Leetcode 2370: Longest Ideal Subsequence
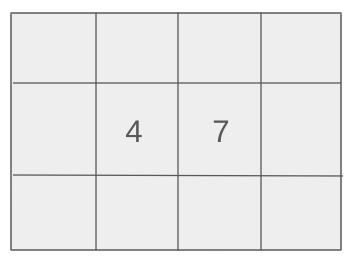
You are given a string s consisting of lowercase letters and an integer k. A string t is considered ideal if it is a subsequence of s and the absolute difference in the alphabet order of every two adjacent letters in t is less than or equal to k. Your task is to return the length of the longest ideal string.
Problem
Approach
Steps
Complexity
Input: The input consists of a string s and an integer k.
Example: s = "azbcde", k = 3
Constraints:
• 1 <= s.length <= 10^5
• 0 <= k <= 25
• s consists of lowercase English letters.
Output: Return the length of the longest ideal string that satisfies the given conditions.
Example: Output: 4
Constraints:
Goal: The goal is to compute the longest subsequence that satisfies the condition on the absolute difference between adjacent characters.
Steps:
• 1. Use dynamic programming to track the length of the longest ideal string that can be formed ending at each character.
• 2. For each character in the string, check for possible valid subsequences by considering the character itself and the preceding ones within a range defined by k.
• 3. Keep updating the maximum length of the ideal subsequence found.
Goal: The input string is guaranteed to have a length between 1 and 10^5, and each character is a lowercase letter.
Steps:
• The length of the string s is between 1 and 10^5.
• k is between 0 and 25.
• The string s only contains lowercase English letters.
Assumptions:
• The string is non-empty and consists only of lowercase English letters.
• The value of k is valid, i.e., between 0 and 25.
• Input: Input: s = "azbcde", k = 3
• Explanation: In this case, the longest ideal string is "abc", with a length of 3. The absolute difference between adjacent characters is within the given range of k = 3.
• Input: Input: s = "abc", k = 2
• Explanation: The longest ideal string is "abc", with a length of 3. The absolute difference between adjacent characters is within the given range of k = 2.
Approach: The problem can be solved using dynamic programming to track the longest ideal subsequence and check if adjacent characters meet the condition.
Observations:
• The problem can be broken down into tracking the longest subsequence at each index.
• We need to check the possible subsequences by comparing each character with those that came before it.
• Dynamic programming will allow us to efficiently compute the longest subsequence by building up from smaller subsequences.
Steps:
• 1. Initialize an array dp to store the length of the longest subsequence ending at each character.
• 2. Iterate through the string s and for each character, check the possible previous characters that can form a valid subsequence by comparing their alphabet order.
• 3. Update the dp array by considering the maximum length of subsequences that can be formed by including the current character.
Empty Inputs:
• There are no empty inputs as the string s is guaranteed to have at least one character.
Large Inputs:
• For large inputs, ensure that the dynamic programming approach performs efficiently within the given constraints.
Special Values:
• Special cases might include strings where all characters are the same, or where no valid subsequences can be formed.
Constraints:
• Ensure that the solution handles strings of maximum length (10^5) efficiently.
int longestIdealString(string s, int k) {
int dp[150] = {}, res = 0;
for(auto &i: s) {
for(int j = i - k; j <= i + k; j++)
dp[i] = max(dp[i], dp[j]);
res = max(res, ++dp[i]);
}
return res;
}
1 : Function Definition
int longestIdealString(string s, int k) {
Function definition for finding the longest ideal string. It accepts a string `s` and an integer `k` as input.
2 : Variable Initialization
int dp[150] = {}, res = 0;
Initializes a dynamic programming array `dp` of size 150 to store the longest subsequence lengths, and a variable `res` to track the maximum length found.
3 : Loop Through String
for(auto &i: s) {
Iterates through each character `i` in the input string `s`.
4 : Inner Loop
for(int j = i - k; j <= i + k; j++)
An inner loop that checks possible characters within a range of `k` difference from the current character `i`.
5 : DP Update
dp[i] = max(dp[i], dp[j]);
Updates the dp value for character `i` by considering the maximum subsequence length that can be formed by including `j`.
6 : Result Update
res = max(res, ++dp[i]);
Updates the result `res` by considering the longest subsequence formed by the current character `i`.
7 : Return Statement
return res;
Returns the maximum subsequence length found, which is the length of the longest ideal string.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the string, as we perform a linear scan of the string and update the dp array.
Best Case: O(n)
Worst Case: O(1)
Description: The space complexity is O(n), as we store the dp array to track the longest ideal subsequence.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus