Leetcode 2271: Maximum White Tiles Covered by a Carpet
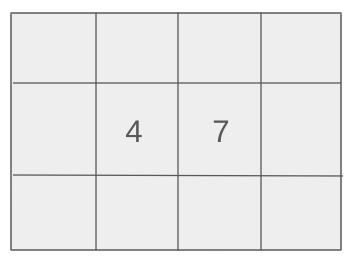
You are given a 2D integer array
tiles
, where each element tiles[i] = [li, ri]
represents that all integers from li
to ri
are painted white on a number line. Additionally, you are given an integer carpetLen
, which represents the length of a carpet that can be placed anywhere on the number line. Your task is to return the maximum number of white tiles that can be covered by placing the carpet in a way that it maximizes the number of white tiles covered.Problem
Approach
Steps
Complexity
Input: The input consists of two values: a list `tiles` containing ranges, and a number `carpetLen` representing the length of the carpet.
Example: tiles = [[1, 4], [6, 8], [12, 14]], carpetLen = 5
Constraints:
• 1 <= tiles.length <= 50,000
• tiles[i].length == 2
• 1 <= li <= ri <= 10^9
• 1 <= carpetLen <= 10^9
• The tiles are non-overlapping.
Output: Return the maximum number of white tiles that the carpet can cover.
Example: Output: 5
Constraints:
Goal: To compute the maximum number of white tiles that can be covered by placing the carpet optimally.
Steps:
• Sort the tiles by their left endpoint.
• Use a sliding window approach to check each possible placement of the carpet over the tiles, and track the maximum number of tiles covered.
• For each placement, consider whether the carpet fully or partially covers each tile, and adjust the count of white tiles covered accordingly.
Goal: Make sure the solution is efficient enough to handle large inputs and works within the provided constraints.
Steps:
• The solution should efficiently handle arrays of up to 50,000 tile ranges and large values of `li`, `ri`, and `carpetLen`.
Assumptions:
• The tiles are non-overlapping, so no two tiles will share the same number.
• Input: tiles = [[1, 5], [10, 11], [12, 18], [20, 25], [30, 32]], carpetLen = 10
• Explanation: In this example, placing the carpet starting at tile 10 will cover 9 white tiles, which is the maximum number of tiles that can be covered by a carpet of length 10.
• Input: tiles = [[1, 2], [4, 5], [7, 10]], carpetLen = 4
• Explanation: Placing the carpet from tile 4 to tile 7 covers 4 white tiles, which is the maximum number that can be covered.
Approach: This problem can be solved using a sliding window approach after sorting the tiles based on their left endpoints. By keeping track of the covered white tiles as the carpet slides over the tile ranges, we can find the maximum number of tiles that the carpet can cover.
Observations:
• The problem is about finding the optimal placement of the carpet, which requires considering each possible starting point and adjusting the number of covered tiles dynamically.
• By using a sliding window technique, we can efficiently determine the maximum coverage without needing to check each position individually.
Steps:
• Sort the tiles based on their left coordinate.
• Iterate over each possible position where the carpet can start, and compute the number of white tiles covered.
• Use a sliding window to track how many tiles are covered by the carpet for each possible starting position, ensuring that partial tiles are counted correctly.
Empty Inputs:
• This problem guarantees that the input array will contain at least one tile, so no need to handle empty input.
Large Inputs:
• Ensure that the solution works within the constraints for large inputs (up to 50,000 tiles).
Special Values:
• Tiles where the carpet length exactly matches the size of a tile or the sum of several tiles.
Constraints:
• The carpet's length should be carefully managed to ensure no overflow or inefficient calculations.
int maximumWhiteTiles(vector<vector<int>>& t, int len) {
int n = t.size(), res = 0,
cover = 0, j = 0, partial = 0;
sort(t.begin(), t.end());
for(int i = 0; i < n && res < len ; ) {
if(t[j][0] + len > t[i][1]) {
cover += t[i][1] - t[i][0] + 1;
res = max(res, cover);
i++;
} else {
partial = max(0, t[j][0] + len - t[i][0]);
res = max(res, cover + partial);
cover -= (t[j][1] - t[j][0] + 1);
j++;
}
}
return res;
}
1 : Function Declaration
int maximumWhiteTiles(vector<vector<int>>& t, int len) {
This is the function header for `maximumWhiteTiles`, which takes a 2D vector `t` representing intervals and an integer `len` representing the target length, and returns the maximum number of white tiles covered.
2 : Variable Initialization
int n = t.size(), res = 0,
Initializes `n` to store the size of the input intervals `t`, and initializes `res` to store the result (maximum number of white tiles covered).
3 : Variable Initialization
cover = 0, j = 0, partial = 0;
Initializes `cover` to keep track of the current covered area, `j` as the pointer for the second interval, and `partial` to store partial coverage for the current interval.
4 : Sort Operation
sort(t.begin(), t.end());
Sorts the intervals in ascending order based on the starting position to facilitate the greedy approach of checking coverage.
5 : Loop Start for Coverage Calculation
for(int i = 0; i < n && res < len ; ) {
Starts a loop where we iterate through the intervals, ensuring the total coverage does not exceed the target `len`.
6 : Condition Check for Full Interval Coverage
if(t[j][0] + len > t[i][1]) {
Checks if the current interval's end exceeds the target length `len` by comparing `t[j][0] + len` to `t[i][1]`. If true, it means the interval is fully covered.
7 : Cover Update
cover += t[i][1] - t[i][0] + 1;
Updates `cover` by adding the length of the current interval, from `t[i][0]` to `t[i][1]`.
8 : Max Result Update
res = max(res, cover);
Updates the result `res` to be the maximum of the current `res` and `cover`, which tracks the maximum number of tiles covered.
9 : Interval Pointer Increment
i++;
Increments the interval pointer `i` to move to the next interval.
10 : Else Block for Partial Coverage
} else {
If the current interval is not fully covered, we calculate the partial coverage.
11 : Partial Coverage Calculation
partial = max(0, t[j][0] + len - t[i][0]);
Calculates the partial coverage for the current interval, ensuring that the coverage does not go below zero.
12 : Max Result Update
res = max(res, cover + partial);
Updates the result `res` with the maximum of the current `res` and the sum of `cover` and `partial` coverage.
13 : Cover Update
cover -= (t[j][1] - t[j][0] + 1);
Decreases `cover` by the length of the interval that is no longer part of the current coverage.
14 : Interval Pointer Increment
j++;
Increments the second interval pointer `j` to move to the next interval.
15 : Return Statement
return res;
Returns the result `res`, which is the maximum number of white tiles covered.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which takes O(n log n) where `n` is the number of tiles.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage needed for the tiles array and any additional variables used for tracking the carpet placement.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus