Leetcode 2380: Time Needed to Rearrange a Binary String
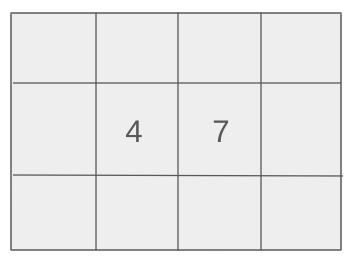
You are given a binary string
s
. In one second, all occurrences of the substring ‘01’ are simultaneously replaced with ‘10’. This process repeats until no occurrences of ‘01’ exist in the string. The task is to return the number of seconds required to complete this process.Problem
Approach
Steps
Complexity
Input: The input consists of a binary string `s` where each character is either '0' or '1'.
Example: s = '0101011'
Constraints:
• 1 <= s.length <= 1000
• s[i] is either '0' or '1'.
Output: Return the number of seconds required to ensure that no occurrences of '01' exist in the string.
Example: Output: 3
Constraints:
Goal: The goal is to simulate the process of replacing all occurrences of '01' with '10' and count the number of seconds until no such occurrences remain.
Steps:
• 1. Traverse the string and look for occurrences of '01'.
• 2. Swap every occurrence of '01' to '10'.
• 3. Count each second where at least one swap occurs.
• 4. Repeat until no '01' substrings remain in the string.
Goal: The input string will have a length between 1 and 1000, and each character will be either '0' or '1'.
Steps:
• The length of `s` is between 1 and 1000.
• Each character in the string `s` is either '0' or '1'.
Assumptions:
• The input string is valid and consists only of '0' and '1'.
• The number of operations will not exceed the time limit for strings of length up to 1000.
• Input: Input: s = '0101011'
• Explanation: After one second, the string becomes '1010110'. After another second, it becomes '1101100'. Finally, after the third second, the string becomes '1111000'. The process requires 3 seconds in total.
• Input: Input: s = '11100'
• Explanation: No occurrence of '01' exists in the string, so no swaps are needed, and the process takes 0 seconds.
Approach: We can simulate the process of replacing all occurrences of '01' with '10' using a loop. After each iteration, we count the number of swaps that occurred and repeat the process until no more '01' substrings are found.
Observations:
• This problem involves repetitive operations on a string, making it suitable for simulation.
• We can solve the problem efficiently by counting the occurrences of '01' in each iteration and performing swaps until no occurrences are left.
Steps:
• 1. Initialize a counter for the seconds.
• 2. Use a while loop to keep checking for occurrences of '01'.
• 3. If '01' is found, swap it to '10' and increment the seconds counter.
• 4. Repeat the process until no '01' exists in the string.
Empty Inputs:
• The input string will never be empty.
Large Inputs:
• For large strings, ensure that the solution is optimized to avoid unnecessary recomputations.
Special Values:
• If the string already contains no '01' substrings, the answer will be 0.
Constraints:
• Ensure the solution can handle strings of length up to 1000 efficiently.
int secondsToRemoveOccurrences(string s) {
int seconds = 0;
bool changed = true;
while(changed) {
changed = false;
for(int i = 0; i < s.size() - 1; i++) {
if(s[i] == '0' && s[i + 1] == '1') {
swap(s[i], s[i + 1]);
changed = true;
i++;
}
}
seconds += changed;
}
return seconds;
}
1 : Function Definition
int secondsToRemoveOccurrences(string s) {
Defines the function `secondsToRemoveOccurrences` that takes a string `s` containing '0' and '1' characters. The function returns the number of seconds needed to remove all occurrences of '01' by swapping them into '10'.
2 : Variable Initialization
int seconds = 0;
Initializes the `seconds` variable to track the number of seconds (or iterations) taken to remove all '01' pairs in the string `s`.
3 : Flag Initialization
bool changed = true;
Initializes the `changed` flag to `true` to start the while loop, which will continue iterating as long as there are swaps performed.
4 : While Loop Start
while(changed) {
Starts the `while` loop that continues as long as any swap occurs in the current iteration (i.e., as long as `changed` is `true`).
5 : Flag Reset
changed = false;
Resets the `changed` flag to `false` at the beginning of each iteration to track whether any swap happens in this cycle.
6 : For Loop Start
for(int i = 0; i < s.size() - 1; i++) {
Starts a for loop to iterate over each character in the string `s`, up to the second-to-last character, checking for '01' pairs.
7 : Condition Check for '01' Pair
if(s[i] == '0' && s[i + 1] == '1') {
Checks if the current character `s[i]` is '0' and the next character `s[i + 1]` is '1', indicating a '01' pair that can be swapped.
8 : Swap '01' to '10'
swap(s[i], s[i + 1]);
Swaps the '0' and '1' characters in the pair, changing '01' to '10'.
9 : Flag Set
changed = true;
Sets the `changed` flag to `true` to indicate that a swap has been performed, and another iteration will be needed.
10 : Skip Next Character
i++;
Increments `i` by an extra step to skip the next character, as it has already been swapped and does not need to be checked again in this cycle.
11 : Increment Seconds
seconds += changed;
If any swap was made during the current iteration, the `seconds` counter is incremented by 1.
12 : Return Result
return seconds;
Returns the total number of seconds (iterations) taken to remove all '01' pairs in the string.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity remains O(n) even in the worst case, as each pass through the string only requires linear time to detect and replace occurrences of '01'.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) because we are modifying the string in place and using only a few extra variables for counting and controlling the loop.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus