Leetcode 2437: Number of Valid Clock Times
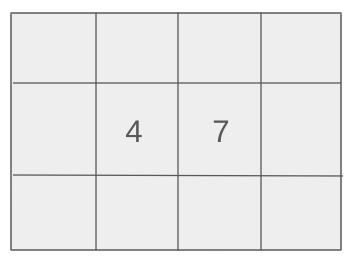
You are given a string
time
representing the current time on a 24-hour digital clock in the format ‘hh:mm’, where some digits are replaced with the ‘?’ symbol. The ‘?’ character can be replaced with any digit from 0 to 9. Your task is to return the total number of valid clock times that can be formed by replacing all ‘?’ characters in the string.Problem
Approach
Steps
Complexity
Input: The input string `time` is a valid time string of length 5 in the format 'hh:mm', with some digits replaced by the '?' character.
Example: time = "?3:15"
Constraints:
• 1 <= length of `time` <= 5
• The string is a valid 24-hour time format, i.e., 00 <= hh <= 23 and 00 <= mm <= 59.
• Any '?' in the string can be replaced with a digit from 0 to 9.
Output: Return an integer representing the number of valid times that can be formed by replacing '?' with digits from 0 to 9.
Example: Output: 9
Constraints:
• The output is the total number of valid time combinations.
Goal: We need to count all valid times that can be formed by replacing '?' with digits while respecting the constraints of the 24-hour clock format.
Steps:
• 1. Iterate through each character in the `time` string.
• 2. If the character is a '?', calculate how many valid digits can replace it based on its position in the time format (hours or minutes).
• 3. Multiply the possibilities for each '?' to get the total number of valid times.
• 4. Return the final count of valid combinations.
Goal: Consider the following constraints while designing the solution.
Steps:
• The `time` string is always in a valid 24-hour format.
• Only the '?' symbols are variable, and they must be replaced with digits that form a valid time.
Assumptions:
• The input string `time` follows the 24-hour format, where hours range from '00' to '23' and minutes range from '00' to '59'.
• The input string is guaranteed to be a valid time representation with '?' representing unknown digits.
• Input: time = "?5:00"
• Explanation: For the time `?5:00`, the first '?' can be replaced with either '0' or '1', but not '2' because the hour cannot be '25'. This gives two valid times: '05:00' and '15:00'.
• Input: time = "0?:0?"
• Explanation: In the time `0?:0?`, the first '?' can be replaced with any digit from 0 to 9, but the second '?' can also be any digit. This results in 100 possible valid times.
Approach: We need to calculate the number of valid time representations that can be formed by replacing '?' in the string with digits from 0 to 9, ensuring the resulting time remains valid under the 24-hour format.
Observations:
• The number of valid time possibilities depends on the positions of the '?' characters in the hours and minutes part of the time.
• We need to carefully handle the replacement of '?' depending on whether it's in the hour or minute part of the time string.
Steps:
• 1. Initialize a variable `ans` to 1, which will hold the total number of valid time combinations.
• 2. If the minute's second digit is '?', multiply `ans` by 10 (since any digit from 0 to 9 can replace it).
• 3. If the minute's first digit is '?', multiply `ans` by 6 (since it can be 0, 1, 2, 3, 4, or 5).
• 4. Handle the hour part: if both digits of the hour are '?', multiply `ans` by 24 (for 24 possible hours).
• 5. If the hour's second digit is '?', handle the first digit of the hour to ensure the resulting time is valid (either 0, 1, or 2).
• 6. Return the total count of valid time combinations.
Empty Inputs:
• The input will always be a valid time string, so no empty inputs need to be considered.
Large Inputs:
• This problem does not involve large inputs outside of the fixed string size.
Special Values:
• If '?' appears at the first digit of the hour, it must be replaced by a valid number based on the second digit's value.
Constraints:
• Ensure that the number of possibilities is always calculated in a way that respects the limits of a 24-hour time format.
int countTime(string time) {
int ans = 1;
if(time[4] == '?') ans = ans * 10;
if(time[3] == '?') ans = ans * 6;
if(time[0] == '?' && time[1] == '?') ans = ans * 24;
else{
if(time[1] == '?'){
if(time[0] == '2' ) ans = ans * 4;
else ans = ans * 10;
}
if(time[0] == '?'){
if(time[1] < '4') ans = ans * 3;
else ans = ans * 2;
}
}
return ans;
}
1 : Function Definition
int countTime(string time) {
This line defines the function `countTime` which takes a string `time` as input and returns an integer representing the number of valid times.
2 : Variable Initialization
int ans = 1;
The variable `ans` is initialized to 1. It will store the result of the calculations based on the input time string.
3 : Conditional Check
if(time[4] == '?') ans = ans * 10;
If the 5th character in the time string (index 4) is a '?' symbol, it can represent any digit between 0 and 9, so we multiply the answer by 10.
4 : Conditional Check
if(time[3] == '?') ans = ans * 6;
If the 4th character in the time string (index 3) is a '?' symbol, it can represent any digit between 0 and 5 (to form a valid minute), so we multiply the answer by 6.
5 : Conditional Check
if(time[0] == '?' && time[1] == '?') ans = ans * 24;
If both the 1st and 2nd characters of the time string are '?', the hour can range from 00 to 23, so we multiply the answer by 24.
6 : Else Condition
else{
If the first two characters are not both '?', the function checks individual components of the hour.
7 : Conditional Check
if(time[1] == '?'){
If only the second character in the hour (minute's tens place) is '?', the function checks the first character.
8 : Conditional Check
if(time[0] == '2' ) ans = ans * 4;
If the first character of the hour is '2', the second character can only be 0, 1, 2, or 3, so we multiply the answer by 4.
9 : Conditional Check
else ans = ans * 10;
If the first character of the hour is not '2', the second character can range from 0 to 9, so we multiply the answer by 10.
10 : Conditional Check
if(time[0] == '?'){
If the first character in the hour (hour's tens place) is '?', we check the value of the second character.
11 : Conditional Check
if(time[1] < '4') ans = ans * 3;
If the second character is less than '4', the first character can be 0, 1, or 2, so we multiply the answer by 3.
12 : Conditional Check
else ans = ans * 2;
If the second character is 4 or greater, the first character can only be 0 or 1, so we multiply the answer by 2.
13 : Return Statement
return ans;
The function returns the final computed value of `ans`, representing the total number of valid times that can be formed.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The time complexity is O(1) as the string length is constant (5 characters), and the solution requires only a few conditional checks.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since no extra space is required, apart from a few integer variables.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus