Leetcode 2256: Minimum Average Difference
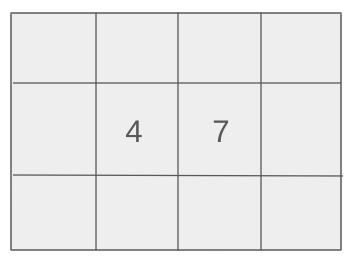
You are given a 0-indexed integer array
nums
of length n
. For each index i
, calculate the absolute difference between the average of the first i + 1
elements and the average of the last n - i - 1
elements. Both averages should be rounded down to the nearest integer. Your task is to find the index i
with the minimum average difference. If there are multiple indices with the same difference, return the smallest index.Problem
Approach
Steps
Complexity
Input: The input consists of a single integer array `nums` representing the list of integers.
Example: nums = [8, 2, 5, 6, 7, 3]
Constraints:
• 1 <= nums.length <= 10^5
• 0 <= nums[i] <= 10^5
Output: Return the index with the minimum average difference.
Example: Output: 2
Constraints:
Goal: Identify the index that results in the smallest average difference, considering the left and right subarrays formed by dividing the array at each index.
Steps:
• Compute the sum of the entire array `nums`.
• Iterate through each index `i` and calculate the average of the first `i + 1` elements and the last `n - i - 1` elements.
• For each index, compute the absolute difference between the two averages.
• Keep track of the minimum difference and the corresponding index.
• Return the index with the smallest difference. In case of a tie, return the smallest index.
Goal: Ensure that the index calculations are performed efficiently, especially for large arrays.
Steps:
• The array size `n` can go up to 100,000, so the solution must be optimized for large inputs.
• Handle cases where the array has only one element.
Assumptions:
• The array `nums` is non-empty.
• If the array has only one element, return 0 since there's no right subarray.
• Input: nums = [8, 2, 5, 6, 7, 3]
• Explanation: For each index `i`, calculate the average of the first `i + 1` elements and the last `n - i - 1` elements, and find the index with the smallest difference in averages.
• Input: nums = [10]
• Explanation: With a single element, the average difference is 0. Therefore, the result is index 0.
Approach: The problem can be solved by computing prefix and suffix sums efficiently to avoid recalculating sums repeatedly for each index.
Observations:
• We need to calculate averages for subarrays efficiently. Prefix sums will help us avoid recomputing sums for each subarray.
• This problem requires iterating over all indices in the array and comparing average differences.
• We can compute the total sum of the array and update the sums for the left and right subarrays incrementally as we move through the array.
Steps:
• Compute the total sum of `nums`.
• Initialize variables for the left sum and right sum.
• Iterate through each index `i`, updating the left sum and right sum incrementally.
• At each index, calculate the averages and their difference.
• Track the smallest difference and the corresponding index.
Empty Inputs:
• If `nums` contains only one element, return index 0.
Large Inputs:
• For arrays with 100,000 elements, ensure the solution runs efficiently by using prefix and suffix sums.
Special Values:
• If all elements are the same, the average difference will always be 0.
Constraints:
• Handle cases where `nums` contains large numbers or a large number of elements efficiently.
int minimumAverageDifference(vector<int>& nums) {
typedef long long ll;
ll sum = 0;
for(int a : nums) sum += a;
ll n = nums.size(), res = INT_MAX, ret = 0, la = 0, ra = 0;
ll l = 0, r = sum;
for(int i = 0; i < n; i++) {
int prv = res;
l += nums[i];
r -= nums[i];
la = l / (i + 1);
ra = (i+1==n)?0:r / (n-(i+1));
res = min(res, abs(la - ra));
if(prv != res) ret = i;
}
return ret;
}
1 : Function Definition
int minimumAverageDifference(vector<int>& nums) {
The function 'minimumAverageDifference' is defined here, which takes a vector of integers 'nums' as input and returns the index where the absolute difference between the left and right average is minimized.
2 : Type Definition
typedef long long ll;
The type 'll' is defined as 'long long' to handle large integer values without overflow.
3 : Variable Initialization
ll sum = 0;
The variable 'sum' is initialized to zero. It will store the total sum of the elements in the 'nums' vector.
4 : Sum Calculation
for(int a : nums) sum += a;
This loop iterates through the vector 'nums' and adds each element to 'sum', calculating the total sum of all elements.
5 : Variable Initialization
ll n = nums.size(), res = INT_MAX, ret = 0, la = 0, ra = 0;
Here, 'n' stores the size of the 'nums' vector. 'res' is initialized to the maximum integer value to track the minimum average difference. 'ret' stores the index with the minimum difference, and 'la' and 'ra' store the left and right averages, respectively.
6 : Left and Right Sums
ll l = 0, r = sum;
Variables 'l' and 'r' are initialized to zero and 'sum', respectively. 'l' will track the cumulative sum from the left, while 'r' starts with the total sum and will decrease as we move through the array.
7 : Iterate Through Elements
for(int i = 0; i < n; i++) {
A loop that iterates through each element in the vector 'nums', adjusting the left and right sums and calculating the averages at each step.
8 : Store Previous Result
int prv = res;
Stores the previous result in 'prv' for comparison later to track if the minimum result has changed.
9 : Update Left Sum
l += nums[i];
Adds the current element 'nums[i]' to the left sum 'l'.
10 : Update Right Sum
r -= nums[i];
Subtracts the current element 'nums[i]' from the right sum 'r'.
11 : Calculate Left Average
la = l / (i + 1);
Calculates the average of the left part by dividing 'l' (the sum of the left part) by the number of elements processed so far (i+1).
12 : Calculate Right Average
ra = (i+1==n)?0:r / (n-(i+1));
Calculates the average of the right part by dividing 'r' (the sum of the remaining elements) by the number of elements left (n-(i+1)). If we're at the last element, the right average is set to zero.
13 : Update Minimum Difference
res = min(res, abs(la - ra));
Updates 'res' to the minimum of its current value and the absolute difference between the left and right averages.
14 : Update Index of Minimum Difference
if(prv != res) ret = i;
If the result has changed, it updates 'ret' with the current index 'i', which now holds the minimum average difference.
15 : Return Result
return ret;
Returns 'ret', which is the index where the absolute difference between the left and right average is minimized.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The solution only requires a single iteration through the array to compute the necessary sums and differences.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant, as we only use a few variables to keep track of sums and the minimum difference.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus