Leetcode 2453: Destroy Sequential Targets
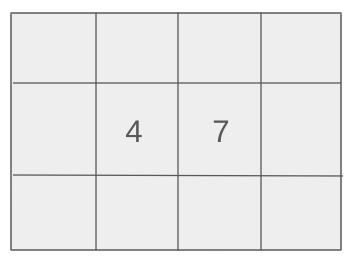
You are given a 0-indexed array ’nums’, where each element represents a target on a number line, and an integer ‘space’. A machine is available that can destroy all targets that can be represented by the formula nums[i] + c * space, where c is a non-negative integer. You need to choose an element from ’nums’ to seed the machine such that it destroys the maximum number of targets. Return the minimum value from ’nums’ that can seed the machine to destroy the most targets.
Problem
Approach
Steps
Complexity
Input: The input consists of an array 'nums' of positive integers and an integer 'space'.
Example: nums = [4, 8, 10, 2, 3, 6], space = 3
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
• 1 <= space <= 10^9
Output: Return the minimum value from 'nums' that can be used to seed the machine and destroy the maximum number of targets.
Example: Output: 2
Constraints:
• Return the smallest seed value in case of a tie.
Goal: Find the minimum seed value from 'nums' that can destroy the maximum number of targets by utilizing the 'space' parameter.
Steps:
• 1. For each target in 'nums', calculate the remainder when divided by 'space'.
• 2. Track the frequency of each remainder using a hash map.
• 3. Identify the remainder with the highest frequency, which corresponds to the seed value that can destroy the most targets.
• 4. In case of a tie (multiple remainders with the same frequency), return the smallest value that can seed the machine.
Goal: The problem should be solved efficiently within the provided constraints.
Steps:
• The number of elements in 'nums' can be as large as 10^5, and values can go up to 10^9.
• Efficient use of memory and time is essential due to large inputs.
Assumptions:
• The 'nums' array will always contain positive integers and there will be at least one element.
• Input: nums = [4, 8, 10, 2, 3, 6], space = 3
• Explanation: The remainder when each element in 'nums' is divided by 3 is: [4 % 3 = 1, 8 % 3 = 2, 10 % 3 = 1, 2 % 3 = 2, 3 % 3 = 0, 6 % 3 = 0]. The frequency of remainders is: {1: 2, 2: 2, 0: 2}. In this case, there is a tie. The smallest value with the highest frequency is 2. Thus, the answer is 2.
• Input: nums = [5, 10, 15, 3, 8], space = 4
• Explanation: The remainder when each element in 'nums' is divided by 4 is: [5 % 4 = 1, 10 % 4 = 2, 15 % 4 = 3, 3 % 4 = 3, 8 % 4 = 0]. The frequency of remainders is: {1: 1, 2: 1, 3: 2, 0: 1}. The value with the highest frequency is 3, so the answer is 3.
Approach: To solve this problem, we will use a hash map to store the frequency of remainders when elements in 'nums' are divided by 'space'. The goal is to find the most frequent remainder and select the smallest number associated with it.
Observations:
• We need to count how often each remainder occurs when we divide each number in 'nums' by 'space'.
• The key insight is that numbers with the same remainder when divided by 'space' can destroy similar targets, so we focus on maximizing the frequency of remainders.
Steps:
• 1. Initialize a hash map to track the frequency of each remainder when elements in 'nums' are divided by 'space'.
• 2. Loop through 'nums' and calculate the remainder for each element.
• 3. Update the frequency of the remainder in the hash map.
• 4. Identify the remainder with the maximum frequency.
• 5. For ties, return the smallest number from 'nums' that produces this remainder.
Empty Inputs:
• If 'nums' is empty, return an error or handle the case as appropriate.
Large Inputs:
• The solution should handle up to 10^5 elements efficiently.
Special Values:
• If all elements in 'nums' have the same value, the solution should return that value.
Constraints:
• The solution should work efficiently with the maximum possible values for 'nums' and 'space'.
int destroyTargets(vector<int>& nums, int space) {
int ans = INT_MAX;
unordered_map<int, int> mp;
int mx = INT_MIN;
for(int n: nums) {
int r = n % space;
mp[r]++;
if(mx < mp[r]) mx = mp[r];
}
for(int n : nums)
if(mx == mp[n %space]) ans = min(ans, n);
return ans;
}
1 : Function Definition
int destroyTargets(vector<int>& nums, int space) {
Defines the function `destroyTargets` that takes a vector of integers `nums` and an integer `space`, and returns the smallest integer from `nums` that satisfies the problem condition.
2 : Variable Initialization
int ans = INT_MAX;
Initializes `ans` to the maximum possible value (INT_MAX) to later find the minimum value that satisfies the condition.
3 : Data Structure Initialization
unordered_map<int, int> mp;
Initializes an unordered map `mp` to store the count of occurrences of each remainder when the elements of `nums` are divided by `space`.
4 : Variable Initialization
int mx = INT_MIN;
Initializes `mx` to the smallest possible integer value (INT_MIN) to keep track of the maximum frequency of any remainder.
5 : First Loop (Counting Remainders)
for(int n: nums) {
Starts a loop to iterate through each element `n` in the `nums` list.
6 : Remainder Calculation
int r = n % space;
Calculates the remainder `r` when the element `n` is divided by `space`.
7 : Map Update
mp[r]++;
Increments the count of the remainder `r` in the map `mp`.
8 : Frequency Update
if(mx < mp[r]) mx = mp[r];
Updates the value of `mx` if the frequency of remainder `r` exceeds the current maximum frequency.
9 : Second Loop (Finding the Minimum)
for(int n : nums)
Starts a second loop to iterate over the `nums` list again to find the smallest number that satisfies the condition.
10 : Condition Check
if(mx == mp[n %space]) ans = min(ans, n);
If the frequency of the remainder of `n` matches the maximum frequency `mx`, updates `ans` to the smaller of its current value or `n`.
11 : Return Statement
return ans;
Returns the smallest number `ans` that satisfies the condition.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: We only need to loop through the array once to calculate remainders and update the hash map.
Best Case: O(n)
Worst Case: O(n)
Description: We use additional space for the hash map to store remainders, which is proportional to the size of the input.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus