Leetcode 2500: Delete Greatest Value in Each Row
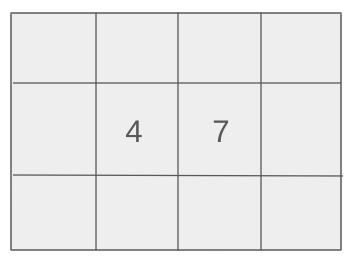
You are given a matrix grid with positive integers. In each operation, remove the greatest value from each row, and if multiple elements have the same greatest value, remove any one of them. After removing the greatest value from all rows, add the maximum of these values to the answer. The number of columns decreases by one after each operation. Perform these operations until the grid is empty, and return the sum of the maximum values from all operations.
Problem
Approach
Steps
Complexity
Input: You are given a 2D grid where each row contains positive integers. The grid has m rows and n columns, and the values in the grid are sorted within each row in ascending order.
Example: Input: grid = [[1, 3, 4], [2, 5, 6]]
Constraints:
• 1 <= m, n <= 50
• 1 <= grid[i][j] <= 100
Output: Return the sum of the maximum values removed from the grid in each operation.
Example: Output: 13
Constraints:
• The sum is calculated by adding the maximum value removed in each operation.
Goal: The goal is to compute the sum of the maximum values from the elements removed from each row during each operation, by reducing the grid by one column at a time.
Steps:
• 1. For each row in the grid, sort the values to easily access the greatest value.
• 2. In each operation, remove the greatest element from each row and find the maximum among them.
• 3. Keep track of the sum of these maximum values until the grid becomes empty.
Goal: Ensure the grid is valid and its size falls within the provided constraints.
Steps:
• The grid has at least one row and one column.
• The matrix is not empty and contains only positive integers.
Assumptions:
• All rows are not empty and contain at least one element.
• Input: Input: grid = [[1, 3, 4], [2, 5, 6]]
• Explanation: In the first operation, we remove 4 from the first row and 6 from the second row, adding 6 to the result. In the second operation, we remove 3 from the first row and 5 from the second row, adding 5 to the result. In the final operation, we remove 1 and 2, adding 2 to the result. The total sum is 6 + 5 + 2 = 13.
• Input: Input: grid = [[10]]
• Explanation: In this case, there is only one element, 10, and it is removed in the first operation. The total sum is 10.
Approach: The solution involves sorting each row to easily access the maximum element and then performing the operation of removing the largest elements until the grid is empty. The result is the sum of the maximum values removed in each operation.
Observations:
• Sorting each row will allow easy access to the maximum element.
• By sorting the rows, we can directly pick the maximum element and track the maximum values in each operation.
Steps:
• 1. Sort each row of the grid in ascending order.
• 2. For each column (from left to right), find the maximum element in the current column across all rows.
• 3. Add the maximum value of the current column to the result.
• 4. Repeat the above steps until the grid becomes empty.
Empty Inputs:
• There will always be at least one row and one column, so this case is not applicable.
Large Inputs:
• Ensure the solution can handle grids of the largest size, with m and n up to 50.
Special Values:
• Handle cases where the grid has only one row or one column.
Constraints:
• The solution should efficiently handle cases with maximum values for m and n.
int deleteGreatestValue(vector<vector<int>>& g) {
int res = 0, si = g.size(), sj = g[0].size();
for (auto &r : g)
sort(begin(r), end(r));
for (int j = 0; j < sj; ++j) {
int max_row = 0;
for (int i = 0; i < si; ++i)
max_row = max(max_row, g[i][j]);
res += max_row;
}
return res;
}
1 : Function Definition
int deleteGreatestValue(vector<vector<int>>& g) {
Defines the function `deleteGreatestValue` that takes a 2D vector `g` (a matrix) and returns an integer representing the sum of the greatest values in each column.
2 : Variable Initialization
int res = 0, si = g.size(), sj = g[0].size();
Initializes the variable `res` to store the result, and calculates the number of rows (`si`) and columns (`sj`) in the 2D vector `g`.
3 : Looping
for (auto &r : g)
Loops through each row of the 2D vector `g`.
4 : Sorting
sort(begin(r), end(r));
Sorts each row of the 2D vector `g` in ascending order.
5 : Looping
for (int j = 0; j < sj; ++j) {
Starts a loop to iterate through each column (`j`) of the sorted 2D vector.
6 : Variable Initialization
int max_row = 0;
Initializes a variable `max_row` to store the maximum value in the current column during the iteration.
7 : Looping
for (int i = 0; i < si; ++i)
Starts a loop to iterate through each row (`i`) in the current column (`j`).
8 : Mathematical Operations
max_row = max(max_row, g[i][j]);
Calculates the maximum value between the current `max_row` and the current element `g[i][j]` in the column.
9 : Result Calculation
res += max_row;
Adds the maximum value found in the current column (`max_row`) to the result `res`.
10 : Return Statement
return res;
Returns the final result `res`, which is the sum of the greatest values from each column.
Best Case: O(m * n log n)
Average Case: O(m * n log n)
Worst Case: O(m * n log n)
Description: The time complexity is dominated by the sorting step for each row, which takes O(n log n) per row, resulting in a total complexity of O(m * n log n).
Best Case: O(m * n)
Worst Case: O(m * n)
Description: The space complexity is O(m * n) since we need to store the grid, and the sorting operation requires additional space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus