Leetcode 2405: Optimal Partition of String
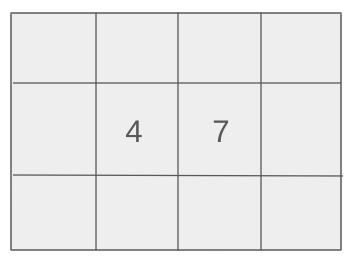
You are given a string
s
. Your task is to partition this string into the fewest number of substrings such that no character appears more than once in each substring. Each character must belong to exactly one substring in the partition.Problem
Approach
Steps
Complexity
Input: The input consists of a string `s` made up of only lowercase English letters.
Example: s = 'abcabc'
Constraints:
• 1 <= s.length <= 10^5
• s consists only of lowercase English letters
Output: Return the minimum number of substrings in such a partition.
Example: Output: 2
Constraints:
Goal: The goal is to minimize the number of substrings in the partition while ensuring each substring contains only unique characters.
Steps:
• 1. Use a bitmask to track characters that have appeared in the current substring.
• 2. Iterate through the string and check if the current character is already in the current substring (tracked by the bitmask).
• 3. If a character repeats, start a new substring and reset the bitmask.
• 4. Count the number of substrings formed during the process.
Goal: The solution should work efficiently for large input sizes (up to 10^5).
Steps:
• The solution should be efficient with time complexity O(n) for input strings of length up to 10^5.
Assumptions:
• The input string contains only lowercase English letters.
• The input string is non-empty, with a length between 1 and 10^5.
• Input: s = 'abacaba'
• Explanation: The partition could be ['a', 'ba', 'cab', 'a'], forming 4 substrings. The character 'a' repeats, so we need to partition it into multiple substrings.
• Input: s = 'ssssss'
• Explanation: The only valid partition is ['s', 's', 's', 's', 's', 's'], where each 's' is in a separate substring due to its repetition.
• Input: s = 'abcabc'
• Explanation: The optimal partition is ['abc', 'abc'], forming 2 substrings, where all characters in each substring are unique.
Approach: We use a bitmask to efficiently track characters that have been included in the current substring. By resetting the bitmask whenever we encounter a duplicate character, we can minimize the number of substrings.
Observations:
• We need to keep track of characters seen so far in the current substring.
• Using a bitmask allows us to track up to 26 characters efficiently.
• A bitmask allows for quick checks and resets without the need for additional data structures like sets or arrays.
Steps:
• 1. Initialize a bitmask (integer flag) to track characters in the current substring.
• 2. Iterate through the string, for each character, check if it is already in the current substring by inspecting the bitmask.
• 3. If the character is already in the bitmask, start a new substring (reset the bitmask) and increase the substring count.
• 4. Continue until the entire string is processed.
Empty Inputs:
• There are no empty inputs as per the problem constraints, but an empty string would theoretically result in 0 substrings.
Large Inputs:
• For large inputs up to 10^5 characters, the solution should run efficiently in O(n) time.
Special Values:
• If the string contains only one unique character (e.g., 'aaaa'), the result will be the length of the string, as each character must be in a separate substring.
Constraints:
• The solution must handle inputs up to 10^5 characters efficiently with O(n) time complexity.
bool checkBit(int &flag, int &n) {
return flag & (1<< n);
}
void setBit(int &flag, int & n) {
flag |= (1<<n);
}
int partitionString(string s) {
int flag = 0, ans = 1;
for(auto c: s) {
int n = c - 'a';
if(checkBit(flag, n)) {
flag = 0;
ans++;
}
setBit(flag, n);
}
return ans;
}
1 : Function Declaration
bool checkBit(int &flag, int &n) {
This function checks if the nth bit in `flag` is set. It returns true if the bit is set, indicating that the character corresponding to `n` has already appeared.
2 : Bitwise Check
return flag & (1<< n);
Performs a bitwise AND operation to check if the nth bit of `flag` is set. If the bit is set, it means that the character has been encountered before.
3 : Function Declaration
void setBit(int &flag, int & n) {
This function sets the nth bit of `flag` to 1, marking the character corresponding to `n` as encountered.
4 : Bitwise Set
flag |= (1<<n);
Performs a bitwise OR operation to set the nth bit of `flag`. This indicates that the character corresponding to `n` has been seen.
5 : Function Declaration
int partitionString(string s) {
This function partitions the string `s` into the least number of substrings where each substring contains unique characters.
6 : Variable Initialization
int flag = 0, ans = 1;
Initialize `flag` to 0 (to track which characters have been encountered) and `ans` to 1 (to start the count of substrings).
7 : Loop Through String
for(auto c: s) {
Loop through each character in the string `s`.
8 : Character Mapping
int n = c - 'a';
Convert the character `c` to an integer `n` representing its position in the alphabet, where 'a' corresponds to 0, 'b' to 1, and so on.
9 : Check for Duplicate Character
if(checkBit(flag, n)) {
Check if the character corresponding to `n` has been encountered previously by calling the `checkBit` function.
10 : Reset and Increment Substring Count
flag = 0;
Reset the `flag` to 0, starting a new substring as a duplicate character was found.
11 : Increment Substring Count
ans++;
Increment the substring count `ans` as a new substring is started.
12 : Set Current Character Bit
setBit(flag, n);
Mark the current character `n` as encountered by calling the `setBit` function.
13 : Return Statement
return ans;
Return the count of substrings `ans`, which is the minimum number of substrings where each substring contains unique characters.
Best Case: O(n), where n is the length of the string, as we only need to process each character once.
Average Case: O(n), since we process every character in the string in linear time.
Worst Case: O(n), where the worst case is when no characters repeat and each character requires a separate substring.
Description: The solution is linear in time complexity, making it efficient for input strings up to the maximum length.
Best Case: O(1), as the space required does not depend on the input size but rather on the number of characters (26 for English letters).
Worst Case: O(1), since we only use a constant amount of space to track characters with the bitmask.
Description: The space complexity is constant, as we only use a bitmask to track characters in the substring.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus