Leetcode 2469: Convert the Temperature
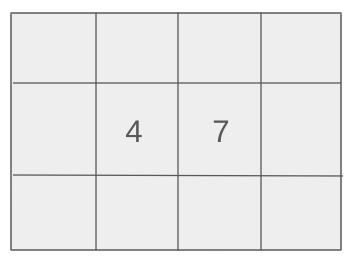
You are given a non-negative floating point number
celsius
(rounded to two decimal places) representing a temperature in Celsius. Convert it to Kelvin and Fahrenheit and return the results in a list. The formulas are: Kelvin = Celsius + 273.15 and Fahrenheit = Celsius * 1.80 + 32.00. Return the results rounded to five decimal places.Problem
Approach
Steps
Complexity
Input: You are given a non-negative floating point number `celsius` representing the temperature in Celsius.
Example: celsius = 25.30
Constraints:
• 0 <= celsius <= 1000
Output: Return a list containing the temperature in Kelvin and Fahrenheit, rounded to five decimal places.
Example: [298.45000, 77.54000]
Constraints:
• Answers within 10^-5 of the actual result will be accepted.
Goal: The goal is to correctly apply the formulas for conversion from Celsius to Kelvin and Fahrenheit.
Steps:
• First, calculate the Kelvin value by adding 273.15 to the Celsius value.
• Next, calculate the Fahrenheit value using the formula Fahrenheit = Celsius * 1.80 + 32.00.
• Return the results as a list, rounding each to five decimal places.
Goal: The value of `celsius` is a non-negative floating point number with two decimal places, ranging from 0 to 1000.
Steps:
• 0 <= celsius <= 1000
Assumptions:
• The input temperature `celsius` is always a valid non-negative floating point number.
• Input: Input: celsius = 25.30
• Explanation: The Kelvin value is calculated by adding 273.15 to the Celsius value, giving 298.45. The Fahrenheit value is calculated by multiplying 25.30 by 1.80 and adding 32, which gives 77.54.
Approach: This problem is a straightforward application of the conversion formulas. First, we convert Celsius to Kelvin and then to Fahrenheit.
Observations:
• The formulas for Kelvin and Fahrenheit are linear and simple to compute.
• This problem requires basic arithmetic and handling floating point numbers.
Steps:
• Calculate the Kelvin value: celsius + 273.15.
• Calculate the Fahrenheit value: celsius * 1.80 + 32.00.
• Return the results as a list, ensuring each value is rounded to five decimal places.
Empty Inputs:
• The input `celsius` will never be empty as per the problem's constraints.
Large Inputs:
• For very large values of `celsius`, the formulas will still work within the provided constraints.
Special Values:
• If `celsius` is 0, the results should correctly return 273.15 Kelvin and 32.00 Fahrenheit.
Constraints:
• The solution needs to handle floating-point arithmetic carefully, especially with rounding to five decimal places.
vector<double> convertTemperature(double celsius) {
return { celsius + 273.15, celsius*1.80 + 32.00 };
}
1 : Function Declaration
vector<double> convertTemperature(double celsius) {
The function `convertTemperature` is declared, taking one parameter `celsius` which represents the temperature in Celsius.
2 : Return Statement
return { celsius + 273.15, celsius*1.80 + 32.00 };
The function returns a vector containing two converted temperatures: the first value is the temperature in Kelvin (Celsius + 273.15), and the second value is the temperature in Fahrenheit (Celsius * 1.80 + 32).
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The time complexity is constant since the solution involves a fixed number of arithmetic operations.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant as we only need to store two results (Kelvin and Fahrenheit).
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus