Leetcode 2579: Count Total Number of Colored Cells
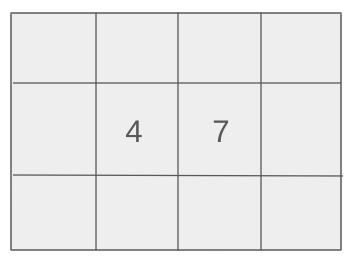
You are given a 2D array
ranges
, where each entry ranges[i] = [starti, endi]
denotes a range of integers between starti
and endi
(inclusive). You need to split the ranges into two groups, ensuring that overlapping ranges belong to the same group. Return the total number of ways to split the ranges into two groups modulo (10^9 + 7).Problem
Approach
Steps
Complexity
Input: The input consists of a 2D array `ranges`, where each element is a pair of integers [starti, endi].
Example: For example, `ranges = [[3, 6], [1, 5]]`.
Constraints:
• 1 <= ranges.length <= 10^5
• 0 <= starti <= endi <= 10^9
Output: The output is an integer representing the total number of ways to split the ranges into two groups modulo (10^9 + 7).
Example: For input `ranges = [[3, 6], [1, 5]]`, the output is `2`.
Constraints:
• The output should be returned modulo (10^9 + 7).
Goal: The goal is to find the total number of ways to split the given ranges into two groups, ensuring that overlapping ranges are in the same group.
Steps:
• 1. Sort the ranges based on the starting value.
• 2. Use a union-find data structure to group overlapping ranges.
• 3. For each group of connected ranges, calculate the possible splits.
• 4. Return the total number of valid ways to split the groups.
Goal: The input will contain a valid list of ranges, and each range has a starting and ending value.
Steps:
• ranges[i].length == 2
• 1 <= ranges.length <= 10^5
• 0 <= starti <= endi <= 10^9
Assumptions:
• The number of ranges can be large, so the solution must be efficient.
• Input: For input `ranges = [[3, 6], [1, 5]]`.
• Explanation: Both ranges overlap, so there are only two possible ways to split them: both in group 1 or both in group 2.
Approach: We will use a union-find (disjoint-set) data structure to identify groups of overlapping ranges and calculate the number of ways to split these groups.
Observations:
• We need to efficiently group overlapping ranges.
• By sorting the ranges and using a union-find structure, we can determine the number of disjoint sets of overlapping ranges.
Steps:
• 1. Sort the ranges based on the start values.
• 2. Use union-find to group the overlapping ranges.
• 3. For each group, there are two choices: group 1 or group 2.
• 4. Multiply the number of ways for each group to get the total number of ways to split the ranges.
Empty Inputs:
• The input will always contain at least one range.
Large Inputs:
• For large inputs, ensure the union-find operations are efficient to handle up to 10^5 ranges.
Special Values:
• If all ranges are disjoint, there are multiple ways to split them.
Constraints:
• Handle the case where all ranges overlap or none of them overlap.
long long coloredCells(int n) {
long long x = 1;
long long y = 4;
while(--n) {
x += y;
y += 4;
}
// 0 4 8 12
return x;
queue<pair<int, int>> q;
unordered_map<int, unordered_map<int, int>> mp;
long long cnt = 1;
q.push({0,0});
mp[0][0] = true;
int dir[] = {0,1,0,-1,0};
while(--n) {
int sz = q.size();
while(sz--) {
auto it = q.front();
q.pop();
// cout << it.first << " " << it.second << "\n";
for(int i = 0; i < 4; i++) {
int x = it.first + dir[i], y = it.second + dir[i + 1];
if(mp.count(x) && mp[x].count(y)) continue;
mp[x][y] = true;
cnt++;
q.push({x, y});
}
}
}
return cnt;
}
1 : Function Definition
long long coloredCells(int n) {
Define a function to calculate the number of colored cells for a given value of n.
2 : Variable Initialization
long long x = 1;
Initialize variable x to represent the initial colored cells count.
3 : Variable Initialization
long long y = 4;
Initialize variable y for the number of colored cells in subsequent rows.
4 : Looping
while(--n) {
Start a loop that continues until n is reduced to zero.
5 : Cell Calculation
x += y;
Add the current value of y to x, incrementing the count of colored cells.
6 : Cell Calculation
y += 4;
Increase y by 4 to account for the new number of cells in the next row.
7 : Return Statement
return x;
Return the final value of x, which represents the total number of colored cells.
8 : Queue Initialization
queue<pair<int, int>> q;
Initialize a queue to store the coordinates of the cells to be processed.
9 : Map Initialization
unordered_map<int, unordered_map<int, int>> mp;
Initialize a map to track the visited cells, ensuring no repeats in processing.
10 : Counter Initialization
long long cnt = 1;
Initialize the counter variable cnt to 1, accounting for the starting cell.
11 : Queue Push
q.push({0,0});
Push the starting cell coordinates (0, 0) into the queue.
12 : Map Setup
mp[0][0] = true;
Mark the starting cell (0, 0) as visited in the map.
13 : Direction Setup
int dir[] = {0,1,0,-1,0};
Define an array of directions to move in the grid (right, down, left, up).
14 : Looping
while(--n) {
Start a loop to process each level of BFS.
15 : Queue Size
int sz = q.size();
Store the current size of the queue to process each element at this level.
16 : Inner Loop
while(sz--) {
Loop through the current level of BFS by processing the elements in the queue.
17 : Queue Processing
auto it = q.front();
Get the front element from the queue for processing.
18 : Queue Pop
q.pop();
Remove the front element from the queue after processing it.
19 : Direction Loop
for(int i = 0; i < 4; i++) {
Loop through all four possible movement directions (right, down, left, up).
20 : Coordinate Calculation
int x = it.first + dir[i], y = it.second + dir[i + 1];
Calculate the new coordinates after moving in the current direction.
21 : Map Check
if(mp.count(x) && mp[x].count(y)) continue;
Check if the new coordinates have already been visited. If so, continue to the next direction.
22 : Mark Visited
mp[x][y] = true;
Mark the new coordinates as visited in the map.
23 : Increment Counter
cnt++;
Increment the counter to reflect the newly visited cell.
24 : Queue Push
q.push({x, y});
Push the new coordinates into the queue for further processing.
25 : Return Statement
return cnt;
Return the final count of the visited cells.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, where n is the number of ranges.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) for storing the ranges and union-find structures.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus