Leetcode 2239: Find Closest Number to Zero
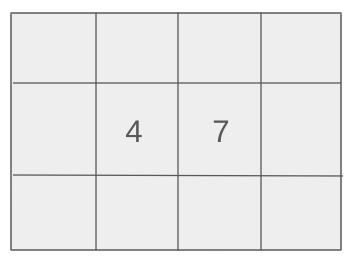
Given an array of integers, find the number closest to 0. If there are multiple numbers equally close, return the largest number among them.
Problem
Approach
Steps
Complexity
Input: An integer array nums of size n where each element can be negative, positive, or zero.
Example: Input: nums = [-7, 2, 3, -3, 5]
Constraints:
• 1 <= n <= 1000
• -105 <= nums[i] <= 105
Output: Return the integer from the array that is closest to 0. If multiple numbers are equally close, return the largest value.
Example: Output: -3
Constraints:
• The output must be a single integer from the input array.
Goal: Find the number closest to 0 based on its absolute value. If two numbers have the same absolute value, select the larger one.
Steps:
• 1. Iterate through all elements in the array.
• 2. Compare the absolute values of each number to determine the closest to 0.
• 3. If two numbers have the same absolute value, select the one with the larger value.
• 4. Return the resulting number.
Goal: The input array will always have at least one element, and each number is within the specified range.
Steps:
• 1 <= n <= 1000
• -105 <= nums[i] <= 105
Assumptions:
• The input array will never be empty.
• All values in the array are integers.
• Input: Input: nums = [-7, 2, 3, -3, 5]
• Explanation: The absolute values are [7, 2, 3, 3, 5]. Both 3 and -3 are equally close to 0, but 3 is larger. So, the output is 3.
• Input: Input: nums = [4, -4, 1]
• Explanation: The absolute values are [4, 4, 1]. Both 4 and -4 are equally close to 0, but 4 is larger. So, the output is 4.
Approach: Use a simple comparison of absolute values with a condition to handle ties for equally close numbers.
Observations:
• The absolute value comparison is key to determining closeness to 0.
• Handling ties by choosing the larger number ensures uniqueness in the output.
• Iterating through the array while maintaining a variable for the current closest value is efficient for solving the problem.
Steps:
• 1. Initialize a variable to hold the closest number, starting with the first element in the array.
• 2. Loop through the array to compare the absolute value of each number to the current closest value.
• 3. If a number has a smaller absolute value, update the closest number.
• 4. If a number has the same absolute value but is larger, update the closest number.
• 5. Return the final closest number.
Empty Inputs:
• Not applicable, as input size is guaranteed to be at least 1.
Large Inputs:
• An array with 1000 elements containing a mix of large positive and negative numbers, e.g., nums = [-105, 105, -50, 50].
Special Values:
• Array contains zeros, e.g., nums = [0, -1, 1].
• Array contains duplicate values, e.g., nums = [-5, 5, -5].
Constraints:
• All integers in the array should be within the range -105 to 105.
int findClosestNumber(vector<int>& nums) {
return *min_element(begin(nums), end(nums), [](int a, int b) {
return abs(a) < abs(b) || (abs(a) == abs(b) && a > b);
});
}
1 : Function Definition
int findClosestNumber(vector<int>& nums) {
This is the definition of the 'findClosestNumber' function that takes a vector of integers and returns an integer, which is the closest to zero.
2 : Finding Minimum Element
return *min_element(begin(nums), end(nums), [](int a, int b) {
This line uses the 'min_element' algorithm to find the closest number to zero in the array, using a lambda function to compare absolute values.
3 : Comparison Logic
return abs(a) < abs(b) || (abs(a) == abs(b) && a > b);
The lambda function compares two integers by their absolute value. If the absolute values are equal, it returns the larger number.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear since the array is traversed once.
Best Case: O(1)
Worst Case: O(1)
Description: No additional space is used beyond a few variables for tracking the result.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus