Leetcode 2335: Minimum Amount of Time to Fill Cups
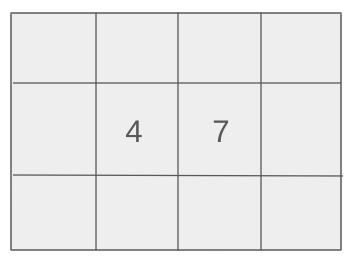
You have a water dispenser that dispenses cold, warm, and hot water. Every second, you can either fill up 2 cups with different types of water, or 1 cup of any type of water. You are given an array ‘amount’ where amount[0], amount[1], and amount[2] represent the number of cold, warm, and hot water cups you need to fill, respectively. Your task is to determine the minimum number of seconds required to fill all the cups.
Problem
Approach
Steps
Complexity
Input: The input consists of an array 'amount' of length 3, where each element represents the number of cups to be filled with cold, warm, and hot water, respectively.
Example: amount = [3, 6, 2]
Constraints:
• amount.length == 3
• 0 <= amount[i] <= 100
Output: Return the minimum number of seconds needed to fill up all the cups.
Example: 6
Constraints:
• The result should be a non-negative integer.
Goal: The goal is to minimize the number of seconds required to fill all cups, utilizing the option of filling two cups per second or one cup per second.
Steps:
• Determine the maximum cups to be filled of any type.
• Calculate the total cups to be filled (sum of all cups).
• Find the maximum time required, either by filling two cups at a time or by the total sum divided by 2.
Goal: The input has the following constraints:
Steps:
• amount.length == 3
• 0 <= amount[i] <= 100
Assumptions:
• The number of cups to fill can be zero for any type of water, meaning that filling cups of that type is not needed.
• Input: amount = [8, 4, 2]
• Explanation: By efficiently pairing the cold, warm, and hot water cups, it can be completed in 6 seconds.
Approach: To minimize the time, we should try to fill up two cups at once as much as possible, pairing different types of cups. The process is driven by the need to fill the cups with the maximum number of cups first.
Observations:
• We can reduce the total time by filling two cups at once.
• Filling up the cups with the largest difference should be prioritized.
• First calculate the maximum number of cups that need to be filled, then compute the sum of cups.
• The minimum time is either based on pairing the cups or based on the sum divided by 2.
Steps:
• Find the maximum number of cups to fill.
• Compute the sum of the cups.
• Return the maximum of the maximum cups and the half of the total sum (rounded up).
Empty Inputs:
• No cups to fill would result in 0 seconds.
Large Inputs:
• When the values in 'amount' are large, the solution must still compute the result efficiently.
Special Values:
• If any amount[i] is 0, only the remaining cups need to be filled.
Constraints:
• Handle cases with no cups or large input sizes efficiently.
int fillCups(vector<int>& A) {
int mx = 0, sum = 0;
for(int& a: A) {
mx = max(a, mx);
sum += a;
}
return max(mx, (sum + 1) / 2);
}
1 : Code Block
int fillCups(vector<int>& A) {
Define the function 'fillCups' that takes a vector of integers A, representing the number of cups of each type.
2 : Variable Initialization
int mx = 0, sum = 0;
Initialize variables: 'mx' to store the maximum cup count, and 'sum' to store the total sum of all cups.
3 : Loop
for(int& a: A) {
Iterate over each element in the vector 'A', which represents the cup counts.
4 : Update Maximum
mx = max(a, mx);
Update the maximum cup count 'mx' with the current value 'a' if it is greater than the current maximum.
5 : Accumulate Sum
sum += a;
Add the current cup count 'a' to the total sum.
6 : Return Result
return max(mx, (sum + 1) / 2);
Return the maximum of the 'mx' (maximum cup count) or half of the total cups, rounded up, representing the minimum number of moves required.
Best Case: O(1) for small inputs.
Average Case: O(1) as the problem only involves simple calculations.
Worst Case: O(1), as the solution does not involve iterating over large arrays or complex operations.
Description: The time complexity is constant.
Best Case: O(1)
Worst Case: O(1) as only a few variables are used.
Description: Space complexity is constant as the space used does not depend on input size.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus