Leetcode 2482: Difference Between Ones and Zeros in Row and Column
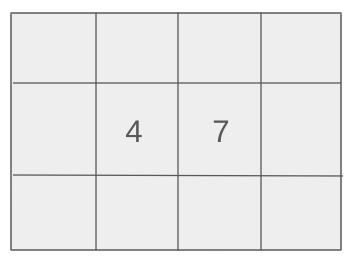
You are given an m x n binary matrix grid. You need to create a difference matrix diff where each element diff[i][j] is calculated by summing the number of ones and subtracting the number of zeros in the respective row and column.
Problem
Approach
Steps
Complexity
Input: The input consists of a binary matrix of size m x n, where each element is either 0 or 1.
Example: grid = [[1,0,1],[0,1,1],[1,1,0]]
Constraints:
• 1 <= m, n <= 10^5
• 1 <= m * n <= 10^5
Output: Return the difference matrix diff, which is of the same size as the input matrix grid.
Example: Output: [[4, 4, 2], [2, 2, 4], [4, 4, 2]]
Constraints:
• The output should be a matrix of integers with the same dimensions as the input.
Goal: The goal is to calculate the difference matrix based on the number of ones and zeros in each row and column.
Steps:
• 1. First, calculate the number of ones and zeros in each row and column.
• 2. Use the formula for diff[i][j] = onesRow_i + onesCol_j - zerosRow_i - zerosCol_j to calculate each value of the matrix diff.
Goal: You are guaranteed that the input grid has at least one row and one column, and the grid will have a maximum size of 10^5 elements.
Steps:
• 1 <= m, n <= 10^5
• 1 <= m * n <= 10^5
Assumptions:
• The grid will always contain binary values (0 or 1).
• Input: Example 1: grid = [[1,0,1],[0,1,1],[1,1,0]]
• Explanation: For each position in the grid, the number of ones and zeros in its respective row and column are used to calculate the difference matrix. The result will depend on how the number of ones and zeros are distributed.
Approach: We can approach this problem by first calculating the number of ones and zeros in each row and column, then using that data to calculate the difference matrix.
Observations:
• We need to efficiently calculate the number of ones and zeros in each row and column, which can be done in O(m*n) time.
• Once we have the row and column sums, calculating the diff matrix is straightforward.
Steps:
• 1. Initialize arrays for row and column sums.
• 2. Loop through the grid to populate these sums.
• 3. Construct the diff matrix by applying the formula to each element.
Empty Inputs:
• There will always be at least one element in the grid, as m and n are guaranteed to be at least 1.
Large Inputs:
• The maximum size of m*n is 10^5, which is feasible for a solution with O(m*n) time complexity.
Special Values:
• If the grid is filled entirely with zeros, the diff matrix will be filled with zeros.
Constraints:
• The problem guarantees that m and n are within the given constraints.
vector<vector<int>> onesMinusZeros(vector<vector<int>>& grid) {
int m = grid.size(), n = grid[0].size();
vector<int> row(m), col(n);
for(int i = 0; i < m; i++)
for(int j = 0; j < n; j++) {
row[i] += grid[i][j];
col[j] += grid[i][j];
}
vector<vector<int>> g(m, vector<int>(n, 0));
for(int i = 0; i < m; i++)
for(int j = 0; j < n; j++) {
g[i][j] = 2 * row[i] + 2 * col[j] - m - n;
}
return g;
}
1 : Function Declaration
vector<vector<int>> onesMinusZeros(vector<vector<int>>& grid) {
This line defines the function `onesMinusZeros`, which takes a 2D vector `grid` as input and returns a 2D vector `g` as the output.
2 : Variable Initialization
int m = grid.size(), n = grid[0].size();
The dimensions of the grid are stored in `m` (number of rows) and `n` (number of columns).
3 : Vector Initialization
vector<int> row(m), col(n);
Two vectors, `row` and `col`, are initialized to store the counts of 1's in each row and column of the grid.
4 : Row and Column Counting
for(int i = 0; i < m; i++)
Loop through each row of the grid.
5 : Nested Loop
for(int j = 0; j < n; j++) {
Nested loop to iterate over each column of the grid.
6 : Row Counting
row[i] += grid[i][j];
Increment the count for the current row `i` based on the value of the cell `grid[i][j]`.
7 : Column Counting
col[j] += grid[i][j];
Increment the count for the current column `j` based on the value of the cell `grid[i][j]`.
8 : Matrix Initialization
vector<vector<int>> g(m, vector<int>(n, 0));
Initialize a new matrix `g` of size `m x n` with all elements set to 0.
9 : Matrix Calculation Loop
for(int i = 0; i < m; i++)
Loop through each row of the result matrix `g`.
10 : Nested Matrix Calculation Loop
for(int j = 0; j < n; j++) {
Nested loop to iterate through each column of the result matrix `g`.
11 : Matrix Calculation
g[i][j] = 2 * row[i] + 2 * col[j] - m - n;
Each element of the result matrix `g[i][j]` is computed using the formula `2 * row[i] + 2 * col[j] - m - n`, where `row[i]` and `col[j]` represent the count of 1's in the respective row and column.
12 : Return Statement
return g;
Return the resulting matrix `g` which has been calculated based on the counts of 1's in the respective rows and columns.
Best Case: O(m*n)
Average Case: O(m*n)
Worst Case: O(m*n)
Description: The time complexity is O(m*n) as we need to traverse the grid to compute row and column sums and then calculate the diff matrix.
Best Case: O(m*n)
Worst Case: O(m*n)
Description: The space complexity is O(m*n) due to the storage of the input grid and the result matrix.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus