Leetcode 2305: Fair Distribution of Cookies
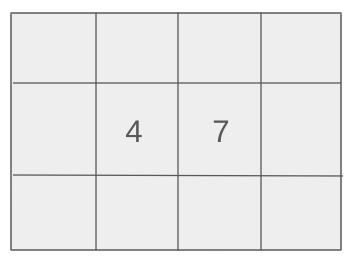
You are given an integer array cookies, where each element represents the number of cookies in a particular bag. You are also given an integer k, which denotes the number of children to distribute these bags of cookies to. Each bag must go to exactly one child, and cookies cannot be split between children. The unfairness of a distribution is defined as the maximum number of cookies any single child receives. Your goal is to return the minimum unfairness across all possible distributions.
Problem
Approach
Steps
Complexity
Input: The input consists of two parameters: cookies, an integer array representing the number of cookies in each bag, and an integer k, the number of children.
Example: cookies = [10, 20, 15, 30], k = 3
Constraints:
• 2 <= cookies.length <= 8
• 1 <= cookies[i] <= 10^5
• 2 <= k <= cookies.length
Output: Return the minimum unfairness of all possible distributions, defined as the maximum number of cookies any single child receives.
Example: For cookies = [10, 20, 15, 30] and k = 3, the output is 35.
Constraints:
• The distribution should minimize the maximum number of cookies assigned to any child.
Goal: The goal is to distribute the cookies in such a way that the maximum number of cookies any child receives is minimized.
Steps:
• Sort the cookies array for easier distribution.
• Use backtracking to try distributing cookies to each child, keeping track of the total cookies each child receives.
• Recursively explore all possible distributions and calculate the maximum unfairness for each combination.
• Return the minimum unfairness across all distributions.
Goal: The problem has constraints that ensure the array size and the number of children are manageable for backtracking.
Steps:
• 2 <= cookies.length <= 8
• 1 <= cookies[i] <= 10^5
• 2 <= k <= cookies.length
Assumptions:
• Each bag of cookies must go to exactly one child.
• All cookies in a bag must be distributed together; they cannot be split.
• Input: cookies = [10, 20, 15, 30], k = 3
• Explanation: An optimal distribution could be: [10, 20] to child 1, [15] to child 2, and [30] to child 3. The maximum number of cookies any child gets is 35.
Approach: To solve this problem, we will use backtracking to explore all possible distributions of cookies, minimizing the unfairness.
Observations:
• We need to divide the cookies into k groups such that the group with the maximum sum of cookies has the smallest possible value.
• Using backtracking, we can try every distribution and calculate the maximum cookies any child receives. We need to minimize this maximum.
Steps:
• 1. Sort the cookies array to make it easier to distribute the cookies.
• 2. Use a backtracking approach to assign cookies to k children, updating the total cookies each child receives.
• 3. Keep track of the maximum cookies received by any child and update the minimum unfairness accordingly.
• 4. Return the minimum unfairness after exploring all possible distributions.
Empty Inputs:
• Empty arrays or k greater than cookies.length should not occur as per the problem constraints.
Large Inputs:
• The solution should handle the maximum input size efficiently.
Special Values:
• If all cookies are the same, the unfairness will be minimized.
Constraints:
• Make sure the number of cookies is always greater than or equal to k.
int k, ans = INT_MAX;
vector<int> cook, dist;
int dp(int idx) {
if(idx == cook.size()) {
int sol = dist[0];
for(int i = 0; i < k; i++) {
sol = max(sol, dist[i]);
}
ans = min(ans, sol);
return sol;
}
int ans = INT_MAX;
for(int i = 0; i < k; i++) {
dist[i] += cook[idx];
ans = min(ans, dp(idx + 1));
dist[i] -= cook[idx];
}
return ans;
}
int distributeCookies(vector<int>& cook, int k) {
this->k = k;
dist.resize(k, 0);
this->cook = cook;
dp(0);
return ans;
}
1 : Variable Declaration
int k, ans = INT_MAX;
Declare two integer variables: `k` for the number of children, and `ans` for storing the minimum maximum number of cookies any child receives, initialized to `INT_MAX`.
2 : Vector Initialization
vector<int> cook, dist;
Declare two vectors: `cook`, which will store the number of cookies, and `dist`, which tracks the current distribution of cookies to the children.
3 : Function Declaration
int dp(int idx) {
Declare the recursive function `dp` that takes an integer `idx` representing the current index in the `cook` array. This function explores different ways to distribute cookies.
4 : Base Case
if(idx == cook.size()) {
Base case for recursion: if the index `idx` equals the size of the `cook` array, meaning all cookies have been distributed, calculate the maximum number of cookies a child has received.
5 : Max Distribution Calculation
int sol = dist[0];
Initialize `sol` to the number of cookies received by the first child. This will be used to track the maximum number of cookies any child has received.
6 : Loop Through Children
for(int i = 0; i < k; i++) {
Loop through each child (from `i = 0` to `i = k-1`) to find the child with the maximum number of cookies.
7 : Update Maximum
sol = max(sol, dist[i]);
Update `sol` to the maximum value between `sol` and `dist[i]`, which is the number of cookies received by the current child.
8 : Update Global Result
ans = min(ans, sol);
Update the global `ans` with the minimum of the current `ans` and `sol` to track the optimal solution (the least worst case of cookie distribution).
9 : Return Maximum Distribution
return sol;
Return the maximum number of cookies received by any child for the current distribution.
10 : Recursive Exploration
int ans = INT_MAX;
Initialize `ans` to `INT_MAX` to store the minimum value of the maximum cookies a child receives, across all possible distributions.
11 : Loop Through Children
for(int i = 0; i < k; i++) {
Loop through each child to explore different ways of distributing cookies.
12 : Update Distribution
dist[i] += cook[idx];
Add the number of cookies from `cook[idx]` to the `i`-th child's current total in `dist[i]`.
13 : Recursive Call
ans = min(ans, dp(idx + 1));
Recursively call `dp` with the next index `idx + 1`, updating `ans` with the minimum value between the current `ans` and the result of the recursive call.
14 : Undo Distribution
dist[i] -= cook[idx];
Backtrack by subtracting `cook[idx]` from `dist[i]` to undo the distribution for the current child before trying the next one.
15 : Return Minimum Maximum Distribution
return ans;
Return the minimum of the maximum distributions, which is the optimal solution for the problem.
16 : Main Function Declaration
int distributeCookies(vector<int>& cook, int k) {
Declare the main function `distributeCookies`, which takes a vector `cook` representing the number of cookies and an integer `k` for the number of children.
17 : Initialize k
this->k = k;
Assign the value of `k` to the class member variable `k`.
18 : Resize Distribution Array
dist.resize(k, 0);
Resize the `dist` array to have `k` elements, initializing all elements to 0.
19 : Initialize Cook Array
this->cook = cook;
Assign the `cook` vector to the class member variable `cook`.
20 : Call dp Function
dp(0);
Start the recursive `dp` function with index `0` to begin the distribution of cookies.
21 : Return Result
return ans;
Return the optimal result stored in `ans`, which is the minimum maximum distribution of cookies.
Best Case: O(k^n)
Average Case: O(k^n)
Worst Case: O(k^n)
Description: Since we explore all possible distributions of the cookies to k children, the time complexity depends on the number of possible assignments.
Best Case: O(k)
Worst Case: O(k)
Description: We need space for the current distribution of cookies to the k children, which takes O(k) space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus