Leetcode 2496: Maximum Value of a String in an Array
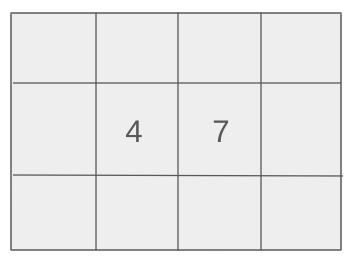
Given an array of alphanumeric strings, you are tasked with determining the maximum value of any string in the array. The value of a string is determined in the following way: If the string consists only of digits, its value is its numeric representation in base 10. If it contains any letters, its value is the length of the string.
Problem
Approach
Steps
Complexity
Input: The input consists of a list of alphanumeric strings, where each string is composed of digits and lowercase English letters.
Example: Input: strs = ["hello1", "abc", "9", "010"]
Constraints:
• 1 <= strs.length <= 100
• 1 <= strs[i].length <= 9
• strs[i] consists of only lowercase English letters and digits.
Output: Return the maximum value of any string in the array, based on the aforementioned rules.
Example: Output: 9
Constraints:
• The maximum value is calculated by evaluating the numeric value for strings with only digits, and the length for strings containing letters.
Goal: The goal is to determine the maximum value by checking if each string consists of digits or contains letters, and then applying the respective rule for value calculation.
Steps:
• 1. Iterate through the list of strings.
• 2. For each string, check if it consists only of digits or contains letters.
• 3. If the string consists of digits, convert it to an integer and compare it with the maximum value found so far.
• 4. If the string contains letters, compare its length with the maximum value.
• 5. Return the maximum value after processing all strings.
Goal: Ensure the list contains valid alphanumeric strings as described, with lengths and formats adhering to the constraints.
Steps:
• No string will contain more than 9 characters.
• All strings consist of lowercase English letters or digits.
Assumptions:
• The input is guaranteed to be valid and conforms to the given constraints.
• Input: Input: strs = ["hello1", "abc", "9", "010"]
• Explanation: In this case, the string "hello1" has a length of 6, "abc" has a length of 3, "9" has a value of 9, and "010" has a value of 10. The maximum value is 10.
• Input: Input: strs = ["1", "2", "01", "3"]
• Explanation: Each string contains only digits, so the values are 1, 2, 1, and 3, respectively. The maximum value is 3.
Approach: To solve this problem, we need to evaluate each string based on whether it consists only of digits or contains letters. We can then calculate its value and track the maximum value.
Observations:
• The challenge lies in correctly differentiating between strings containing digits and those containing letters.
• We can leverage built-in functions like all_of to check for digit-only strings and handle the string length calculation for others.
Steps:
• 1. Initialize a variable to track the maximum value, set to 0 initially.
• 2. For each string in the input array, check if all characters are digits using all_of.
• 3. If the string consists of digits, convert it to an integer and update the maximum value.
• 4. If the string contains letters, calculate its length and update the maximum value.
• 5. Return the maximum value after processing all strings.
Empty Inputs:
• There are no edge cases for empty input since the constraints guarantee at least one string in the array.
Large Inputs:
• Ensure the solution handles up to 100 strings, each with a maximum length of 9 characters.
Special Values:
• Be cautious of strings with leading zeros, which should still be evaluated correctly as numeric values.
Constraints:
• The function should efficiently handle the constraints and provide the correct result.
public:
int maximumValue(vector<string>& strs)
{
int m = 0;
for (string s : strs)
if (all_of(s.begin(), s.end(), ::isdigit))
m = max(m, stoi(s));
else
m = max(m, (int)s.size());
return m;
}
1 : Variable Initialization
public:
Marks the beginning of the function definition in C++ syntax, where the function is defined as a public member function.
2 : Function Definition
int maximumValue(vector<string>& strs)
Defines the function `maximumValue`, which takes a vector of strings as an argument and returns an integer, which will be the maximum value determined by the function logic.
3 : Variable Initialization
int m = 0;
Initializes a variable `m` to 0, which will hold the maximum value found during the iteration over the input strings.
4 : Looping
for (string s : strs)
Starts a loop that iterates through each string `s` in the vector `strs`.
5 : Condition Checking
if (all_of(s.begin(), s.end(), ::isdigit))
Checks if every character in the string `s` is a digit. The `all_of` function verifies this condition.
6 : Mathematical Operations
m = max(m, stoi(s));
If the string `s` consists only of digits, the value is converted to an integer using `stoi()` and compared with `m`. The maximum value is assigned to `m`.
7 : Else Condition
else
Executes the following block of code if the string `s` is not fully numeric.
8 : Mathematical Operations
m = max(m, (int)s.size());
If the string is not numeric, the function compares the length of the string `s` (converted to an integer) with `m` and updates `m` to the larger value.
9 : Return Statement
return m;
Returns the maximum value found, either the largest integer found or the maximum length of any non-numeric string.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the number of strings in the input array, as each string is processed once.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1), as we only use a few variables to track the maximum value.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus