Leetcode 2558: Take Gifts From the Richest Pile
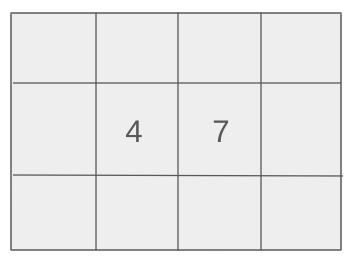
You are given an integer array
gifts
where each element represents the number of gifts in different piles. Every second, you pick the pile with the most gifts and leave behind the floor of the square root of the number of gifts. Repeat this operation for k
seconds, and return the total number of gifts left in all piles.Problem
Approach
Steps
Complexity
Input: An array `gifts` representing the number of gifts in different piles. Also, an integer `k` representing the number of seconds.
Example: gifts = [100, 64, 25, 16], k = 3
Constraints:
• 1 <= gifts.length <= 1000
• 1 <= gifts[i] <= 10^9
• 1 <= k <= 1000
Output: The total number of gifts left after performing the operation for `k` seconds.
Example: 36
Constraints:
Goal: After performing the operation `k` times, return the total number of gifts remaining in all piles.
Steps:
• 1. Create a max heap to efficiently get the pile with the most gifts.
• 2. For `k` seconds, pick the pile with the most gifts, compute the floor of the square root of the number of gifts, and replace the number of gifts in that pile with the result.
• 3. After `k` operations, sum the remaining gifts in all piles.
Goal: The solution must handle arrays with up to 1000 piles, and values up to 10^9 in a time-efficient manner.
Steps:
• The number of piles will not exceed 1000.
• The number of gifts in any pile will not exceed 10^9.
• The number of seconds will not exceed 1000.
Assumptions:
• The array `gifts` is initially non-empty.
• The number of seconds `k` is always valid.
• Input: gifts = [100, 64, 25, 16], k = 3
• Explanation: After 3 seconds of choosing the pile with the most gifts, the remaining gifts will be 36.
• Input: gifts = [1, 1, 1, 1], k = 3
• Explanation: After 3 seconds, no pile can have fewer than 1 gift, so the total number of remaining gifts is 4.
Approach: The key is to use a max heap to always select the pile with the most gifts efficiently. After selecting the pile, compute the floor of the square root of the pile and update it.
Observations:
• To solve this efficiently, we need a data structure that can give us the largest pile in constant time.
• A max heap (or priority queue) will help in efficiently getting and updating the pile with the maximum number of gifts.
Steps:
• 1. Initialize a max heap with the gifts array.
• 2. For each of the `k` seconds, pop the largest pile, compute its square root, update the pile, and push it back into the heap.
• 3. After `k` seconds, sum the elements of the heap and return the total.
Empty Inputs:
• The array `gifts` is guaranteed to be non-empty.
Large Inputs:
• Handle large values of gifts (up to 10^9) efficiently.
Special Values:
• If all piles have 1 gift, no pile can be reduced further, and the total will remain constant.
Constraints:
• Ensure the algorithm handles arrays with up to 1000 elements and operates within the time limit for large values of `gifts[i]`.
long long pickGifts(vector<int>& g, int k) {
make_heap(g.begin(), g.end());
while(k--) {
pop_heap(begin(g), end(g));
g.back() = sqrt(g.back());
push_heap(begin(g), end(g));
}
return accumulate(begin(g), end(g), 0LL);
}
1 : Function Definition
long long pickGifts(vector<int>& g, int k) {
The function 'pickGifts' is defined, taking a vector of integers 'g' and an integer 'k' as input. It returns a long long integer representing the total sum after 'k' operations.
2 : Heap Initialization
make_heap(g.begin(), g.end());
The function initializes the vector 'g' as a max heap using the 'make_heap' function. This allows efficient access to the largest element in 'g'.
3 : Loop Start
while(k--) {
A while loop begins, which will run 'k' times, where each iteration modifies the largest element in the heap.
4 : Heap Pop
pop_heap(begin(g), end(g));
The largest element in the heap is removed using 'pop_heap'. This moves the largest element to the end of the vector 'g'.
5 : Element Modification
g.back() = sqrt(g.back());
The largest element (now at the back of the vector) is replaced with its square root. This simulates 'modifying' the gift.
6 : Heap Push
push_heap(begin(g), end(g));
The modified element is pushed back into the heap to restore the heap property.
7 : Return Result
return accumulate(begin(g), end(g), 0LL);
After 'k' iterations, the function returns the sum of all the elements in the heap using the 'accumulate' function, starting with an initial sum of 0.
Best Case: O(k * log n), where n is the number of piles and k is the number of seconds.
Average Case: O(k * log n), since in each of the `k` operations, we perform a log n operation for heap insertion/removal.
Worst Case: O(k * log n), which is the time complexity for handling all `k` seconds with `n` piles.
Description:
Best Case: O(n), as the space complexity depends on the number of piles.
Worst Case: O(n), where n is the number of piles, as we need space to store the heap.
Description: The space complexity is dominated by the space required to store the heap of piles.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus