Leetcode 2506: Count Pairs Of Similar Strings
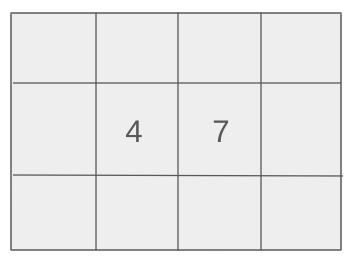
You are given a list of words, where each word consists of lowercase English letters. Two words are considered similar if they contain the exact same set of unique characters, regardless of the order. Your task is to count how many pairs of words (i, j) satisfy the condition where both words have the same set of unique characters. Return the count of such pairs (i, j) where 0 ≤ i < j ≤ len(words) - 1.
Problem
Approach
Steps
Complexity
Input: The input consists of a list of words, where each word is a string of lowercase English letters.
Example: ["aba", "aabb", "abcd", "bac", "aabc"]
Constraints:
• 1 <= words.length <= 100
• 1 <= words[i].length <= 100
• words[i] consist of only lowercase English letters.
Output: The output is the number of pairs (i, j) where the words[i] and words[j] have the same set of unique characters.
Example: 2
Constraints:
Goal: The goal is to count pairs of words that consist of the same set of unique characters.
Steps:
• For each word in the input list, calculate a unique bitmask representing the set of characters in the word.
• Use a map to store the frequency of each unique bitmask.
• For each word's bitmask, find how many previous words have the same bitmask, indicating that the two words are similar.
• Count the number of such pairs and return the total count.
Goal: The number of words and the length of each word are constrained to ensure that the solution runs efficiently.
Steps:
• 1 <= words.length <= 100
• 1 <= words[i].length <= 100
• words[i] consist of only lowercase English letters.
Assumptions:
• The input list will not contain any null or empty words.
• Each word consists only of lowercase English letters.
• Input: ["aba", "aabb", "abcd", "bac", "aabc"]
• Explanation: In this example, the words 'aba' and 'aabb' are similar because they consist of the characters 'a' and 'b', and the words 'bac' and 'aabc' are similar because they consist of 'a', 'b', and 'c'. Therefore, there are 2 such pairs.
Approach: The approach uses bitwise operations to efficiently represent and compare the set of characters in each word.
Observations:
• Each word can be represented by a bitmask where each bit represents a character from 'a' to 'z'.
• If two words have the same bitmask, they contain the same set of characters, regardless of order.
Steps:
• For each word, compute its bitmask by iterating through its characters and setting the corresponding bit.
• Store the frequency of each bitmask in a hashmap.
• For each word's bitmask, count how many times this bitmask has been seen before, which indicates a pair of similar words.
Empty Inputs:
• If the input list is empty, the result should be 0.
Large Inputs:
• If the input list has the maximum number of words (100), ensure that the solution efficiently handles this size.
Special Values:
• Words with only one character should also be handled correctly.
Constraints:
• Ensure that words containing only distinct characters are correctly identified as similar if another word contains the same characters.
int similarPairs(vector<string>& words) {
int ans = 0;
unordered_map<int, int> freq;
for (auto& word : words) {
int mask = 0;
for (auto& c : word) mask |= 1 << (c-'a');
ans += freq[mask]++;
}
return ans;
}
1 : Function Definition
int similarPairs(vector<string>& words) {
Defines the function `similarPairs` which takes a reference to a vector of strings `words` and returns the number of similar pairs.
2 : Variable Initialization
int ans = 0;
Initializes a variable `ans` to store the count of similar pairs. The initial value is 0.
3 : Data Structure Initialization
unordered_map<int, int> freq;
Declares an unordered map `freq` where the key is an integer (representing a unique bitmask) and the value is the frequency of that bitmask.
4 : Loop Structure
for (auto& word : words) {
Begins a loop that iterates over each word in the input vector `words`.
5 : Variable Initialization
int mask = 0;
Initializes a variable `mask` to 0. This will be used to create a bitmask representing the unique characters in the current word.
6 : Loop Structure
for (auto& c : word) mask |= 1 << (c-'a');
Inner loop that iterates over each character `c` in the word. It updates the `mask` by setting the corresponding bit for the character (using bitwise operations).
7 : Map Manipulation
ans += freq[mask]++;
Increments `ans` by the frequency of the current `mask` in the `freq` map, then updates the frequency of `mask` in the map.
8 : Loop Structure
}
Ends the loop that processes each word in the `words` vector.
9 : Return Statement
return ans;
Returns the total count of similar pairs stored in `ans`.
Best Case: O(n * m), where n is the number of words and m is the average length of the words.
Average Case: O(n * m), since we have to process each word individually.
Worst Case: O(n * m), where n is the number of words and m is the maximum length of the words.
Description: The complexity comes from processing each word and storing its bitmask, as well as looking up bitmask frequencies in the hashmap.
Best Case: O(n), where n is the number of words.
Worst Case: O(n), since we need to store the bitmask frequencies for each word.
Description: The space complexity is proportional to the number of words and the number of distinct bitmasks.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus