Leetcode 2357: Make Array Zero by Subtracting Equal Amounts
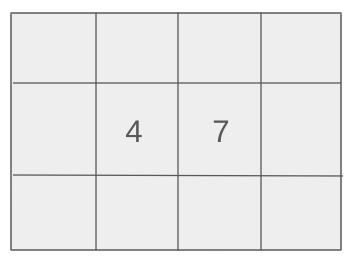
You are given a non-negative integer array nums. In each operation, you must:
- Choose a positive integer x that is less than or equal to the smallest non-zero element in nums.
- Subtract x from every positive element in nums.
Return the minimum number of operations to make every element in nums equal to 0.
Problem
Approach
Steps
Complexity
Input: The input consists of an array nums where each element is a non-negative integer.
Example: Input: nums = [1, 5, 0, 3, 5]
Constraints:
• 1 <= nums.length <= 100
• 0 <= nums[i] <= 100
Output: Return an integer representing the minimum number of operations required.
Example: Output: 3
Constraints:
• The output will always be a non-negative integer.
Goal: Sort the input array and calculate the number of operations based on distinct positive elements.
Steps:
• Sort the input array.
• Iterate over the array and count how many distinct non-zero elements exist.
• For each distinct positive element, consider an operation to reduce those elements to zero.
Goal: The size of the array is small enough (n ≤ 100) to apply sorting and iteration methods.
Steps:
• The length of nums will always be between 1 and 100.
• Each element in nums will be between 0 and 100.
Assumptions:
• All elements in nums are non-negative integers.
• At least one element in nums is positive.
• Input: Example 1: nums = [1, 5, 0, 3, 5]
• Explanation: In this case, we perform three operations: subtract 1 from all elements (resulting in [0, 4, 0, 2, 4]), subtract 2 from all elements (resulting in [0, 2, 0, 0, 2]), and finally subtract 2 again (resulting in [0, 0, 0, 0, 0]). Thus, the output is 3.
Approach: The approach is to sort the array and calculate the number of operations based on distinct positive elements in the array.
Observations:
• We can sort the array to easily track the smallest non-zero element.
• We need to perform operations for each distinct positive element in the array.
Steps:
• Sort the array of numbers.
• Iterate through the array, checking for distinct positive elements.
• For each new distinct positive number, perform an operation and increase the operation count.
Empty Inputs:
• If the array has only zeroes, the output should be 0.
Large Inputs:
• Even with the largest possible input size (100 elements), sorting and iterating through the array will be efficient enough.
Special Values:
• If all elements are zero, no operation is needed.
Constraints:
• Ensure that only positive elements are considered when counting operations.
int minimumOperations(vector<int>& nums) {
sort(nums.begin(), nums.end());
int mx = 0, res = 0, diff;
for(int i = 0; i < nums.size(); i++) {
if(mx < nums[i]) {
mx = nums[i];
res++;
}
}
return res;
}
1 : Function Definition
int minimumOperations(vector<int>& nums) {
Defines the function `minimumOperations`, which takes a vector of integers `nums` and returns an integer representing the minimum operations needed.
2 : Sorting
sort(nums.begin(), nums.end());
Sorts the vector `nums` in ascending order to facilitate comparison between the current element and the maximum element.
3 : Variable Initialization
int mx = 0, res = 0, diff;
Initializes `mx` to track the maximum number encountered, `res` to count the number of operations, and `diff` which is unused in this case.
4 : Loop
for(int i = 0; i < nums.size(); i++) {
Loops through each element of the sorted `nums` array.
5 : Condition Check
if(mx < nums[i]) {
Checks if the current number is greater than the previously encountered maximum `mx`.
6 : Update Maximum
mx = nums[i];
Updates `mx` to the current number `nums[i]` if it is greater.
7 : Increment Operation Count
res++;
Increments the operation count `res` each time a new maximum is found, implying a change operation.
8 : Return Result
return res;
Returns the total number of operations performed.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The worst-case time complexity is O(n log n) due to sorting the array.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the array being stored in memory.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus