Leetcode 2465: Number of Distinct Averages
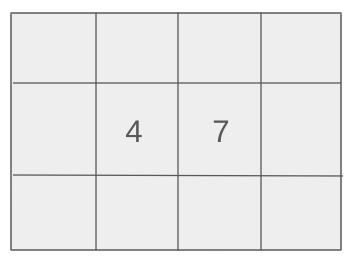
You are given an array of even length containing integers. In each step, repeatedly remove the smallest and the largest numbers from the array, calculate their average, and continue until the array is empty. The goal is to find how many distinct averages were calculated during this process.
Problem
Approach
Steps
Complexity
Input: You are given a list of integers called 'nums', where the length of 'nums' is always even.
Example: nums = [3, 8, 1, 6, 5, 7]
Constraints:
• 2 <= nums.length <= 100
• nums.length is even.
• 0 <= nums[i] <= 100
Output: Return the number of distinct averages calculated as per the process described.
Example: For nums = [3, 8, 1, 6, 5, 7], the output is 3.
Constraints:
• The result should be an integer.
Goal: To find the number of distinct averages by following the rule of removing the smallest and largest elements, calculating their average, and repeating until the list is empty.
Steps:
• Sort the array to easily access the minimum and maximum values.
• Remove the smallest and largest values from the array, calculate their average, and store the average in a set to ensure uniqueness.
• Repeat the process until the array is empty.
• Return the size of the set containing distinct averages.
Goal: Make sure to follow the rules for calculating distinct averages.
Steps:
• The array always has an even number of elements.
Assumptions:
• The length of the array is always even, so there will always be an even number of elements to pair up.
• Input: For nums = [3, 8, 1, 6, 5, 7]
• Explanation: In the first step, we remove 1 (min) and 8 (max), calculating their average 4.5. In the second step, we remove 3 (min) and 7 (max), calculating 5. Then, we remove 5 (min) and 6 (max), calculating 5.5. There are 3 distinct averages: 4.5, 5, and 5.5.
Approach: Sort the array and repeatedly remove the smallest and largest elements to compute the averages. Use a set to store the averages and return the size of the set.
Observations:
• Sorting the array simplifies accessing the minimum and maximum values.
• Using a set is crucial for ensuring uniqueness of averages.
Steps:
• Sort the input array.
• Initialize two pointers, one at the start and one at the end of the array.
• Remove the elements at these pointers, calculate their average, and store it in a set.
• Move the pointers inward and repeat the process until the array is empty.
Empty Inputs:
• The array will always have an even length and at least two elements.
Large Inputs:
• Ensure the solution can handle arrays of length up to 100.
Special Values:
• When all elements in the array are the same, all averages will be the same.
Constraints:
• The array will always have an even length.
int distinctAverages(vector<int>& nums) {
map<long, int> mp;
sort(nums.begin(), nums.end());
int i = 0, j = nums.size() - 1;
while(i < j){
int a = nums[j--];
int b = nums[i++];
long c = a + b;
mp[c] = 1;
}
return mp.size();
}
1 : Function Definition
int distinctAverages(vector<int>& nums) {
Defines a function to calculate distinct averages from the input vector `nums`.
2 : Map Initialization
map<long, int> mp;
Initializes a map to store unique sums of pairs.
3 : Sorting
sort(nums.begin(), nums.end());
Sorts the input array in ascending order to easily pair smallest and largest numbers.
4 : Variable Initialization
int i = 0, j = nums.size() - 1;
Initializes two pointers `i` and `j` to point to the smallest and largest elements in the array.
5 : Loop
while(i < j){
Begins a loop to pair the smallest and largest elements until the pointers meet.
6 : Element Access
int a = nums[j--];
Retrieves the largest element and decrements the pointer `j`.
7 : Element Access
int b = nums[i++];
Retrieves the smallest element and increments the pointer `i`.
8 : Sum Calculation
long c = a + b;
Calculates the sum of the smallest and largest elements.
9 : Map Insertion
mp[c] = 1;
Stores the sum in the map, ensuring uniqueness.
10 : Result
return mp.size();
Returns the number of unique sums stored in the map.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: Sorting the array takes O(n log n) time, and the subsequent process of removing elements and calculating averages is O(n).
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage required for the set of averages.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus