Leetcode 2299: Strong Password Checker II
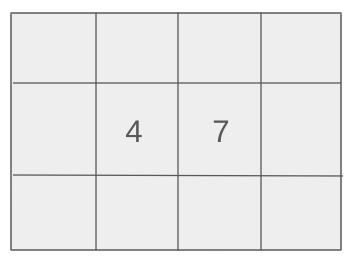
A strong password is defined by the following criteria:
- It must contain at least 8 characters.
- It must have at least one lowercase letter.
- It must have at least one uppercase letter.
- It must have at least one digit.
- It must have at least one special character from the set: ‘!@#$%^&*()-+’.
- It cannot contain two consecutive identical characters.
Given a string
password
, returntrue
if the password satisfies all these conditions. Otherwise, returnfalse
.
Problem
Approach
Steps
Complexity
Input: The input consists of a string `password` containing letters, digits, and special characters from the set: '!@#$%^&*()-+'.
Example: Input: password = 'Secure123!'
Constraints:
• 1 <= password.length <= 100
• The password consists only of letters, digits, and the special characters '!@#$%^&*()-+'.
Output: Return `true` if the password is strong according to the conditions outlined. Otherwise, return `false`.
Example: Output: true
Constraints:
Goal: To verify whether a given password meets all the criteria for being strong.
Steps:
• Step 1: Check if the password has at least 8 characters.
• Step 2: Verify that the password contains at least one lowercase letter, one uppercase letter, one digit, and one special character from the specified set.
• Step 3: Ensure that no two adjacent characters in the password are the same.
• Step 4: Return `true` if all conditions are met; otherwise, return `false`.
Goal: The password must adhere to the constraints mentioned, including having the required length, character types, and no adjacent duplicate characters.
Steps:
Assumptions:
• The password contains at least one character and does not exceed 100 characters.
• The password includes a mix of letters, digits, and special characters as per the specified set.
• Input: Input: password = 'Password123!'
• Explanation: This password meets all the criteria: it has 12 characters, includes lowercase, uppercase, a digit, a special character, and no adjacent identical characters.
• Input: Input: password = '12345abc!'
• Explanation: This password fails because it doesn't contain an uppercase letter, though it meets other conditions.
Approach: To solve this problem, we can check each condition one by one and return `false` immediately when any condition fails. If we successfully pass all checks, we return `true`.
Observations:
• We need to iterate through the password string and check for specific character types, as well as adjacent identical characters.
• The problem can be solved in a single pass through the string with constant time checks for each condition.
Steps:
• Step 1: Initialize flags for each required condition (lowercase, uppercase, digit, special character) and a variable to track previous characters.
• Step 2: Loop through the password and update the flags for each condition as needed, checking for consecutive identical characters.
• Step 3: After the loop, check all flags and ensure the password is long enough (at least 8 characters).
Empty Inputs:
• Empty strings are not valid passwords since the length must be at least 1 character.
Large Inputs:
• The solution should handle passwords of maximum length efficiently (100 characters).
Special Values:
• Passwords that consist of only one type of character (e.g., only digits or only special characters) will fail multiple checks.
Constraints:
• Ensure that all conditions are checked in O(n) time, where n is the length of the password.
bool strongPasswordCheckerII(string p) {
int lo = 0, up = 0, digit = 0, sz = p.size();
for (int i = 0; i < sz; ++i) {
if (i > 0 && p[i - 1] == p[i])
return false;
lo += islower(p[i]) ? 1 : 0;
up += isupper(p[i]) ? 1 : 0;
digit += isdigit(p[i]) ? 1 : 0;
}
return sz > 7 && lo && up && digit && (sz - lo - up - digit > 0);
}
1 : Function Declaration
bool strongPasswordCheckerII(string p) {
The function `strongPasswordCheckerII` is declared, taking a string `p` as the input, which represents the password to check. It returns a boolean value indicating whether the password meets the strength criteria.
2 : Variable Initialization
int lo = 0, up = 0, digit = 0, sz = p.size();
Four variables are initialized: `lo` to count lowercase letters, `up` to count uppercase letters, `digit` to count digits, and `sz` to store the length of the password `p`.
3 : Loop Through Password
for (int i = 0; i < sz; ++i) {
A for-loop is initiated to iterate through each character of the password `p`.
4 : Consecutive Characters Check
if (i > 0 && p[i - 1] == p[i])
The condition checks if the current character `p[i]` is the same as the previous character `p[i-1]`. If so, the password is invalid and the function returns `false`.
5 : Return False
return false;
If two consecutive characters are the same, the function immediately returns `false`, indicating the password is not strong.
6 : Lowercase Check
lo += islower(p[i]) ? 1 : 0;
The `islower` function checks if the current character `p[i]` is a lowercase letter. If true, `lo` is incremented by 1.
7 : Uppercase Check
up += isupper(p[i]) ? 1 : 0;
The `isupper` function checks if the current character `p[i]` is an uppercase letter. If true, `up` is incremented by 1.
8 : Digit Check
digit += isdigit(p[i]) ? 1 : 0;
The `isdigit` function checks if the current character `p[i]` is a digit. If true, `digit` is incremented by 1.
9 : Return Password Validity
return sz > 7 && lo && up && digit && (sz - lo - up - digit > 0);
The function returns `true` if the password length is greater than 7, contains at least one lowercase letter, one uppercase letter, one digit, and at least one special character. Otherwise, it returns `false`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the password, since we check each character once.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) because we only use a constant amount of extra space for the flags and previous character tracking.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus