Leetcode 2497: Maximum Star Sum of a Graph
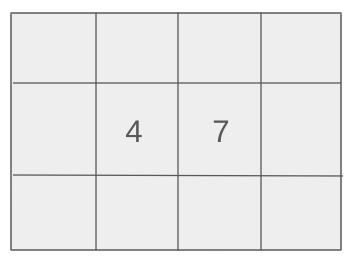
Given a graph with n nodes, each node having a value, and a list of edges connecting the nodes, your task is to determine the maximum star sum that can be obtained. A star graph is a subgraph where all edges are connected to a central node. The star sum is the sum of the values of the central node and the nodes connected by edges to it. You are allowed to include at most k edges in the star graph.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer array vals representing the values of the nodes, a 2D integer array edges representing the undirected edges, and an integer k representing the maximum number of edges that can be included in the star graph.
Example: Input: vals = [1,2,3,4,10,-10,-20], edges = [[0,1],[1,2],[1,3],[3,4],[3,5],[3,6]], k = 2
Constraints:
• 1 <= n <= 10^5
• -10^4 <= vals[i] <= 10^4
• 0 <= edges.length <= min(n * (n - 1) / 2, 10^5)
• edges[i].length == 2
• 0 <= ai, bi <= n - 1
• ai != bi
• 0 <= k <= n - 1
Output: Return the maximum star sum that can be obtained from any star graph in the input graph, with at most k edges.
Example: Output: 16
Constraints:
• The sum should include the value of the central node and the values of the nodes connected by at most k edges.
Goal: The goal is to calculate the maximum possible sum of a star graph, centered at any node, using at most k edges.
Steps:
• 1. Initialize a priority queue for each node to store the values of its neighbors.
• 2. Iterate over the nodes and calculate the sum of the node's value and the maximum values from the neighbors' values, considering at most k neighbors.
• 3. For each node, compute the net sum and update the maximum star sum encountered.
• 4. Return the maximum star sum after processing all nodes.
Goal: The input graph should be valid, adhering to the constraints provided, ensuring the graph is undirected and the number of nodes and edges is manageable.
Steps:
• Ensure that the number of nodes does not exceed 100,000 and that the graph is not too sparse for the problem constraints.
Assumptions:
• The input graph is connected and the values of nodes and edges are within the specified range.
• Input: Input: vals = [1,2,3,4,10,-10,-20], edges = [[0,1],[1,2],[1,3],[3,4],[3,5],[3,6]], k = 2
• Explanation: In this case, the star graph with the maximum sum is centered at node 3, with neighbors 1 and 4. The sum is 4 (value of node 3) + 2 (value of node 1) + 10 (value of node 4) = 16.
• Input: Input: vals = [-5], edges = [], k = 0
• Explanation: Here, the only possible star graph is the node itself (node 0). Since there are no edges and k = 0, the star sum is just the value of node 0, which is -5.
Approach: To solve this problem efficiently, we need to use a greedy approach where we consider each node as the center of a potential star graph and evaluate the maximum possible sum by selecting at most k neighboring nodes with the highest values.
Observations:
• We need to prioritize nodes with higher values in order to maximize the star sum.
• A priority queue can help us efficiently select the highest values from a node's neighbors.
Steps:
• 1. For each edge in the graph, store the neighbor values in a priority queue for each node.
• 2. For each node, calculate its star sum by adding its value and the values of its top k neighbors from the priority queue.
• 3. Track the maximum sum encountered during this process.
• 4. Return the maximum star sum.
Empty Inputs:
• If there are no edges in the graph, the maximum star sum is just the value of any single node.
Large Inputs:
• For large graphs with up to 100,000 nodes, the algorithm must be efficient in handling edge cases and ensuring the process runs within time limits.
Special Values:
• Handle cases where nodes have negative values effectively, ensuring that the star sum is correctly calculated even when all nodes have negative values.
Constraints:
• Ensure that the algorithm respects the constraint of selecting at most k neighbors for the star graph.
int maxStarSum(vector<int>& vals, vector<vector<int>>& edges, int k) {
int n = vals.size();
vector<priority_queue<int>> grid(n);
for(auto it: edges) {
grid[it[0]].push(vals[it[1]]);
grid[it[1]].push(vals[it[0]]);
}
int sum = INT_MIN;
for(int i = 0; i < n; i++) {
int net = vals[i];
int tmp = k;
sum = max(net, sum);
while(tmp-- && !grid[i].empty()) {
net += grid[i].top();
grid[i].pop();
sum = max(net, sum);
}
}
return sum;
}
1 : Function Definition
int maxStarSum(vector<int>& vals, vector<vector<int>>& edges, int k) {
Defines the function `maxStarSum` which accepts a vector of integers `vals`, a 2D vector `edges`, and an integer `k` representing the maximum number of neighboring node values to add to the sum.
2 : Array Operations
int n = vals.size();
Gets the size of the `vals` vector, which represents the number of nodes in the graph.
3 : Data Structure Initialization
vector<priority_queue<int>> grid(n);
Initializes a vector of priority queues to store the neighboring node values for each node. The priority queue will help to easily fetch the highest values.
4 : Looping
for(auto it: edges) {
Iterates through each edge in the `edges` list, where each edge is a pair of connected nodes.
5 : Priority Queue Operations
grid[it[0]].push(vals[it[1]]);
For each edge, pushes the value of the neighboring node (`it[1]`) into the priority queue of the first node (`it[0]`).
6 : Priority Queue Operations
grid[it[1]].push(vals[it[0]]);
Similarly, for the reverse direction of the edge, pushes the value of the first node (`it[0]`) into the priority queue of the second node (`it[1]`).
7 : Variable Initialization
int sum = INT_MIN;
Initializes `sum` to the smallest possible integer value to track the maximum sum encountered during the calculations.
8 : Looping
for(int i = 0; i < n; i++) {
Starts a loop to process each node in the graph.
9 : Variable Initialization
int net = vals[i];
Initializes `net` with the value of the current node (`vals[i]`). This variable will store the sum for the current node.
10 : Variable Initialization
int tmp = k;
Initializes `tmp` to `k`, which tracks the remaining number of neighboring nodes to consider for the sum.
11 : Mathematical Operations
sum = max(net, sum);
Compares the current node's value (`net`) with the overall `sum` and updates `sum` with the larger value.
12 : Looping
while(tmp-- && !grid[i].empty()) {
Enters a while loop to add the largest neighbor values to `net`, as long as there are remaining neighbors (`tmp--`) and the priority queue for the node is not empty.
13 : Priority Queue Operations
net += grid[i].top();
Adds the largest value from the current node's priority queue to `net`.
14 : Priority Queue Operations
grid[i].pop();
Removes the largest value from the current node's priority queue after it has been added to `net`.
15 : Mathematical Operations
sum = max(net, sum);
Updates the `sum` to the larger value between `net` and the previous `sum`.
16 : Return Statement
return sum;
Returns the final maximum sum after processing all nodes.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is O(n log n) because we process each node and each edge, and the priority queue operations take logarithmic time.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) as we store a priority queue for each node, which can store up to n neighbor values.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus