Leetcode 2568: Minimum Impossible OR
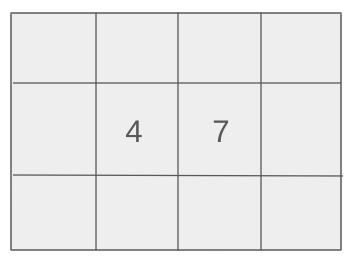
You are given a 0-indexed integer array nums. A number x is expressible from nums if there exists a subsequence of elements in nums whose bitwise OR equals x. Your task is to return the smallest positive integer that cannot be expressed as the bitwise OR of any subsequence from nums.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer array nums, where each element represents a number.
Example: For example, nums = [1, 2, 4].
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
Output: Return the smallest positive integer that cannot be expressed as the bitwise OR of any subsequence from nums.
Example: For nums = [1, 2, 4], the output is 8.
Constraints:
• The result must be a positive integer that cannot be formed by the OR operation of any subsequence of the input array.
Goal: To find the smallest positive integer that cannot be expressed as the bitwise OR of any subsequence from nums.
Steps:
• 1. Initialize a set to store the unique OR values of subsequences.
• 2. Traverse the nums array and compute the bitwise OR for all possible subsequences.
• 3. Check which positive integer is not part of the computed values, starting from 1 upwards.
Goal: The length of the nums array is between 1 and 100,000, and each integer in nums lies between 1 and 10^9.
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
Assumptions:
• The nums array will always contain at least one element.
• The integers in nums are positive and within the specified range.
• Input: For nums = [1, 2, 4], we can express 1, 2, 3 (from 1 | 2), 4, 5 (from 1 | 4), 6 (from 2 | 4), and 7 (from 1 | 2 | 4).
• Explanation: The smallest integer that cannot be expressed is 8.
• Input: For nums = [7, 3, 1], we can express 1, 3, 7, 4 (from 1 | 3), and 6 (from 3 | 7).
• Explanation: The smallest integer that cannot be expressed is 8.
Approach: The approach is based on finding all possible bitwise OR values from subsequences of the array and identifying the smallest integer that cannot be formed.
Observations:
• Bitwise OR is cumulative, so we can track which values can be formed from any subsequence.
• Start with a small integer (1) and check whether it is expressible. If not, that is the smallest non-expressible integer.
Steps:
• 1. Use a set to track which integers can be formed from bitwise ORs.
• 2. Sort the array to make the OR computations efficient.
• 3. Check for the smallest missing positive integer from the set of OR results.
Empty Inputs:
• Empty input is not possible since nums contains at least one integer.
Large Inputs:
• The solution should be efficient enough to handle large inputs, up to 100,000 elements.
Special Values:
• If all elements are powers of two, it is possible to express every positive integer from 1 up to the sum of all the powers of two.
Constraints:
• Ensure the solution can handle arrays with large values efficiently.
int minImpossibleOR(vector<int>& nums) {
set<int> cnt;
int n = nums.size();
for(int i = 0; i < n; i++) {
cnt.insert(nums[i]);
}
int ret = 1;
while(cnt.count(ret)) {
ret *= 2;
}
// to create all the numbers till a positive integer
// presents of all the powers of 2s below it are mandatory.
return ret;
}
1 : Function Declaration
int minImpossibleOR(vector<int>& nums) {
Declares the function `minImpossibleOR` which takes a vector `nums` and returns an integer, representing the smallest impossible OR value.
2 : Set Initialization
set<int> cnt;
Declares a set `cnt` to store unique elements from the array, ensuring no duplicates are included.
3 : Array Size Calculation
int n = nums.size();
Calculates the size of the input array `nums` and stores it in variable `n`.
4 : Loop
for(int i = 0; i < n; i++) {
Begins a loop to iterate through the entire array `nums`.
5 : Set Insertion
cnt.insert(nums[i]);
Inserts each element of the array `nums` into the set `cnt`. This ensures that only unique elements are considered.
6 : Variable Initialization
int ret = 1;
Initializes the variable `ret` with a value of `1`, representing the smallest possible result.
7 : Loop
while(cnt.count(ret)) {
Starts a loop that continues while the set `cnt` contains the current value of `ret`.
8 : Multiplication
ret *= 2;
Doubles the value of `ret` in each iteration, ensuring that `ret` is a power of 2.
9 : Return Statement
return ret;
Returns the value of `ret`, which is the smallest positive integer that is not present in the set `cnt`.
Best Case: O(n log n), where n is the number of elements in nums.
Average Case: O(n log n), dominated by the sorting step.
Worst Case: O(n log n), as all subsequences need to be considered.
Description: The sorting operation dominates the time complexity.
Best Case: O(n), in case we store all possible OR results.
Worst Case: O(n), where n is the number of elements in nums.
Description: Space complexity is driven by the storage required for the nums array and intermediate OR results.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus