Leetcode 2466: Count Ways To Build Good Strings
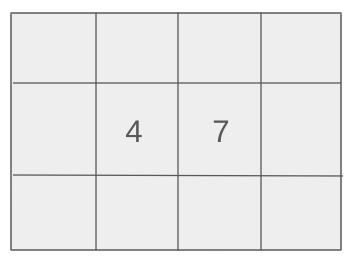
You are tasked with constructing binary strings using the characters ‘0’ and ‘1’. The string can be built by repeatedly appending ‘0’ zero times or ‘1’ one times, where ‘zero’ and ‘one’ are given values. The length of the final string must lie between ’low’ and ‘high’, inclusive. Your task is to find how many different binary strings can be created that meet these requirements. Return the count modulo 1e9 + 7.
Problem
Approach
Steps
Complexity
Input: You are provided with four integers: low, high, zero, and one. The integers 'low' and 'high' define the range for the possible lengths of the resulting binary string, while 'zero' and 'one' specify how many times the character '0' or '1' can be appended at each step.
Example: low = 2, high = 4, zero = 1, one = 2
Constraints:
• 1 <= low <= high <= 105
• 1 <= zero, one <= low
Output: Return the number of distinct binary strings that can be constructed such that their length lies between 'low' and 'high' inclusive. The answer should be returned modulo 1e9 + 7.
Example: For low = 2, high = 4, zero = 1, one = 2, the output is 6.
Constraints:
• The result should be an integer.
Goal: We need to calculate the number of distinct good binary strings that can be constructed using the provided constraints.
Steps:
• For each possible length between 'low' and 'high', calculate the number of ways to construct a binary string of that length using dynamic programming.
• Store previously computed results to avoid redundant calculations.
• Return the sum of the results modulo 1e9 + 7.
Goal: The given integers define constraints for the binary string construction process.
Steps:
• The array of integers must respect the conditions 1 <= low <= high <= 105 and 1 <= zero, one <= low.
Assumptions:
• The input will always respect the given constraints.
• Input: For low = 2, high = 4, zero = 1, one = 2
• Explanation: The good binary strings that can be formed are: '00', '11', '000', '011', '110', '111'. There are 6 distinct strings, so the output is 6.
Approach: The solution uses dynamic programming to efficiently count the number of distinct binary strings for each length between 'low' and 'high'.
Observations:
• This problem can be solved efficiently using dynamic programming to keep track of previously computed results for subproblems.
• Dynamic programming is the best approach here because it avoids recalculating the number of ways to form strings of the same length multiple times.
Steps:
• Initialize a dp array to store the number of ways to form binary strings of each length.
• Base case: dp[0] = 1 because an empty string is a valid string.
• For each target length, compute the number of ways to form it by appending '0' or '1' the respective number of times.
• Sum the results for all lengths between 'low' and 'high' and return the result modulo 1e9 + 7.
Empty Inputs:
• The problem guarantees that the input values will always be within the valid range.
Large Inputs:
• Ensure that the solution works within the time limits for inputs where high can be as large as 100,000.
Special Values:
• If zero equals one, the problem becomes simpler as the number of ways to form strings is identical for both cases.
Constraints:
• The solution needs to handle inputs where the difference between low and high can be large.
class Solution {
int mod = 1e9 + 7;
public:
int countGoodStrings(int low, int high, int zero, int one) {
vector<int> dp(high + 1, -1);
int ans = 0;
for(int i = low; i <= high; i++) {
ans = (ans + score(i, dp, zero, one)) % mod;
}
return ans;
}
int score(int target, vector<int> &dp, int zero, int one) {
if(target == 0) return 1;
if(target < 1 ) return 0;
if(dp[target] != -1) return dp[target];
long long sum = score(target - zero, dp, zero, one) + score(target - one, dp, zero, one);
return dp[target] = sum % mod;
}
1 : Class Definition
class Solution {
Defines the main class for the solution.
2 : Variable Initialization
int mod = 1e9 + 7;
Declares a constant modulus to handle large numbers and prevent overflow.
3 : Access Modifier
public:
Marks the following methods as accessible from outside the class.
4 : Function Definition
int countGoodStrings(int low, int high, int zero, int one) {
Defines the main function to count good strings within a range.
5 : Vector Initialization
vector<int> dp(high + 1, -1);
Initializes a DP array to store intermediate results, reducing redundant calculations.
6 : Variable Initialization
int ans = 0;
Initializes a variable to store the cumulative count of good strings.
7 : Loop
for(int i = low; i <= high; i++) {
Iterates through each number in the given range.
8 : DP Calculation
ans = (ans + score(i, dp, zero, one)) % mod;
Updates the result using the `score` function while applying modulus to avoid overflow.
9 : Return Statement
return ans;
Returns the total count of good strings.
10 : Recursive Function
int score(int target, vector<int> &dp, int zero, int one) {
Defines a helper function to recursively calculate the number of good strings for a target value.
11 : Base Case
if(target == 0) return 1;
Returns 1 if the target is 0, as an empty string is a valid good string.
12 : Base Case
if(target < 1 ) return 0;
Returns 0 if the target is negative, as it is not valid.
13 : Memoization Check
if(dp[target] != -1) return dp[target];
Checks if the value for the current target is already computed to avoid redundant calculations.
14 : Recursive Calculation
long long sum = score(target - zero, dp, zero, one) + score(target - one, dp, zero, one);
Recursively calculates the sum of ways to achieve the target using `zero` and `one` steps.
15 : Memoization Store
return dp[target] = sum % mod;
Stores the computed result for the current target in the DP array and returns it.
Best Case: O(n) where n is the difference between high and low.
Average Case: O(n)
Worst Case: O(n)
Description: The solution computes the number of ways to form strings for each length in the range between low and high.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because of the dynamic programming array used to store intermediate results.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus