Leetcode 2413: Smallest Even Multiple
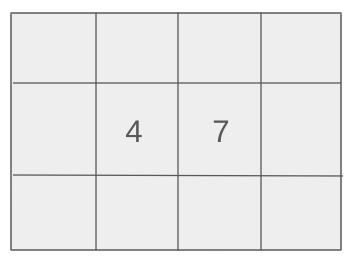
Given a positive integer ’n’, return the smallest positive integer that is a multiple of both 2 and ’n'.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer 'n'.
Example: n = 7
Constraints:
• 1 <= n <= 150
Output: Return the smallest integer that is a multiple of both 2 and 'n'.
Example: Output: 14
Constraints:
Goal: The goal is to find the smallest integer that is divisible by both 2 and 'n'.
Steps:
• 1. Check if 'n' is already divisible by 2.
• 2. If 'n' is even, return 'n'.
• 3. If 'n' is odd, return 'n * 2' because the next multiple of both 2 and 'n' will be twice 'n'.
Goal: The solution must efficiently handle values of 'n' up to 150.
Steps:
• 1 <= n <= 150
Assumptions:
• The input is a positive integer.
• The problem ensures that 'n' is always between 1 and 150.
• Input: n = 7
• Explanation: For 'n' = 7, the smallest multiple of both 2 and 7 is 14. For 'n' = 6, since 6 is already divisible by 2, the result is 6.
Approach: The solution uses a simple check to determine if 'n' is already divisible by 2. If not, we double 'n' to get the smallest multiple of both 2 and 'n'.
Observations:
• The smallest multiple of both 2 and any integer can be obtained by checking if the number is even.
• If the number is odd, we just need to multiply it by 2.
• This approach is straightforward and runs in constant time, making it very efficient.
Steps:
• 1. Check if 'n' is divisible by 2.
• 2. If 'n' is even, return 'n'.
• 3. If 'n' is odd, return 'n * 2'.
Empty Inputs:
• The input will always be a positive integer as per the problem constraints.
Large Inputs:
• The input is constrained to a maximum of 150, so there are no concerns regarding large inputs.
Special Values:
• If 'n' is 1, the smallest multiple of both 2 and 1 is 2.
Constraints:
• Make sure the solution handles both even and odd numbers correctly.
int smallestEvenMultiple(int n) {
return n % 2 == 0? n: n * 2;
}
1 : Function Declaration
int smallestEvenMultiple(int n) {
Function declaration for 'smallestEvenMultiple'. It takes an integer 'n' as input.
2 : Return Statement
return n % 2 == 0? n: n * 2;
The function checks if 'n' is even. If true, it returns 'n'; otherwise, it returns 'n' multiplied by 2 to make it even.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The time complexity is constant because only a few arithmetic operations are needed.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant, requiring only a small amount of space to store the result.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus