Leetcode 2369: Check if There is a Valid Partition For The Array
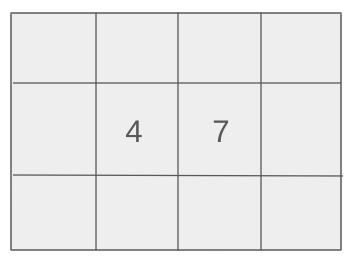
You are given a 0-indexed integer array, and you need to partition it into one or more contiguous subarrays. A partition is valid if each subarray meets one of the following conditions:
- The subarray contains exactly 2 equal elements.
- The subarray contains exactly 3 equal elements.
- The subarray contains exactly 3 consecutive increasing elements, where the difference between adjacent elements is 1. Return true if there is at least one valid partition in the array, otherwise return false.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer array, where each element represents an integer in the array.
Example: nums = [3, 3, 4, 5, 6]
Constraints:
• 2 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^6
Output: Return a boolean value: true if at least one valid partition exists, otherwise return false.
Example: Output: true
Constraints:
Goal: The goal is to check if there is any valid partition in the array that meets the conditions provided.
Steps:
• 1. Implement a dynamic programming solution to check if each possible partition is valid.
• 2. For each position in the array, check the potential subarrays of length 2 or 3 and validate them according to the given conditions.
• 3. Use memoization to store intermediate results to optimize the solution and avoid redundant computations.
Goal: The input array is guaranteed to have at least 2 elements. All array elements are integers between 1 and 10^6.
Steps:
• The length of the array is between 2 and 10^5.
• Each element in the array is between 1 and 10^6.
Assumptions:
• The array is non-empty and has at least two elements.
• There are no additional constraints such as the elements being sorted or having specific values.
• Input: Input: nums = [3, 3, 4, 5, 6]
• Explanation: In this example, the array can be partitioned into two subarrays: [3, 3] and [4, 5, 6]. Both subarrays satisfy the conditions, so the output is true.
• Input: Input: nums = [1, 1, 1, 2]
• Explanation: In this example, no valid partition exists, as no subarray meets the conditions, so the output is false.
Approach: The problem can be solved using dynamic programming to check for valid subarrays and memoization to optimize the solution.
Observations:
• We need to consider each subarray of length 2 and 3.
• The problem can be optimized using dynamic programming to store intermediate results.
• A dynamic programming approach will be efficient for this problem as it reduces the number of repetitive checks for each partition.
Steps:
• 1. Initialize a memoization table to store results of valid partitions from each index.
• 2. Loop through the array and check for possible partitions of length 2 and 3.
• 3. Use recursion with memoization to efficiently compute whether a valid partition exists starting from each index.
Empty Inputs:
• There are no empty inputs as the length of nums is guaranteed to be at least 2.
Large Inputs:
• For large inputs, ensure that the memoization technique works efficiently to avoid timeouts.
Special Values:
• Special cases could include arrays with repeating elements or consecutive elements that form valid partitions.
Constraints:
• Make sure that the input constraints are adhered to, especially for large arrays.
int n;
vector<int> nums, memo;
bool dp(int idx) {
if(idx == n) return true;
if(memo[idx] != -1) return memo[idx];
if(idx + 1 < n && nums[idx] == nums[idx + 1]) {
if(dp(idx + 2)) return memo[idx] = true;
}
if(idx + 2 < n) {
if(nums[idx] == nums[idx + 1] && nums[idx] == nums[idx + 2]) {
if(dp(idx + 3)) return memo[idx] = true;
}
if((nums[idx + 1] == nums[idx] + 1) && (nums[idx + 2] == nums[idx] + 2)) {
if(dp(idx + 3)) return memo[idx] =true;
}
}
return memo[idx] = false;
}
bool validPartition(vector<int>& nums) {
this->nums = nums;
n = nums.size();
memo.resize(n, -1);
return dp(0);
}
1 : Variable Initialization
int n;
This initializes the integer variable 'n' that will store the size of the input array 'nums'.
2 : Variable Initialization
vector<int> nums, memo;
The 'nums' vector stores the input array, while 'memo' is used to store computed results for subproblems in dynamic programming.
3 : Function Definition
bool dp(int idx) {
Defines a recursive function 'dp' that checks if a valid partition is possible starting from index 'idx'.
4 : Base Case
if(idx == n) return true;
Base case: If the index equals the size of the array, it means the entire array has been processed and is valid.
5 : Memoization
if(memo[idx] != -1) return memo[idx];
Check if the result for the current index 'idx' has already been computed. If so, return the stored value.
6 : Recursive Case - Pair
if(idx + 1 < n && nums[idx] == nums[idx + 1]) {
Checks if the current and next elements are equal, indicating a valid pair. If so, it recursively checks from the next pair.
7 : Recursive Case - Pair
if(dp(idx + 2)) return memo[idx] = true;
If the pair is valid, the function recursively checks from the index two positions ahead.
8 : Recursive Case - Triplet
if(idx + 2 < n) {
Checks if a triplet is possible starting at the current index.
9 : Recursive Case - Triplet
if(nums[idx] == nums[idx + 1] && nums[idx] == nums[idx + 2]) {
Checks if the current and the next two elements form a valid triplet (all elements equal).
10 : Recursive Case - Triplet
if(dp(idx + 3)) return memo[idx] = true;
If the triplet is valid, the function recursively checks from the index three positions ahead.
11 : Recursive Case - Incrementing Triplet
if((nums[idx + 1] == nums[idx] + 1) && (nums[idx + 2] == nums[idx] + 2)) {
Checks if the next two elements form a sequence incrementing by 1 (e.g., [x, x+1, x+2]).
12 : Recursive Case - Incrementing Triplet
if(dp(idx + 3)) return memo[idx] = true;
If the sequence is valid, the function recursively checks from the index three positions ahead.
13 : Base Case - Failure
return memo[idx] = false;
If no valid partitioning is found, return false and store the result in 'memo'.
14 : Function Definition
}
End of the 'dp' function definition.
15 : Function Definition
bool validPartition(vector<int>& nums) {
Defines the main function 'validPartition' which initializes variables and calls the 'dp' function.
16 : Function Logic
this->nums = nums;
Assigns the input vector 'nums' to the class member 'nums'.
17 : Function Logic
n = nums.size();
Sets the value of 'n' to the size of the input array 'nums'.
18 : Function Logic
memo.resize(n, -1);
Resizes the memoization vector 'memo' to the size of the array 'nums', initializing all values to -1.
19 : Function Logic
return dp(0);
Calls the 'dp' function starting from index 0 and returns the result.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the array, due to the linear traversal with memoization.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the memoization table used to store intermediate results.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus