Leetcode 2287: Rearrange Characters to Make Target String
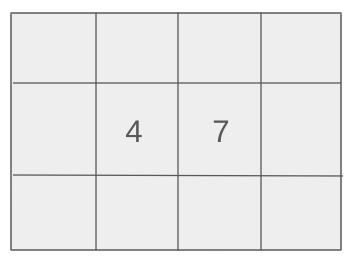
You are given two strings, s and target. Your task is to determine the maximum number of times you can rearrange the characters of s to form the string target. A character from s can only be used once in the target string, and the letters must be rearranged to form a new target string each time.
Problem
Approach
Steps
Complexity
Input: You are given two strings, s and target, where s represents a string of available characters, and target is the string you are trying to form. Both strings consist of lowercase English letters.
Example: Input: s = "helloworld", target = "low"
Constraints:
• 1 <= s.length <= 100
• 1 <= target.length <= 10
• s and target consist of lowercase English letters.
Output: Return the maximum number of times the string target can be formed by rearranging letters from s.
Example: Output: 1
Constraints:
Goal: The goal is to calculate how many full copies of the target string can be formed using characters from s.
Steps:
• Step 1: Count the frequency of each character in the target string.
• Step 2: Count the frequency of each character in the string s.
• Step 3: For each character in the target string, check how many times it can be used from s and keep track of the minimum count across all characters.
• Step 4: Return the minimum count as the result, which represents the maximum number of target strings that can be formed.
Goal: Both strings are guaranteed to have at least one character, and the characters are all lowercase English letters.
Steps:
Assumptions:
• The strings consist only of lowercase English letters.
• All characters in the target string must be present in the source string s, and we can't reuse characters from s.
• Input: Input: s = "helloworld", target = "low"
• Explanation: In this case, we can use 'l', 'o', and 'w' from s to form one copy of the target string 'low'. We can only form one copy as the string 'low' requires a specific combination of characters, and there are no extra 'w' or 'o' left to form another copy.
• Input: Input: s = "aabbcc", target = "abc"
• Explanation: Here, we can form one copy of the string 'abc' as we have exactly one 'a', one 'b', and one 'c'. The result is 1.
Approach: To solve this problem, we'll count the frequency of characters in both s and target, then calculate how many full target strings can be formed based on the availability of each character.
Observations:
• This problem is similar to counting the frequency of characters and comparing how many full sets can be made.
• The key is to keep track of how many times each character appears in both s and target.
• We need to calculate the minimum number of target strings that can be formed, which is determined by the character in the target string that is the most limiting.
Steps:
• Step 1: Create a frequency map for the target string to determine the number of each character needed.
• Step 2: Create a frequency map for the string s to determine the number of available characters.
• Step 3: For each character in the target string, divide the available characters in s by the required characters and find the minimum number across all characters.
• Step 4: Return the minimum value as the result.
Empty Inputs:
• Both s and target will not be empty as per the problem constraints.
Large Inputs:
• The algorithm should efficiently handle strings of length up to 100 for s.
Special Values:
• If s contains more characters than target, it does not affect the number of full target strings that can be formed.
Constraints:
• We only need to consider lowercase English letters.
int rearrangeCharacters(string s, string target) {
unordered_map<char,int> targetFreq ;
for(auto a : target) {
targetFreq[a] ++;
}
unordered_map<char , int> sentFreq ;
for(auto a : s) {
sentFreq[a] ++ ;
}
int mn = INT_MAX ;
for(auto a : targetFreq ) {
mn = min(mn , sentFreq[a.first]/a.second);
}
return mn ;
}
1 : Function Declaration
int rearrangeCharacters(string s, string target) {
The function `rearrangeCharacters` is declared, which takes two strings `s` and `target` as input and returns an integer representing the maximum number of times the `target` string can be formed from the characters of `s`.
2 : Initialize Target Frequency Map
unordered_map<char,int> targetFreq ;
An unordered map `targetFreq` is initialized to store the frequency of each character in the `target` string.
3 : Iterate Over Target String
for(auto a : target) {
A `for` loop iterates over each character in the `target` string to count its frequency.
4 : Update Target Frequency Map
targetFreq[a] ++;
For each character `a` in the `target` string, the frequency of that character is incremented in the `targetFreq` map.
5 : Initialize Sent Frequency Map
unordered_map<char , int> sentFreq ;
An unordered map `sentFreq` is initialized to store the frequency of each character in the `s` string.
6 : Iterate Over Sent String
for(auto a : s) {
A `for` loop iterates over each character in the `s` string to count its frequency.
7 : Update Sent Frequency Map
sentFreq[a] ++ ;
For each character `a` in the `s` string, the frequency of that character is incremented in the `sentFreq` map.
8 : Initialize Minimum Count
int mn = INT_MAX ;
The variable `mn` is initialized to `INT_MAX` to track the minimum number of times the `target` string can be formed from the characters in `s`.
9 : Check for Minimum Rearrangement
for(auto a : targetFreq ) {
A `for` loop iterates over each character-frequency pair in the `targetFreq` map.
10 : Update Minimum Count
mn = min(mn , sentFreq[a.first]/a.second);
For each character in `targetFreq`, the minimum number of times the character can be rearranged is calculated by dividing the frequency in `sentFreq` by the required frequency in `targetFreq`. The result is stored in `mn`.
11 : Return the Result
return mn ;
The function returns the value of `mn`, which represents the maximum number of times the `target` string can be formed using characters from `s`.
Best Case: O(n + m)
Average Case: O(n + m)
Worst Case: O(n + m)
Description: The time complexity is O(n + m), where n is the length of s and m is the length of target, as we need to count the frequency of each character in both strings.
Best Case: O(n + m)
Worst Case: O(n + m)
Description: The space complexity is O(n + m), where n is the length of s and m is the length of target, due to the frequency maps used for both strings.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus