
sum += nums[i];
Adds the current element of 'nums' to the cumulative 'sum'.
9 : Condition
if(sum > 0) cnt++;
If the cumulative sum is positive, increments the 'cnt' variable.
10 : Return
return cnt;
Returns the count of elements that resulted in a positive cumulative sum.
Best Case: O(n)
Average Case: O(n^2)
Worst Case: O(n^3)
Description: In the worst case, we may need to check each subarray, which can lead to a time complexity of O(n^3). Optimizing this solution is essential.
Best Case: O(n)
Worst Case: O(n^2)
Description: The space complexity depends on the storage of subarrays being checked.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus