Leetcode 2336: Smallest Number in Infinite Set
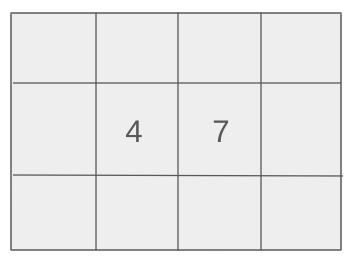
You have a set containing all positive integers starting from 1. You need to implement a class SmallestInfiniteSet that has the following methods:
- SmallestInfiniteSet() Initializes the set to contain all positive integers starting from 1.
- popSmallest() Removes and returns the smallest integer in the set.
- addBack(int num) Adds a positive integer num back into the set, if it’s not already present in the set.
Problem
Approach
Steps
Complexity
Input: The input consists of a list of method calls on the SmallestInfiniteSet class.
Example: Input: ["SmallestInfiniteSet", "addBack", "popSmallest", "popSmallest", "popSmallest"]
Output: [null, null, 1, 2, 3]
Constraints:
• 1 <= num <= 1000
• At most 1000 calls will be made in total to popSmallest and addBack.
Output: The output consists of the return values of the method calls in the input sequence.
Example: [null, null, 1, 2, 3]
Constraints:
• The output list will contain the return values of each operation in the order they were executed.
Goal: The goal is to design a set data structure that efficiently supports removing the smallest element and adding an element back into the set if it's not already there.
Steps:
• Initialize an empty set and maintain a counter to track the smallest number.
• For each popSmallest operation, check if the set is non-empty and pop the smallest number from the set.
• For each addBack operation, ensure the number is less than the current counter and not already in the set before adding it back.
Goal: The problem constraints ensure that the solution handles a maximum of 1000 calls to popSmallest and addBack.
Steps:
• The number to be added back should be a positive integer.
• The set must efficiently support operations up to 1000 times.
Assumptions:
• The smallest number in the set is always the first positive integer not yet removed or added back.
• Input: ["SmallestInfiniteSet", "addBack", "popSmallest", "popSmallest", "popSmallest"]
• Explanation: The sequence of operations ensures that the smallest integers are popped and numbers can be re-added as needed.
Approach: To solve the problem, maintain an ongoing counter to track the smallest number not yet popped. Use a set to store numbers that are added back to the infinite set.
Observations:
• The set should handle duplicate insertions gracefully and should always prioritize the smallest available number.
• We can use a counter variable that tracks the smallest number, along with a set to track any numbers that are added back to the infinite set.
Steps:
• Initialize the counter to 1 and an empty set.
• For each addBack operation, add the number to the set if it's less than the counter and not already present.
• For each popSmallest operation, either return the smallest number in the set or the current counter value and increment the counter.
Empty Inputs:
• No edge cases related to empty inputs, as the set is always initialized and can be called multiple times.
Large Inputs:
• The problem must handle up to 1000 operations efficiently.
Special Values:
• Handle the case where a number is added back multiple times or when the set has large numbers.
Constraints:
• Ensure the solution works with at most 1000 operations.
int fillCups(vector<int>& A) {
int mx = 0, sum = 0;
for(int& a: A) {
mx = max(a, mx);
sum += a;
}
return max(mx, (sum + 1) / 2);
}
1 : Code Block
int fillCups(vector<int>& A) {
Define the function 'fillCups' that takes a vector of integers A, representing the number of cups of each type.
2 : Variable Initialization
int mx = 0, sum = 0;
Initialize variables: 'mx' to store the maximum cup count, and 'sum' to store the total sum of all cups.
3 : Loop
for(int& a: A) {
Iterate over each element in the vector 'A', which represents the cup counts.
4 : Update Maximum
mx = max(a, mx);
Update the maximum cup count 'mx' with the current value 'a' if it is greater than the current maximum.
5 : Accumulate Sum
sum += a;
Add the current cup count 'a' to the total sum.
6 : Return Result
return max(mx, (sum + 1) / 2);
Return the maximum of the 'mx' (maximum cup count) or half of the total cups, rounded up, representing the minimum number of moves required.
Best Case: O(1) when the set is empty and we simply pop the counter value.
Average Case: O(1) for both popSmallest and addBack operations as they involve constant time checks.
Worst Case: O(log n) when adding back numbers to the set and when removing from the set.
Description: The time complexity is constant for most operations, but adding numbers back involves managing the set.
Best Case: O(1) if no numbers are added back.
Worst Case: O(n) where n is the number of numbers added back to the set.
Description: Space complexity depends on the number of elements in the set.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus