Leetcode 2423: Remove Letter To Equalize Frequency
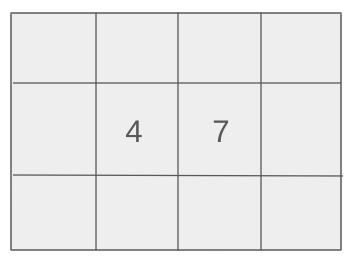
You are given a string consisting of lowercase English letters. You must remove one letter from the string so that the frequency of all remaining letters is equal. Return true if it is possible to achieve this by removing exactly one letter, otherwise return false.
Problem
Approach
Steps
Complexity
Input: You are given a string word consisting of lowercase English letters.
Example: word = "abcc"
Constraints:
• 2 <= word.length <= 100
• word consists of lowercase English letters only.
Output: Return true if it is possible to remove one letter so that all remaining letters have equal frequency, otherwise return false.
Example: Output: true
Constraints:
Goal: Determine if it is possible to remove one letter so that all remaining characters have equal frequencies.
Steps:
• 1. Count the frequency of each letter in the string.
• 2. Count how many times each frequency appears in the string.
• 3. If there are more than two distinct frequencies, return false.
• 4. If there is only one frequency, check if all letters have this frequency or if one letter appears once (removable).
• 5. If the two frequencies differ by 1 and one letter has that frequency, it can be removed to balance frequencies, return true.
Goal: The solution must handle strings of size up to 100 efficiently.
Steps:
• Ensure the solution works within time limits for string lengths up to 100.
Assumptions:
• The string will always contain at least 2 characters.
• Input: Input: word = "abcc"
• Explanation: By removing the last 'c', the frequency of 'a', 'b', and 'c' becomes equal (1 for each). Hence, the answer is true.
• Input: Input: word = "aazz"
• Explanation: It is impossible to remove one letter and make the frequency of all characters equal because the frequencies of 'a' and 'z' differ, and neither can be reduced to equal the other.
Approach: We need to check if it's possible to remove one character so that the frequencies of all remaining characters are equal. This can be done by analyzing the frequency distribution of characters in the string.
Observations:
• The problem requires checking how frequencies of characters are distributed.
• By counting frequencies and the frequency of frequencies, we can easily determine if removing a letter will balance the remaining frequencies.
Steps:
• 1. Use a hash map to store the frequency of each character in the string.
• 2. Use a second map to count how many times each frequency appears.
• 3. Analyze the frequencies: if more than two distinct frequencies exist, return false.
• 4. If only one frequency exists, check if the string consists of identical letters or if one letter appears once.
• 5. If two frequencies exist and differ by 1, check if the letter with the higher frequency occurs only once.
Empty Inputs:
• The problem guarantees that word will have at least 2 characters.
Large Inputs:
• The solution should efficiently handle strings of length 100.
Special Values:
• Check for cases where only one letter appears multiple times and all others appear once.
Constraints:
• Ensure that time complexity is O(n) for counting frequencies.
bool equalFrequency(string word) {
unordered_map<char, int> mp;
map<int, int> mp2;
for(auto c: word) mp[c]++;
for(auto m: mp) mp2[m.second]++;
if(mp2.size() > 2) return false;
map<int, int>::iterator it1 = mp2.begin();
map<int, int>::iterator it2 = mp2.begin();
it2++;
if(mp2.size() == 1){
if(mp.size() == 1 || it1->first == 1) return true;
return false;
}
if(it1->first == 1 && it1->second == 1) return true;
if(it1->first == it2->first-1 && it2->second == 1) return true;
return false;
}
1 : Function Definition
bool equalFrequency(string word) {
Defines the function 'equalFrequency' which accepts a string and returns a boolean value indicating whether it is possible to equalize the frequency of characters in the string.
2 : Data Structure Initialization
unordered_map<char, int> mp;
Declares an unordered map 'mp' to store the frequency count of each character in the word.
3 : Data Structure Initialization
map<int, int> mp2;
Declares a map 'mp2' to store how many times each frequency appears.
4 : Frequency Counting
for(auto c: word) mp[c]++;
Loops through each character in the word, incrementing its count in the map 'mp'.
5 : Frequency Counting
for(auto m: mp) mp2[m.second]++;
Iterates over the map 'mp' to fill 'mp2' with the frequency of each frequency.
6 : Validation
if(mp2.size() > 2) return false;
If there are more than two different frequencies, return false as it is impossible to make the frequencies equal by removing or modifying one character.
7 : Iterator Setup
map<int, int>::iterator it1 = mp2.begin();
Creates an iterator 'it1' pointing to the beginning of the 'mp2' map.
8 : Iterator Setup
map<int, int>::iterator it2 = mp2.begin();
Creates another iterator 'it2' also pointing to the beginning of the 'mp2' map.
9 : Iterator Adjustment
it2++;
Moves the second iterator 'it2' to the second element in the 'mp2' map.
10 : Condition Check
if(mp2.size() == 1){
Checks if there is only one frequency in 'mp2', indicating all characters have the same frequency.
11 : Condition Check
if(mp.size() == 1 || it1->first == 1) return true;
If there is only one character or all characters have a frequency of 1, return true (it is possible to equalize frequencies).
12 : Condition Check
return false;
If neither of the previous conditions are met, return false.
13 : Final Condition
if(it1->first == 1 && it1->second == 1) return true;
If the frequency of 1 appears exactly once, return true as it can be equalized by removing a single character.
14 : Final Condition
if(it1->first == it2->first-1 && it2->second == 1) return true;
If the difference between the two frequencies is 1 and the second frequency occurs exactly once, return true.
15 : Final Condition
return false;
Return false if none of the conditions for equalizing the frequencies are met.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) where n is the length of the string, as we need to traverse the string and perform frequency analysis.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the two hash maps used for counting character and frequency distributions.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus