Leetcode 2527: Find Xor-Beauty of Array
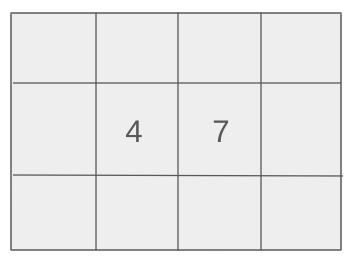
You are given an integer array nums. The xor-beauty of the array is the XOR of the effective values of all possible triplets of indices (i, j, k) where 0 <= i, j, k < n. The effective value for each triplet is calculated as ((nums[i] | nums[j]) & nums[k]).
Problem
Approach
Steps
Complexity
Input: The input consists of a list of integers nums.
Example: [3, 7]
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
Output: Return the xor-beauty of the array, which is the XOR of the effective values for all triplets of indices (i, j, k).
Example: 4
Constraints:
• The output will be a single integer representing the xor-beauty of the array.
Goal: To calculate the xor-beauty by XORing all the effective values of triplets of indices in the array.
Steps:
• Iterate through all possible triplets (i, j, k).
• For each triplet, compute the effective value ((nums[i] | nums[j]) & nums[k]).
• XOR all the computed effective values to obtain the final result.
Goal: The array can have up to 100,000 elements, and each element can be as large as 10^9.
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
Assumptions:
• The length of the array and the size of the numbers will fit within the given constraints.
• The solution should handle large inputs efficiently.
• Input: [3, 7]
• Explanation: The xor-beauty is calculated by XORing the effective values of all the triplets of indices in the array, resulting in the final value of 4.
• Input: [15, 22, 11, 8, 40]
• Explanation: The xor-beauty of the array is calculated by XORing the effective values of all possible triplets, and the result is 22.
Approach: The solution involves iterating over all possible triplets and calculating the effective values by applying bitwise OR and AND operations. The final result is obtained by XORing all the effective values.
Observations:
• This problem involves iterating over all triplets and performing bitwise operations, which can be optimized by reducing the number of calculations.
• Given the constraints, a direct approach that computes the XOR for each possible triplet will be inefficient. We need to explore optimizations or mathematical insights for faster computation.
Steps:
• Loop through all possible triplets (i, j, k).
• For each triplet, calculate the effective value using bitwise OR and AND.
• Accumulate the XOR of the effective values.
• Return the accumulated result.
Empty Inputs:
• There are no empty inputs since the length of nums is always at least 1.
Large Inputs:
• Ensure that the solution can handle large arrays efficiently, especially when nums.length is close to 10^5.
Special Values:
• Handle cases where all numbers in nums are the same.
Constraints:
• Ensure that the time complexity is optimized for large inputs.
int xorBeauty(vector<int>& nums) {
int tmp = 0;
for(int it: nums)
tmp ^= it;
return tmp;
}
1 : Function Definition
int xorBeauty(vector<int>& nums) {
This is the definition of the 'xorBeauty' function which takes a reference to a vector of integers 'nums' and returns an integer result.
2 : Variable Initialization
int tmp = 0;
Here, a temporary variable 'tmp' is initialized to 0. This variable will store the result of the cumulative XOR operation.
3 : Loop
for(int it: nums)
This is a range-based for loop that iterates through each element 'it' in the 'nums' vector.
4 : XOR Operation
tmp ^= it;
In each iteration of the loop, the XOR operation is performed between the current value of 'tmp' and the element 'it'. This accumulates the XOR of all elements in the vector.
5 : Return Statement
return tmp;
After completing the loop, the function returns the final value of 'tmp', which is the cumulative XOR of all elements in the 'nums' vector.
Best Case: O(n^3)
Average Case: O(n^3)
Worst Case: O(n^3)
Description: The time complexity is O(n^3) because we need to consider every triplet in the array for calculating the effective values.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we only need a constant amount of extra space for the XOR calculation.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus