Leetcode 2411: Smallest Subarrays With Maximum Bitwise OR
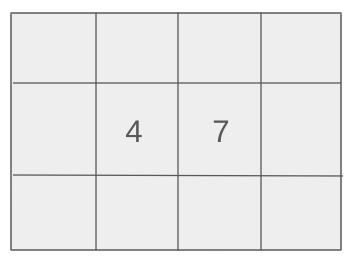
Given a 0-indexed array of non-negative integers, ’nums’, you need to find the length of the smallest subarray starting at each index that has the maximum possible bitwise OR. For each index ‘i’, find the minimum length subarray nums[i…j] such that the bitwise OR of this subarray equals the maximum OR value possible starting from index ‘i’.
Problem
Approach
Steps
Complexity
Input: The input consists of a single array, 'nums', of non-negative integers.
Example: nums = [3,1,2,5]
Constraints:
• 1 <= n <= 10^5
• 0 <= nums[i] <= 10^9
Output: Return an array where each element at index 'i' represents the size of the minimum sized subarray starting from index 'i' with the maximum bitwise OR.
Example: Output: [3,2,2,1]
Constraints:
Goal: The goal is to find the minimum length subarray starting at index 'i' whose OR is maximized among all subarrays starting at indices greater than or equal to 'i'.
Steps:
• 1. Initialize a result array with size 'n' where each element is initially set to 1.
• 2. Track the last occurrence of each bit in the binary representation of the elements.
• 3. Starting from the last element, calculate the bitwise OR for each subarray starting at the current index and update the answer accordingly.
• 4. Use bitwise OR operation and last position tracking to efficiently compute the result for each index.
Goal: Ensure that the solution works efficiently with the constraints given the maximum input sizes.
Steps:
• The solution should run in O(n) time for efficient processing of large inputs.
Assumptions:
• The input array is non-empty, and all numbers are non-negative integers.
• Subarrays must contain at least one element.
• Input: nums = [3,1,2,5]
• Explanation: For index 0, the maximum OR is 7. The shortest subarray starting at index 0 is [3, 1, 2], which has an OR of 7. For index 1, the OR is maximized by [1, 2], and for index 2, it is maximized by [2, 5]. Thus, the result is [3, 2, 2, 1].
Approach: The solution uses a greedy approach with bitwise OR operations and last position tracking to minimize the subarray size.
Observations:
• Bitwise OR operations combine all set bits, and the maximum OR for a subarray will involve including all contributing bits.
• The approach needs to track the last occurrence of set bits to determine the shortest subarray that gives the maximum OR.
• Efficient handling of bitwise operations and subarray size computation will be key to solving the problem within the given constraints.
Steps:
• 1. Initialize an array to store the results, and an array to track the last occurrence of each bit.
• 2. Iterate through the array from right to left, updating the OR result for each subarray and adjusting the result array for each index.
• 3. For each index, calculate the maximum OR and track the smallest subarray that reaches this maximum.
Empty Inputs:
• The array will not be empty as per the problem constraints.
Large Inputs:
• The solution must efficiently handle large inputs (up to 100,000 elements) by using a linear time complexity approach.
Special Values:
• If all elements in the array are the same, the result will be the same length for each index.
Constraints:
• Handling large numbers efficiently, as bitwise operations should be performed on integers within the range 0 to 10^9.
vector<int> smallestSubarrays(vector<int>& nums) {
int n = nums.size();
vector<int> ans(n, 1), last(30, 0);
for(int i = n - 1; i >= 0; i--) {
for(int j = 0; j < 30; j++) {
if(nums[i] & (1 << j)) {
last[j] = i;
}
ans[i] = max(ans[i], last[j] - i + 1);
}
}
return ans;
}
1 : Function Declaration
vector<int> smallestSubarrays(vector<int>& nums) {
Function declaration to find the smallest subarray for each element of the input array 'nums'.
2 : Variable Initialization
int n = nums.size();
Initializing 'n' as the size of the input array 'nums'.
3 : Variable Initialization
vector<int> ans(n, 1), last(30, 0);
Declaring and initializing 'ans' to store the answer for each element and 'last' to keep track of the last index of a set bit.
4 : Loop
for(int i = n - 1; i >= 0; i--) {
Starting the loop from the last element of the array and iterating backwards.
5 : Inner Loop
for(int j = 0; j < 30; j++) {
Inner loop iterating over the possible bit positions (0-29).
6 : Condition Check
if(nums[i] & (1 << j)) {
Checking if the j-th bit is set (1) in the current number 'nums[i]'.
7 : Update
last[j] = i;
Updating the 'last' array to store the current index for the j-th set bit.
8 : Update
ans[i] = max(ans[i], last[j] - i + 1);
Updating the 'ans' array to hold the size of the smallest subarray that includes the current set bit.
9 : Return Statement
return ans;
Returning the final array 'ans' which contains the size of the smallest subarray for each element.
Best Case: O(n), where n is the size of the input array.
Average Case: O(n), since each element is processed once.
Worst Case: O(n), the worst case is still linear due to the single pass through the array and constant time bitwise operations.
Description: The approach runs in linear time, processing each element once and performing constant-time bitwise operations.
Best Case: O(n), the space complexity remains linear in all cases.
Worst Case: O(n), for the result array and last occurrence tracking arrays.
Description: The space complexity is linear, requiring space for the result array and bitwise tracking arrays.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus