Leetcode 2571: Minimum Operations to Reduce an Integer to 0
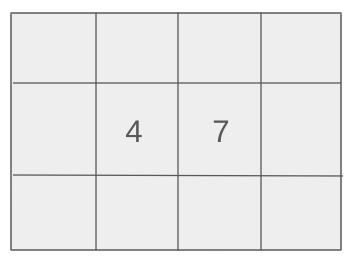
You are given a positive integer n. You can perform the following operation any number of times: Add or subtract a power of 2 from n. Your goal is to find the minimum number of operations required to make n equal to 0.
Problem
Approach
Steps
Complexity
Input: The input consists of a positive integer n.
Example: For example, n = 15.
Constraints:
• 1 <= n <= 10^5
Output: Return the minimum number of operations required to make n equal to 0.
Example: For n = 15, the output is 4.
Constraints:
• The result must be an integer representing the minimum number of operations.
Goal: The goal is to minimize the number of operations required to reduce the number n to 0 by adding or subtracting powers of 2.
Steps:
• 1. Use dynamic programming (or recursion) to evaluate the minimum number of operations needed to reach 0.
• 2. In each operation, subtract or add the largest power of 2 that is less than or equal to n.
Goal: The input n is a positive integer and is bounded by 1 <= n <= 10^5.
Steps:
• 1 <= n <= 100000
Assumptions:
• n is always a positive integer.
• Input: For n = 15, the output is 4.
• Explanation: We subtract powers of 2 in the following steps: 15 - 8 = 7, 7 - 4 = 3, 3 - 2 = 1, 1 - 1 = 0. Thus, we need 4 operations.
Approach: The approach uses dynamic programming or recursion to break down the problem. By analyzing how powers of 2 can be added or subtracted, we can compute the minimum number of steps required.
Observations:
• Powers of 2 (such as 1, 2, 4, 8, etc.) are the key to solving the problem efficiently.
• Dynamic programming allows us to minimize operations by storing intermediate results to avoid recomputation.
Steps:
• 1. If n is zero, return 0.
• 2. If n is odd, subtract the largest power of 2 and recursively solve for the remaining n.
• 3. If n is even, simply shift it right and recursively solve.
Empty Inputs:
• n will always be positive as per the problem constraints.
Large Inputs:
• Ensure that the solution can handle n up to 10^5 efficiently.
Special Values:
• For n = 1, the answer is 1 since it's already a power of 2.
Constraints:
• The operations must be minimized, making an efficient approach necessary.
int dp(int n) {
if(n == 0) return 0;
// cout << n << " " ;
int ans = 0;
if((n & 1) == 0) {
ans = dp(n >> 1);
} else {
if(((n >> 1) & 1)== 1) {
// if(n == 5) cout << "'" << n<< "'";
n += 1;
// while((n & 1) == 0) n >>= 1;
ans = 1 + dp(n);
} else {
ans = 1 + dp(n >> 1);
}
}
return ans;
}
int minOperations(int n) {
// aggregate consecutive ones
// turn off one
return dp(n);
}
1 : Function Declaration
int dp(int n) {
Declares the function `dp`, which takes an integer `n` and recursively calculates the minimum number of operations to reduce it to 0.
2 : Base Case
if(n == 0) return 0;
Base case: If `n` is 0, return 0 as no operations are needed.
3 : Variable Initialization
int ans = 0;
Initialize a variable `ans` to store the result of the recursive operations.
4 : Condition Check
if((n & 1) == 0) {
Check if the least significant bit of `n` is 0 (i.e., `n` is even).
5 : Recursive Call (Even Case)
ans = dp(n >> 1);
If `n` is even, recursively call `dp` with `n` right-shifted by 1 bit (equivalent to dividing by 2).
6 : Else Block
} else {
If `n` is odd, enter the else block.
7 : Condition Check (Odd Case)
if(((n >> 1) & 1) == 1) {
Check if the second least significant bit of `n` is 1 (i.e., the number is odd and the next bit is also set).
8 : Increment n
n += 1;
If the next least significant bit is 1, increment `n` by 1 to make it even, which will facilitate further bit manipulation.
9 : Recursive Call (Odd Case)
ans = 1 + dp(n);
If `n` is odd, increment the operation count by 1 and recursively call `dp` on the modified `n`.
10 : Else Block (Even Case)
} else {
If the second least significant bit is not set (i.e., `n` is an odd number with the next bit cleared), enter this block.
11 : Recursive Call (Even Case)
ans = 1 + dp(n >> 1);
If the second least significant bit is 0, right-shift `n` by 1 and recursively calculate the number of operations.
12 : Return Statement
return ans;
Return the calculated result `ans`.
13 : Function Declaration
int minOperations(int n) {
Defines the `minOperations` function that calculates the minimum number of operations to reduce `n` to 0 by calling `dp`.
14 : Return Statement
return dp(n);
Call the `dp` function with `n` and return the result.
Best Case: O(log n), where n is the input value, due to the logarithmic nature of powers of 2.
Average Case: O(log n), assuming the recursion depth is bounded by log n.
Worst Case: O(log n), as the problem is based on powers of 2 and can be solved within log n steps.
Description: The time complexity is logarithmic with respect to the input n, as each operation reduces n significantly.
Best Case: O(log n), if using recursion or memoization.
Worst Case: O(log n), due to the depth of recursion or the space needed for dynamic programming.
Description: The space complexity is logarithmic because of the recursive calls or memoization used to store intermediate results.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus