Leetcode 2400: Number of Ways to Reach a Position After Exactly k Steps
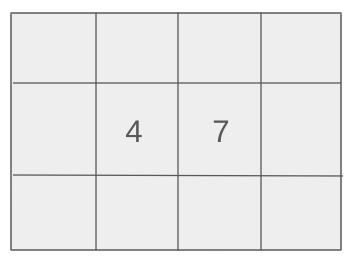
You are standing at a starting position
startPos
on an infinite number line, and you need to reach a destination endPos
in exactly k
steps. In each step, you can move either one position to the left or one position to the right. Your task is to find the number of different ways to reach endPos
from startPos
in exactly k
steps. Since the number of ways can be very large, return the result modulo 10^9 + 7.Problem
Approach
Steps
Complexity
Input: You are given the integers `startPos`, `endPos`, and `k`. These represent the starting position, the destination, and the number of steps respectively.
Example: startPos = 3, endPos = 5, k = 4
Constraints:
• 1 <= startPos, endPos, k <= 1000
Output: Return the number of ways to reach `endPos` from `startPos` in exactly `k` steps, modulo 10^9 + 7.
Example: Output: 4
Constraints:
Goal: The goal is to find the number of valid ways to move from `startPos` to `endPos` in exactly `k` steps, adhering to the movement rules (left or right in each step).
Steps:
• 1. Calculate the absolute difference `d` between `startPos` and `endPos`.
• 2. Use dynamic programming or recursion with memoization to count the number of ways to reach the target by moving either left or right.
• 3. Return the count modulo 10^9 + 7.
Goal: The problem constraints ensure that the input values are within a manageable range, making it feasible to solve using dynamic programming or recursion with memoization.
Steps:
• The length of `startPos`, `endPos`, and `k` is limited to 1000, which ensures that brute force solutions are not feasible and dynamic programming is necessary.
Assumptions:
• The input values are valid and within the specified range.
• The number of ways can be very large, so modulo operation must be used.
• Input: startPos = 1, endPos = 2, k = 3
• Explanation: We can reach position 2 from 1 in exactly 3 steps in three distinct ways: 1 -> 2 -> 3 -> 2, 1 -> 2 -> 1 -> 2, and 1 -> 0 -> 1 -> 2. Therefore, the output is 3.
• Input: startPos = 2, endPos = 5, k = 10
• Explanation: It is impossible to reach position 5 from position 2 in exactly 10 steps. Hence, the output is 0.
Approach: The approach involves using a dynamic programming technique or recursion with memoization to count the number of valid ways to move from `startPos` to `endPos` in `k` steps.
Observations:
• The problem can be reduced to finding paths with exactly `k` steps on a number line, which can be modeled as a dynamic programming problem.
• We need to calculate the number of paths efficiently, considering both left and right moves at each step.
Steps:
• 1. Define a recursive function `dfs(k, d)` where `k` is the remaining number of steps and `d` is the current distance from `startPos` to `endPos`.
• 2. Implement memoization to store results of subproblems and avoid redundant calculations.
• 3. Return the number of ways modulo 10^9 + 7.
Empty Inputs:
• This problem does not have an empty input case since the input size is guaranteed to be within the range 1 to 1000.
Large Inputs:
• For large values of `k`, the recursive approach should be optimized using dynamic programming or memoization to avoid recalculating results for the same subproblems.
Special Values:
• If `startPos` and `endPos` are the same, we must ensure that we move in such a way that we still perform exactly `k` steps.
Constraints:
• Dynamic programming or memoization should be used to efficiently handle inputs close to the upper limit.
int numberOfWays(int start, int end, int k) {
return dfs(k, abs(start - end));
}
int dfs(int k, int d) {
if (d >= k) return d == k;
if(dp[k][d] == 0)
dp[k][d] = (1 + dfs(k-1, d + 1) + dfs(k -1, abs(d -1))) % mod;
return dp[k][d] -1;
}
1 : Function Definition
int numberOfWays(int start, int end, int k) {
Defines the function `numberOfWays` which takes three parameters: `start`, `end`, and `k`. The function will return the number of ways to reach from `start` to `end` with `k` steps.
2 : Recursive Call
return dfs(k, abs(start - end));
This line calls the helper function `dfs`, passing `k` and the absolute difference between `start` and `end` as arguments.
3 : Helper Function Definition
int dfs(int k, int d) {
Defines the helper function `dfs`, which takes two parameters: `k` (number of steps remaining) and `d` (the current distance to cover). It will return the number of ways to complete the journey recursively.
4 : Base Case Check
if (d >= k) return d == k;
Checks if the distance `d` is greater than or equal to `k`. If it is, it checks if `d` is exactly equal to `k` and returns 1 if true (base case), otherwise returns 0.
5 : Memoization Check
if(dp[k][d] == 0)
Checks if the value of `dp[k][d]` has been previously computed. If it hasn't been computed (i.e., it is 0), the code proceeds to calculate it.
6 : Recursion and Memoization
dp[k][d] = (1 + dfs(k-1, d + 1) + dfs(k -1, abs(d -1))) % mod;
Calculates the number of ways recursively by making two recursive calls to `dfs`: one where the distance increases by 1 (`d + 1`) and another where the distance decreases by 1 (`abs(d - 1)`). The result is stored in `dp[k][d]` and taken modulo `mod`.
7 : Return Value
return dp[k][d] -1;
Returns the computed result from the `dp` array, decrementing it by 1 (to account for the last unnecessary computation step).
Best Case: O(k), where k is the number of steps. This is when the number of recursive calls is minimized due to memoization.
Average Case: O(k * d), where d is the distance between `startPos` and `endPos`. This depends on the recursive branching.
Worst Case: O(k * d), where d is the maximum possible distance. Memoization ensures that redundant calculations are avoided.
Description: The time complexity is determined by the number of recursive calls and the distance between `startPos` and `endPos`.
Best Case: O(1), if no memoization is used and the problem is solved directly.
Worst Case: O(k * d), where d is the distance between `startPos` and `endPos`. This is the space used by the memoization table.
Description: The space complexity is primarily determined by the size of the memoization table.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus