Leetcode 2555: Maximize Win From Two Segments
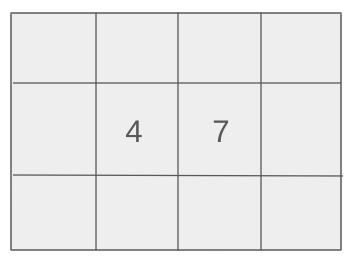
You are given a sorted array
prizePositions
containing positions of prizes along a line and an integer k
. Your task is to select two segments of length k
such that you can maximize the number of prizes collected. The two segments may overlap, and you can collect all prizes within either of the segments.Problem
Approach
Steps
Complexity
Input: You are given an integer array `prizePositions` sorted in non-decreasing order, and an integer `k` representing the length of the two segments.
Example: prizePositions = [1, 1, 2, 2, 3, 3, 5], k = 2
Constraints:
• 1 <= prizePositions.length <= 10^5
• 1 <= prizePositions[i] <= 10^9
• 0 <= k <= 10^9
Output: Return the maximum number of prizes you can collect by selecting two segments of length `k` optimally.
Example: 7
Constraints:
Goal: Maximize the number of prizes collected by optimally selecting two segments.
Steps:
• 1. Iterate through the array `prizePositions` while maintaining a dynamic programming array `dp` to track the maximum number of prizes collectable with one segment.
• 2. For each position, calculate the maximum number of prizes you can win by selecting a segment ending at that position.
• 3. Update the result by considering the combination of the second segment after the first segment, ensuring the total number of prizes is maximized.
Goal: Ensure the solution handles both small and large inputs, as well as edge cases.
Steps:
• 1 <= prizePositions.length <= 10^5
• 1 <= prizePositions[i] <= 10^9
• 0 <= k <= 10^9
Assumptions:
• The array `prizePositions` is sorted in non-decreasing order.
• Input: prizePositions = [1, 1, 2, 2, 3, 3, 5], k = 2
• Explanation: By selecting two segments: [1, 3] and [3, 5], all 7 prizes are covered.
• Input: prizePositions = [1, 2, 3, 4], k = 0
• Explanation: With `k = 0`, the segments can only cover a single prize, so the maximum is 2 prizes with segments [3, 3] and [4, 4].
Approach: We aim to maximize the number of prizes collected by optimally choosing two segments. We use dynamic programming to track the best selection of prizes for each segment.
Observations:
• We need to select the maximum possible number of prizes within two non-overlapping segments.
• We can use dynamic programming to track the maximum number of prizes we can win by selecting one segment and combining it with the next segment.
Steps:
• 1. Use a dynamic programming array `dp` where `dp[i]` represents the maximum number of prizes that can be collected by selecting a segment ending at position `i`.
• 2. Iterate over the `prizePositions` array and update `dp` by considering the number of prizes that can be selected for each segment.
• 3. Use a sliding window or two-pointer technique to efficiently combine two segments and maximize the total number of prizes.
Empty Inputs:
• The array `prizePositions` will always contain at least one element, according to the constraints.
Large Inputs:
• Ensure that the solution works efficiently with large inputs where `prizePositions.length` can go up to 100,000.
Special Values:
• Handle cases where `k = 0`, which means the segments can only cover a single prize.
Constraints:
• The solution should handle large numbers up to the maximum constraint of `10^9`.
int maximizeWin(vector<int>& pos, int k) {
int n = pos.size(), res = 0;
vector<int> dp(n + 1, 0);
int j = 0;
for(int i = 0; i < n; i++) {
while(pos[j] < pos[i] - k) j++;
dp[i + 1] = max(dp[i], i - j + 1);
res = max(res, i - j + 1 + dp[j]);
}
return res;
}
1 : Function Definition
int maximizeWin(vector<int>& pos, int k) {
This is the function definition for 'maximizeWin'. It takes a vector 'pos' representing the positions and an integer 'k' representing the constraint.
2 : Variable Initialization
int n = pos.size(), res = 0;
Here, the integer 'n' is initialized to the size of the 'pos' vector, and 'res' is initialized to 0 to store the result (maximum number of wins).
3 : Dynamic Programming Array Initialization
vector<int> dp(n + 1, 0);
A dynamic programming (DP) array 'dp' is initialized with size 'n + 1' and all elements set to 0. This array will store the maximum number of wins up to each position.
4 : Variable Initialization
int j = 0;
The variable 'j' is initialized to 0 and will be used as the sliding window pointer to track the range of valid positions for counting wins.
5 : Loop Through Positions
for(int i = 0; i < n; i++) {
This for loop iterates over the positions in the 'pos' vector, processing each position one by one.
6 : Sliding Window Movement
while(pos[j] < pos[i] - k) j++;
The while loop shifts the window pointer 'j' to the right until the condition 'pos[j] < pos[i] - k' is no longer true, maintaining the valid range of positions.
7 : Update DP Array
dp[i + 1] = max(dp[i], i - j + 1);
This line updates the DP array at index 'i + 1', calculating the maximum wins between the current value 'dp[i]' and the number of wins in the valid window (i - j + 1).
8 : Update Result
res = max(res, i - j + 1 + dp[j]);
This line updates the result 'res' to the maximum value between the current 'res' and the sum of the number of wins in the current window (i - j + 1) plus the previously computed wins up to position 'j'.
9 : Return Result
return res;
The function returns the value of 'res', which is the maximum number of wins a player can achieve given the positions and constraint 'k'.
Best Case: O(n), where n is the length of the `prizePositions` array.
Average Case: O(n), since we iterate through the array once and use dynamic programming to compute the result.
Worst Case: O(n), as we need to iterate over the entire array to calculate the best result.
Description:
Best Case: O(1), if no additional space is used.
Worst Case: O(n), since we need to store the dynamic programming array `dp` of size n.
Description: Space complexity is dominated by the `dp` array used for dynamic programming.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus