Leetcode 2460: Apply Operations to an Array
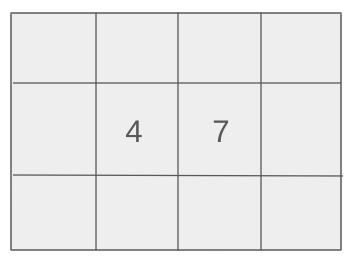
You are given an array of non-negative integers. You need to perform a series of operations on this array where, in each operation, you compare adjacent elements. If the elements are equal, you double the value of the first element and set the second element to zero. After performing these operations on all pairs, shift all the zeros to the end of the array. Return the resulting array.
Problem
Approach
Steps
Complexity
Input: The input consists of a single array nums containing non-negative integers.
Example: nums = [3, 3, 1, 1, 2, 0]
Constraints:
• 2 <= nums.length <= 2000
• 0 <= nums[i] <= 1000
Output: The output should be the array after performing the operations and moving all zeros to the end.
Example: For nums = [3, 3, 1, 1, 2, 0], the output should be [6, 2, 1, 1, 0, 0].
Constraints:
Goal: The goal is to perform the operations as described and shift zeros to the end.
Steps:
• 1. Iterate through the array and compare adjacent elements.
• 2. If elements are equal, double the first one and set the second to zero.
• 3. After all operations, shift all zeros in the array to the end.
Goal: The solution must handle arrays up to a length of 2000 efficiently.
Steps:
• The array will always contain at least two elements.
Assumptions:
• It is guaranteed that the operations will be valid and will not lead to any invalid state.
• Input: nums = [3, 3, 1, 1, 2, 0]
• Explanation: The operations proceed as follows:
- For i = 0, nums[0] and nums[1] are equal, so we double nums[0] and set nums[1] to 0. The array becomes [6, 0, 1, 1, 2, 0].
- For i = 1, nums[1] and nums[2] are not equal, so no operation is performed.
- For i = 2, nums[2] and nums[3] are equal, so we double nums[2] and set nums[3] to 0. The array becomes [6, 0, 2, 0, 2, 0].
- Finally, we shift all the zeros to the end, resulting in [6, 2, 1, 1, 0, 0].
Approach: We will perform the operations sequentially and adjust the array. The approach works by iterating over the array, applying the operations on adjacent elements, and shifting the zeros at the end once the operations are complete.
Observations:
• We need to identify and apply operations only when adjacent elements are equal.
• We can apply the operation in a single pass over the array, followed by another pass to move the zeros to the end.
Steps:
• 1. Iterate over the array to check adjacent elements.
• 2. If the elements are equal, double the first element and set the second element to zero.
• 3. After performing all operations, shift the zeros to the end by swapping non-zero elements with zeros.
Empty Inputs:
• The input will always have at least two elements, so there will never be an empty array.
Large Inputs:
• The algorithm should work efficiently for arrays up to the maximum length of 2000.
Special Values:
• If no adjacent elements are equal, no operations will be performed.
Constraints:
• The solution should be able to handle large inputs efficiently.
vector<int> applyOperations(vector<int>& A) {
for (int i = 0, j = 0; i < size(A); ++i){
if (i + 1 < size(A) and A[i] == A[i + 1]){
A[i] *= 2;
A[i + 1] = 0;
}
if (A[i]) swap(A[j++], A[i]);
}
return A;
}
1 : Function Definition
vector<int> applyOperations(vector<int>& A) {
Defines the function to process the array and return the modified version.
2 : Loop Initialization
for (int i = 0, j = 0; i < size(A); ++i){
Initializes a loop to iterate through the array, with `i` as the current index and `j` to track the position of non-zero elements.
3 : Condition Check
if (i + 1 < size(A) and A[i] == A[i + 1]){
Checks if the current element is equal to the next element to combine their values.
4 : Value Update
A[i] *= 2;
Doubles the value of the current element when it matches the next element.
5 : Set Zero
A[i + 1] = 0;
Sets the next element to zero after combining its value with the current element.
6 : Swap Non-Zero
if (A[i]) swap(A[j++], A[i]);
Swaps non-zero elements to the left of the array, maintaining their relative order.
7 : Return Statement
return A;
Returns the modified array after processing.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we iterate through the array twice, once to apply the operations and once to shift the zeros.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we use only a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus