Leetcode 2278: Percentage of Letter in String
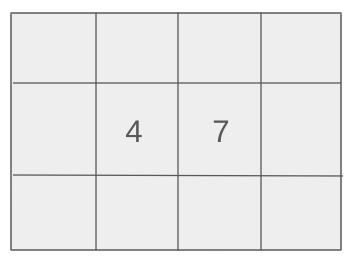
You are given a string
s
and a character letter
. Your task is to determine the percentage of characters in s
that are equal to letter
, rounding down to the nearest whole percent.Problem
Approach
Steps
Complexity
Input: You are given a string `s` and a character `letter` where `s` consists of lowercase English letters and `letter` is a lowercase English letter.
Example: Input: s = "hello", letter = "l"
Constraints:
• 1 <= s.length <= 100
• s consists of lowercase English letters.
• letter is a lowercase English letter.
Output: Return the percentage of characters in `s` that are equal to `letter`, rounded down to the nearest whole percent.
Example: Output: 40
Constraints:
Goal: Find the percentage of occurrences of `letter` in the string `s` and return it as an integer.
Steps:
• Count how many times `letter` appears in the string `s`.
• Calculate the percentage by dividing the count by the length of the string and multiplying by 100.
• Return the integer part of the percentage.
Goal: The solution must efficiently handle strings with lengths up to 100 characters.
Steps:
Assumptions:
• The string `s` is not empty.
• The character `letter` exists within the alphabet.
• Input: Input: s = "hello", letter = "l"
• Explanation: In this case, the letter 'l' appears 2 times out of 5 characters in the string 'hello'. The percentage is 2 / 5 * 100 = 40%, so the output is 40.
• Input: Input: s = "abcabc", letter = "a"
• Explanation: The letter 'a' appears 2 times out of 6 characters in the string 'abcabc'. The percentage is 2 / 6 * 100 = 33.33%. When rounded down, the result is 33.
Approach: To solve this problem, we will count how many times the letter appears in the string, calculate the percentage, and return the rounded down value.
Observations:
• The percentage is calculated by dividing the count of the letter by the length of the string and multiplying by 100.
• Rounding down is equivalent to using integer division in many programming languages.
• The problem is straightforward and involves basic counting and arithmetic operations.
Steps:
• Initialize a counter to track how many times the letter appears in the string.
• Use the count method or a loop to count the occurrences of `letter` in the string `s`.
• Calculate the percentage and round it down by performing integer division.
Empty Inputs:
• There are no empty inputs, as the length of the string is guaranteed to be at least 1.
Large Inputs:
• The solution should work for strings with a length of up to 100 characters.
Special Values:
• If the letter does not appear in the string, the percentage will be 0.
Constraints:
• The solution must handle up to 100 characters efficiently.
int percentageLetter(string s, char letter) {
return 100 * count(begin(s), end(s), letter) / s.size();
}
1 : Function Declaration
int percentageLetter(string s, char letter) {
This is the function header for `percentageLetter`, which takes a string `s` and a character `letter` as inputs. The function returns an integer representing the percentage of times `letter` appears in the string `s`.
2 : Return Statement
return 100 * count(begin(s), end(s), letter) / s.size();
The function uses the `count` function to determine how many times `letter` appears in the string `s`. It then multiplies this count by 100 and divides it by the total size of the string `s` to calculate the percentage of the letter's occurrences in the string.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the string `s`, because we need to iterate through the string to count the occurrences of `letter`.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) because we are using only a few variables to store the result.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus