Leetcode 2578: Split With Minimum Sum
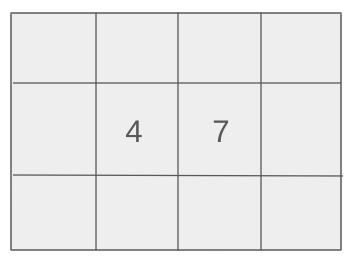
Given a positive integer num, split it into two non-negative integers num1 and num2 such that the concatenation of num1 and num2 is a permutation of the digits of num. The goal is to minimize the sum of num1 and num2.
Problem
Approach
Steps
Complexity
Input: The input consists of a positive integer num.
Example: For example, num = 8657.
Constraints:
• 10 <= num <= 10^9
Output: The output is an integer, the minimum possible sum of num1 and num2 after splitting the digits of num.
Example: For num = 592, the output is 56.
Constraints:
• The output is an integer representing the minimal possible sum.
Goal: The goal is to find the minimum sum after splitting the digits of num into two valid numbers.
Steps:
• 1. Extract the digits of num.
• 2. Sort the digits in ascending order to minimize the sum.
• 3. Distribute the digits alternatively between num1 and num2 to form two valid numbers.
• 4. Compute and return the sum of num1 and num2.
Goal: The input number num is guaranteed to be between 10 and 10^9.
Steps:
• 10 <= num <= 10^9
Assumptions:
• The number num will always have at least two digits.
• Input: For num = 592, the output is 56.
• Explanation: We split 592 into num1 = 5 and num2 = 9. The sum of 5 and 9 is 56, which is the minimum possible sum.
Approach: We approach this problem by first extracting and sorting the digits of num. Then, we distribute the digits between num1 and num2 in an alternating manner to ensure the minimal sum.
Observations:
• The digits need to be rearranged optimally to form the smallest possible sum.
• Sorting the digits in ascending order and then distributing them alternately between num1 and num2 should give the minimal sum.
Steps:
• 1. Convert num into an array of digits.
• 2. Sort the array of digits.
• 3. Distribute the digits into two numbers by alternating between num1 and num2.
• 4. Return the sum of num1 and num2.
Empty Inputs:
• The input will always be a valid integer with at least two digits.
Large Inputs:
• For large inputs (up to 10^9), ensure the algorithm handles large integers efficiently.
Special Values:
• If all digits in num are the same, num1 and num2 should be split equally to minimize the sum.
Constraints:
• Ensure that the final sum is the minimal possible value.
int splitNum(int num) {
int num1 = 0, num2 = 0, x = 1;
vector<int> v;
while(num){
v.push_back(num%10);
num /= 10;
}
sort(v.begin(),v.end());
num = 0;
for(auto &i: v){
num *= 10;
num += i;
}
while(num){
num1 += x*(num%10);
num /= 10;
num2 += x*(num%10);
num /= 10;
x *= 10;
}
return num1+num2;
}
1 : Code Block
int splitNum(int num) {
Function declaration: Starts the splitNum function with an integer input.
2 : Variable Initialization
int num1 = 0, num2 = 0, x = 1;
Initializes variables num1, num2 for storing results, and x for scaling the digits during the second loop.
3 : Variable Initialization
vector<int> v;
Creates a vector 'v' to store individual digits of the number.
4 : Loop
while(num){
Begins a loop that continues as long as there are digits in the number.
5 : Action
v.push_back(num%10);
Extracts the last digit of 'num' and adds it to the vector 'v'.
6 : Action
num /= 10;
Divides 'num' by 10 to remove the last digit.
7 : Action
sort(v.begin(),v.end());
Sorts the vector 'v' containing digits in ascending order.
8 : Variable Initialization
num = 0;
Resets 'num' to 0 before processing the sorted digits.
9 : Loop
for(auto &i: v){
Starts a loop to process each digit in the sorted vector.
10 : Action
num *= 10;
Multiplies 'num' by 10 to shift left by one digit.
11 : Action
num += i;
Adds the current digit 'i' from the sorted vector to 'num'.
12 : Loop
while(num){
Starts a loop to process the number with sorted digits.
13 : Action
num1 += x*(num%10);
Extracts the last digit of 'num' and adds it to 'num1' scaled by 'x'.
14 : Action
num /= 10;
Divides 'num' by 10 to remove the last digit.
15 : Action
num2 += x*(num%10);
Extracts the last digit of 'num' again and adds it to 'num2' scaled by 'x'.
16 : Action
num /= 10;
Divides 'num' by 10 again to remove the last digit.
17 : Action
x *= 10;
Multiplies 'x' by 10 to scale the next digit in the next iteration.
18 : Return
return num1+num2;
Returns the sum of 'num1' and 'num2' as the result of the function.
Best Case: O(d log d)
Average Case: O(d log d)
Worst Case: O(d log d)
Description: The time complexity is dominated by the sorting step, where d is the number of digits in num.
Best Case: O(d)
Worst Case: O(d)
Description: The space complexity is O(d) due to the storage of the digits of num.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus