Leetcode 2549: Count Distinct Numbers on Board
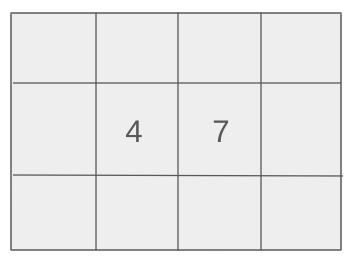
You are given a positive integer
n
, initially placed on a board. For each number x
on the board, find all integers i
such that 1 <= i <= n
and x % i == 1
. Add these numbers to the board. Your goal is to determine how many distinct integers will be present on the board after a billion days.Problem
Approach
Steps
Complexity
Input: You are given a single integer `n` which represents the number initially placed on the board.
Example: n = 6
Constraints:
• 1 <= n <= 100
Output: Return the number of distinct integers on the board after performing the described procedure for one billion days.
Example: 4
Constraints:
Goal: Find the distinct numbers on the board after the given procedure is performed for one billion days.
Steps:
• 1. Start with `n` as the initial number on the board.
• 2. For each number `x` on the board, find all `i` such that `x % i == 1`.
• 3. Add the values of `i` to the board if they are not already present.
• 4. Repeat the above steps for one billion days.
Goal: The solution should efficiently handle the constraints given the number of iterations.
Steps:
• 1 <= n <= 100
Assumptions:
• The procedure will run for a large number of iterations (one billion days).
• The board will keep adding distinct numbers that satisfy the modulo condition.
• Input: n = 6
• Explanation: Initially, the number 6 is placed on the board. Over time, the numbers 2, 4, and 3 will be added to the board, resulting in the distinct numbers: 2, 3, 4, and 6.
• Input: n = 7
• Explanation: For `n = 7`, the numbers added to the board after the operations are 2, 3, 6, and 7, making the distinct numbers 2, 3, 6, and 7.
Approach: The key observation is that the process of adding numbers to the board can be efficiently modeled using the modulo operation to identify which numbers will appear on the board after many iterations.
Observations:
• The operation depends on finding numbers where `x % i == 1` for all current numbers `x` on the board.
• The numbers that appear on the board will be a result of modulo operations from previous numbers.
• If we start with `n`, we need to find all integers `i` such that `x % i == 1`. After a few iterations, a pattern emerges that allows us to count distinct values efficiently.
Steps:
• 1. Start with the number `n` on the board.
• 2. For each number `x` on the board, identify all numbers `i` such that `x % i == 1`.
• 3. Add those numbers to the board if they are not already present.
• 4. Repeat the process for one billion days. Since the operation depends on modulo properties, we can predict the distinct numbers that will remain.
Empty Inputs:
• There are no cases where the input is empty, as the problem guarantees that `n >= 1`.
Large Inputs:
• For very large inputs of `n`, the solution must be optimized to avoid iterating over all days explicitly.
Special Values:
• Consider special cases where `n` is very small (e.g., `n = 1`) or where all numbers on the board quickly stabilize.
Constraints:
• Ensure the solution handles the upper constraint of `n = 100` efficiently.
int distinctIntegers(int n) {
if(n == 1 || n == 2) return 1;
return n - 1;
}
1 : Function Definition
int distinctIntegers(int n) {
The function 'distinctIntegers' is defined with one parameter, 'n'. It calculates the number of distinct integers in a given set based on the value of 'n'.
2 : Condition Check
if(n == 1 || n == 2) return 1;
If n is equal to 1 or 2, the function immediately returns 1 as the number of distinct integers in this case is always 1.
3 : Return Statement
return n - 1;
If n is greater than 2, the function returns n-1, which represents the number of distinct integers in the set from 1 to n.
Best Case: O(n), where n is the number of distinct numbers on the board.
Average Case: O(n), as the number of new numbers added can be at most proportional to `n`.
Worst Case: O(n), as the number of distinct values that can appear on the board is bounded by `n`.
Description: The time complexity is linear in terms of the size of `n`, as we need to process each number once.
Best Case: O(1), in cases where the number of distinct integers does not increase.
Worst Case: O(n), as we store the distinct numbers on the board.
Description: The space complexity is O(n), as we only need to store the distinct numbers added to the board.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus