Leetcode 2270: Number of Ways to Split Array
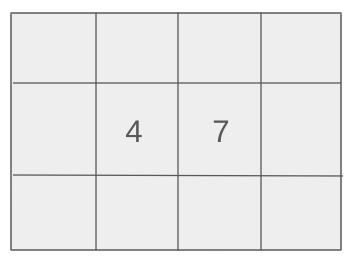
You are given an integer array
nums
of length n
. The task is to find how many valid splits exist in nums
. A valid split is defined as an index i
such that the sum of the first i + 1
elements is greater than or equal to the sum of the remaining elements from index i + 1
to the end. The split must be at an index where at least one element exists on the right side of i
.Problem
Approach
Steps
Complexity
Input: You are given an array `nums` of integers.
Example: nums = [5, 3, -2, 4]
Constraints:
• 2 <= nums.length <= 10^5
• -10^5 <= nums[i] <= 10^5
Output: Return the number of valid splits in `nums`.
Example: Output: 3
Constraints:
Goal: To calculate the number of valid splits where the sum of elements from index 0 to `i` is greater than or equal to the sum of elements from index `i + 1` to the end of the array.
Steps:
• Compute the prefix sum array to track cumulative sums from the start.
• Check each potential split point `i` from 0 to `n - 2` to see if the sum of the first `i + 1` elements is greater than or equal to the sum of the remaining elements.
• Count the number of valid splits where the condition holds.
Goal: Ensure that the solution works efficiently within the constraints provided.
Steps:
• The input array `nums` must be of size between 2 and 100,000.
• The array elements `nums[i]` must lie between -100,000 and 100,000.
Assumptions:
• The array `nums` will always contain at least two elements.
• Input: nums = [5, 3, -2, 4]
• Explanation: There are three possible splits: at index 0, index 1, and index 2. The valid splits occur at index 0 and 1, because the sum of elements before these indices is greater than or equal to the sum of elements after them.
• Input: nums = [1, 2, 3, 4, 5]
• Explanation: Valid splits happen at indices 0, 1, 2, and 3 because the sums of the first `i+1` elements at each of these indices are greater than or equal to the sums of the elements after them.
Approach: The approach uses a prefix sum array to efficiently calculate the sum of elements on the left and right sides of each potential split point. By comparing the sum of elements before the split with the sum of elements after the split, we can determine if a split is valid.
Observations:
• The problem involves comparing sums at each index, so calculating cumulative sums can speed up the solution.
• Prefix sum arrays allow us to compute the sum of elements efficiently without recalculating sums repeatedly.
Steps:
• Calculate the prefix sum array, where each element at index `i` contains the sum of the elements in `nums` from index 0 to `i`.
• For each potential split point `i`, calculate the sum of the first `i + 1` elements and the sum of the remaining elements after `i`.
• Count the number of valid splits where the sum of the first part is greater than or equal to the sum of the second part.
Empty Inputs:
• The problem guarantees that the length of `nums` will always be at least 2.
Large Inputs:
• Ensure the solution can handle large input arrays up to 100,000 elements.
Special Values:
• Arrays with all elements being the same value.
Constraints:
• The solution should handle both positive and negative integers efficiently.
int waysToSplitArray(vector<int>& in) {
// partial_sum(nums.begin(), nums.end(), nums.begin());
int n = in.size(), res = 0;
vector<long long> nums(n, 0);
for(int i = 0; i < n; i++)
nums[i] = (i == 0) ? in[0] : nums[i - 1] + in[i];
for(int i = 0; i < n - 1; i++) {
if(nums[i] >= nums[n - 1] - nums[i]) res++;
}
return res;
}
1 : Function Declaration
int waysToSplitArray(vector<int>& in) {
This is the function header for `waysToSplitArray`, which takes a vector of integers `in` and returns the number of valid splits where the sum of the left part is greater than or equal to the sum of the right part.
2 : Variable Initialization
int n = in.size(), res = 0;
Initializes `n` to store the size of the input array `in` and `res` to store the count of valid splits.
3 : Prefix Sum Array Initialization
vector<long long> nums(n, 0);
Creates a vector `nums` of size `n` to store the prefix sum values of the array.
4 : Prefix Sum Calculation
for(int i = 0; i < n; i++)
Starts a loop to calculate the prefix sum of the input array and store it in the `nums` vector.
5 : Prefix Sum Calculation Logic
nums[i] = (i == 0) ? in[0] : nums[i - 1] + in[i];
Calculates the prefix sum for each index `i`. If `i` is 0, `nums[i]` is just the first element of `in`. Otherwise, it adds the current element of `in` to the previous value in `nums`.
6 : Loop Start for Checking Splits
for(int i = 0; i < n - 1; i++) {
Starts a loop to check possible splits of the array from index `0` to `n-2`.
7 : Split Condition Check
if(nums[i] >= nums[n - 1] - nums[i]) res++;
Checks if the sum of the left part of the array (i.e., `nums[i]`) is greater than or equal to the sum of the right part (i.e., `nums[n - 1] - nums[i]`). If the condition is true, increments `res`.
8 : Return Statement
return res;
Returns the result `res`, which is the total number of valid splits where the left sum is greater than or equal to the right sum.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) where `n` is the length of the input array `nums`. We only need one pass to compute the prefix sum and another pass to count valid splits.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because we need to store the prefix sum array of length `n`.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus