Leetcode 2517: Maximum Tastiness of Candy Basket
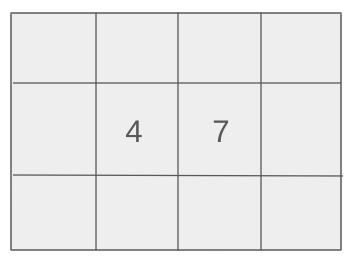
You are given an array of positive integers
price
where price[i]
denotes the price of the i
-th candy and a positive integer k
. The store sells baskets containing k
distinct candies. The tastiness of a candy basket is defined as the smallest absolute difference between the prices of any two candies in the basket. Your task is to return the maximum tastiness of a candy basket that can be formed by selecting k
distinct candies.Problem
Approach
Steps
Complexity
Input: You are given an array of positive integers `price` where each element represents the price of a candy, and a positive integer `k` which denotes the number of candies to be selected.
Example: price = [15, 3, 10, 8, 25, 4], k = 3
Constraints:
• 2 <= k <= price.length <= 10^5
• 1 <= price[i] <= 10^9
Output: Return the maximum tastiness of the candy basket that can be formed, or -1 if it is not possible.
Example: Output: 7
Constraints:
• The output should be the maximum tastiness of a candy basket.
Goal: We need to find the maximum tastiness for a basket of `k` distinct candies from the given prices.
Steps:
• Sort the array of prices.
• Use binary search to determine the maximum tastiness.
• Check if a basket with `k` candies can have the desired tastiness using a helper function.
• Return the maximum tastiness found.
Goal: The input values for the prices array and the integer `k` must satisfy the given constraints.
Steps:
• The array `price` has at least `k` elements.
• The value of each element in `price` is positive and does not exceed 10^9.
• The value of `k` is between 2 and the length of `price`.
Assumptions:
• All elements in `price` are positive integers.
• The number of candies `k` is valid and at least 2.
• Input: price = [15, 3, 10, 8, 25, 4], k = 3
• Explanation: After sorting the prices, the array becomes [3, 4, 8, 10, 15, 25]. The maximum tastiness is achieved by selecting the basket with prices [15, 10, 25], where the smallest absolute difference is 7.
• Input: price = [5, 6, 7, 5, 7], k = 2
• Explanation: The array of prices is [5, 5, 6, 7, 7]. The maximum tastiness of a basket formed by selecting two candies is 1, which occurs by selecting prices [5, 6].
Approach: We will use binary search on the tastiness value to find the maximum tastiness for a basket of `k` distinct candies.
Observations:
• The problem requires finding the maximum tastiness of a basket of `k` distinct candies.
• Binary search can be used to efficiently find the maximum tastiness.
• We can use binary search to minimize the tastiness and check if it is possible to create a valid basket.
Steps:
• Sort the `price` array.
• Set the initial binary search range between 0 and the difference between the largest and smallest price.
• Use a helper function `can()` to check if it's possible to form a basket with the desired tastiness.
• Adjust the binary search range based on the result of `can()`.
Empty Inputs:
• If the array `price` is empty or contains fewer than `k` elements, return -1.
Large Inputs:
• Ensure the solution is efficient enough to handle large inputs, up to 10^5 elements.
Special Values:
• If all elements in the `price` array are the same, the tastiness will always be 0.
Constraints:
• Ensure the solution handles cases where `k` is equal to the length of the `price` array.
bool can(vector<int> nums, int mid, int k) {
int cnt = 1, n = nums.size();
int i = 1;
int prv = nums[0];
while(i < n) {
if(nums[i] - prv >= mid) {
cnt++;
prv = nums[i];
}
if(cnt >= k) return true;
i++;
}
return false;
}
int maximumTastiness(vector<int>& nums, int k) {
sort(nums.begin(), nums.end());
int n = nums.size();
int l = 0, r = nums[n - 1] - nums[0];
int ans = r;
while(l <= r) {
int mid = l + (r - l + 1) / 2;
// cout << mid << " " << can(nums, mid, k) << "\n";
if(can(nums, mid, k)) {
ans = mid;
l = mid + 1;
} else r = mid - 1;
}
return ans;
}
1 : Function Definition
bool can(vector<int> nums, int mid, int k) {
Define the `can` function that checks if it's possible to select `k` elements from the list `nums` where the minimum difference between consecutive selected elements is at least `mid`.
2 : Variable Initialization
int cnt = 1, n = nums.size();
Initialize `cnt` to 1 (since we select the first element), and `n` to the size of the input list `nums`.
3 : Variable Initialization
int i = 1;
Initialize the variable `i` to 1 to start the iteration from the second element in the list.
4 : Variable Initialization
int prv = nums[0];
Store the first element of `nums` in the variable `prv` to track the previously selected element.
5 : Loop
while(i < n) {
Start a `while` loop that iterates over the list `nums` starting from the second element.
6 : Condition Check
if(nums[i] - prv >= mid) {
Check if the difference between the current element `nums[i]` and the previously selected element `prv` is greater than or equal to `mid`.
7 : Count Update
cnt++;
Increment `cnt` to reflect the selection of another element that meets the condition.
8 : Update Previous Element
prv = nums[i];
Update the `prv` variable to store the current selected element `nums[i]`.
9 : Early Exit
if(cnt >= k) return true;
If `cnt` reaches `k`, return `true` as we have successfully selected `k` elements with the desired property.
10 : Increment Iterator
i++;
Increment `i` to move to the next element in the list.
11 : Return Statement
return false;
Return `false` if it's not possible to select `k` elements with the desired property.
12 : Function Definition
int maximumTastiness(vector<int>& nums, int k) {
Define the `maximumTastiness` function that aims to find the maximum possible value of `mid` for which `can(nums, mid, k)` is true.
13 : Sort Array
sort(nums.begin(), nums.end());
Sort the input list `nums` to facilitate the selection of elements with a minimum difference.
14 : Variable Initialization
int n = nums.size();
Store the size of the sorted `nums` list in `n`.
15 : Binary Search Setup
int l = 0, r = nums[n - 1] - nums[0];
Initialize the binary search bounds: `l` to 0 and `r` to the difference between the largest and smallest elements in `nums`.
16 : Variable Initialization
int ans = r;
Initialize `ans` to `r`, which represents the current best answer for the maximum tastiness.
17 : Binary Search Loop
while(l <= r) {
Start the binary search loop with the condition `l <= r`.
18 : Midpoint Calculation
int mid = l + (r - l + 1) / 2;
Calculate the midpoint `mid` to check the possible value of tastiness.
19 : Condition Check
if(can(nums, mid, k)) {
Check if the current value of `mid` satisfies the condition by calling the `can` function.
20 : Update Answer
ans = mid;
Update the best answer `ans` to the current value of `mid` if the condition is satisfied.
21 : Adjust Search Bounds
l = mid + 1;
Adjust the lower bound `l` to search for larger values of `mid`.
22 : Adjust Search Bounds
} else r = mid - 1;
Adjust the upper bound `r` to search for smaller values of `mid` if the condition is not satisfied.
23 : Return Answer
return ans;
Return the final answer `ans`, which is the maximum possible tastiness.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: Sorting the array takes O(n log n) time, and the binary search is O(log n).
Best Case: O(n)
Worst Case: O(n)
Description: We use O(n) space for sorting the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus