Leetcode 2580: Count Ways to Group Overlapping Ranges
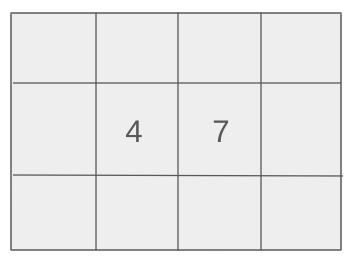
There are
n
people standing in a line labeled from 1 to n
. The first person starts with a pillow. Every second, the person holding the pillow passes it to the next person in line. Once the pillow reaches the last person in the line, it starts moving back in the opposite direction, passing towards the first person. Given n
and time
, return the index of the person holding the pillow after time
seconds.Problem
Approach
Steps
Complexity
Input: The input consists of two integers, `n` (number of people in the line) and `time` (the time in seconds after which we want to determine who holds the pillow).
Example: For example, `n = 4`, `time = 5`.
Constraints:
• 2 <= n <= 1000
• 1 <= time <= 1000
Output: The output is a single integer representing the index of the person holding the pillow after `time` seconds.
Example: For input `n = 4, time = 5`, the output is `2`.
Constraints:
• The result will always be a valid person index in the range from 1 to `n`.
Goal: The goal is to compute the index of the person holding the pillow after `time` seconds, considering the back-and-forth passing mechanism.
Steps:
• 1. Determine the cycle length: the pillow will move back and forth through all the people, so the cycle is `2 * (n - 1)` seconds.
• 2. If the time is less than the cycle length, determine if it's in the forward or backward direction.
• 3. Calculate the index of the person holding the pillow by simulating the movement through the cycle.
Goal: The values for `n` and `time` will always be within the given bounds.
Steps:
• 2 <= n <= 1000
• 1 <= time <= 1000
Assumptions:
• The time will always be a positive integer.
• Input: For `n = 5, time = 7`
• Explanation: The pillow passes from person 1 to person 5, then back to person 4, and stops at person 4 after 7 seconds.
Approach: The approach is to determine the cycle of pillow passing, and based on the time value, compute the position of the pillow. We use a modulo operation to determine the effective time within the cycle.
Observations:
• The pillow follows a repeating back-and-forth pattern.
• Since the cycle is fixed, we can reduce the time into a manageable range using modulo operation.
Steps:
• 1. Calculate the cycle length: `2 * (n - 1)`.
• 2. If the time exceeds the cycle length, reduce it using modulo: `time % cycle_length`.
• 3. Determine the current position by considering the time and the direction of pillow passing.
Empty Inputs:
• No empty inputs are expected; `n` and `time` will always be positive.
Large Inputs:
• The solution should handle large values for `n` and `time` efficiently.
Special Values:
• If `n` is the smallest value (2), the solution should handle it correctly.
Constraints:
• Ensure that `n` and `time` are within the constraints and that the result is correctly calculated.
int countWays(vector<vector<int>>& range) {
sort(range.begin(), range.end());
int n = range.size();
int mod = (int) 1e9 + 7;
int next = range[0][1];
long cnt = 2;
for(int i = 0; i < n; i++) {
if(range[i][0] <= next) {
next = max(next, range[i][1]);
continue;
}
cnt = (cnt * 2) % mod;
next = range[i][1];
}
return cnt;
}
1 : Function Definition
int countWays(vector<vector<int>>& range) {
Define the function `countWays`, which takes a vector of integer vectors `range` as input.
2 : Sorting
sort(range.begin(), range.end());
Sort the `range` vector in ascending order based on the first element of each sub-vector.
3 : Size Initialization
int n = range.size();
Store the size of the `range` vector in the variable `n`.
4 : Modulo Constant
int mod = (int) 1e9 + 7;
Define a constant `mod` with the value `1e9 + 7`, used for modular arithmetic to prevent overflow.
5 : Initialization
int next = range[0][1];
Initialize the `next` variable with the second value of the first range, representing the end of the current interval.
6 : Initialization
long cnt = 2;
Initialize `cnt` to 2, representing the initial number of ways to arrange intervals.
7 : Loop Start
for(int i = 0; i < n; i++) {
Start a loop to iterate over each interval in the `range` vector.
8 : Condition Check
if(range[i][0] <= next) {
Check if the start of the current interval is less than or equal to `next`, indicating overlap or continuity.
9 : Interval Update
next = max(next, range[i][1]);
Update the `next` value to the maximum of the current `next` and the end of the current interval.
10 : Continue
continue;
If the current interval overlaps with the previous one, continue to the next iteration.
11 : Counting
cnt = (cnt * 2) % mod;
If no overlap, multiply the current number of ways `cnt` by 2 and take modulo `mod` to avoid overflow.
12 : Interval Update
next = range[i][1];
Update `next` to the end of the current interval.
13 : Return
return cnt;
Return the final result, which is the number of ways to arrange intervals.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The solution operates in constant time as it only requires modulo and basic arithmetic operations.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant as we only use a few variables.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus