Leetcode 2507: Smallest Value After Replacing With Sum of Prime Factors
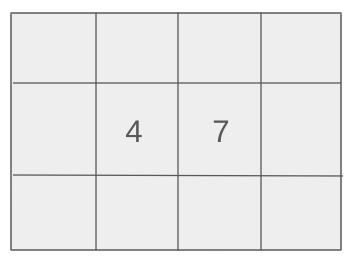
You are given a positive integer n. The task is to repeatedly replace n with the sum of its prime factors, and continue this process until n reaches a value that cannot be reduced further. The process stops when the sum of the prime factors is equal to the current value of n. Return the smallest value n will eventually become.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer n, where 2 <= n <= 10^5.
Example: n = 18
Constraints:
• 2 <= n <= 10^5
Output: The output should be the smallest value that n will eventually become.
Example: 5
Constraints:
Goal: The goal is to find the smallest value that n will eventually become after repeatedly replacing it with the sum of its prime factors.
Steps:
• Find the prime factors of the number n, including repeated factors.
• Replace n with the sum of its prime factors.
• Repeat the process until n cannot be reduced further, i.e., when the sum of the prime factors equals the current value of n.
Goal: The integer n will always be a positive number greater than or equal to 2.
Steps:
• 2 <= n <= 10^5
Assumptions:
• n is always a positive integer.
• n is not a prime number in all cases (except when the prime factors sum process stabilizes).
• Input: n = 18
• Explanation: The process goes as follows: 18 -> 8 -> 6 -> 5. Therefore, 5 is the smallest value n will eventually reach.
Approach: The approach involves finding the prime factors of n and repeatedly replacing n with the sum of its prime factors until a stable value is reached.
Observations:
• Prime factorization can be done using a trial division method.
• If n is divisible by a prime, we repeatedly divide n by that prime, adding it to the sum.
• This process will eventually stabilize because each step reduces n, and the prime factor sum either stays the same or reduces to a smaller number.
Steps:
• Initialize n as the input number.
• Iteratively calculate the sum of prime factors of n until n reaches a stable value.
• For each iteration, find the prime factors, add them, and check if the new n is equal to the sum of the prime factors. If so, stop the process.
Empty Inputs:
• There will always be an input, since n >= 2.
Large Inputs:
• For large values of n, ensure the factorization is efficient to handle up to n = 10^5.
Special Values:
• If n is prime, the process will immediately stop since the prime factor sum will equal n.
Constraints:
• Ensure that the solution handles edge cases like n being a small prime number.
int getFactSum(int n) {
int div = 2, sum = 0;
while(n > 1) {
if(n % div == 0) {
sum += div;
n /= div;
} else div++;
}
return sum;
}
int smallestValue(int n) {
while(1) {
int sum = getFactSum(n);
if(sum == n) break;
n = sum;
}
return n;
}
1 : Function Definition
int getFactSum(int n) {
Defines the `getFactSum` function that calculates the sum of divisors of the given integer `n`.
2 : Variable Initialization
int div = 2, sum = 0;
Initializes two variables: `div`, starting at 2 (the smallest prime divisor), and `sum`, which stores the sum of divisors of `n`.
3 : Loop Structure
while(n > 1) {
Starts a while loop that runs as long as `n` is greater than 1. This loop will find divisors of `n`.
4 : Condition Check
if(n % div == 0) {
Checks if `n` is divisible by `div`. If true, the loop continues to find the divisors.
5 : Sum Update
sum += div;
Adds `div` to the sum if `div` is a divisor of `n`.
6 : Variable Update
n /= div;
Divides `n` by `div` to reduce it for the next iteration, potentially to find other divisors.
7 : Variable Update
} else div++;
If `n` is not divisible by `div`, it increments `div` to check the next potential divisor.
8 : Return Statement
return sum;
Returns the sum of divisors of `n`.
9 : Function Definition
int smallestValue(int n) {
Defines the `smallestValue` function that repeatedly updates `n` with the sum of its divisors until `n` equals its divisor sum.
10 : Infinite Loop
while(1) {
Starts an infinite loop that will keep running until the condition inside the loop is satisfied.
11 : Function Call
int sum = getFactSum(n);
Calls the `getFactSum` function to calculate the sum of divisors of `n`.
12 : Condition Check
if(sum == n) break;
Checks if the sum of divisors of `n` is equal to `n`. If they are equal, it exits the loop.
13 : Variable Update
n = sum;
If the sum of divisors is not equal to `n`, it updates `n` to the sum and continues the loop.
14 : Return Statement
return n;
Returns the value of `n` after it becomes equal to the sum of its divisors.
Best Case: O(log n) for prime numbers, as no further changes are needed.
Average Case: O(sqrt(n)) for factorization of each number.
Worst Case: O(n) for large composite numbers, depending on the prime factorization steps.
Description: Time complexity is dominated by the prime factorization process.
Best Case: O(1), if n is prime and no additional storage is needed.
Worst Case: O(log n), due to the storage needed for prime factorization.
Description: The space complexity is minimal unless we store intermediate factors.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus