Leetcode 2235: Add Two Integers
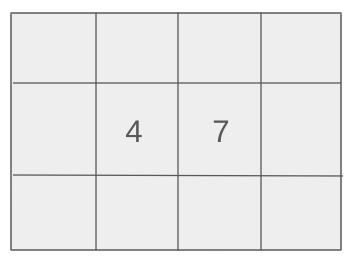
Given two integers, calculate and return their sum.
Problem
Approach
Steps
Complexity
Input: The input consists of two integers num1 and num2.
Example: num1 = 8, num2 = -3
Constraints:
• -100 <= num1, num2 <= 100
Output: Return the sum of num1 and num2 as an integer.
Example: Output: 5
Constraints:
• The result will always be within the range of a 32-bit signed integer.
Goal: Compute the sum of two integers using basic arithmetic.
Steps:
• 1. Take the two input integers, num1 and num2.
• 2. Compute their sum using the addition operator.
• 3. Return the resulting sum.
Goal: The integers should fall within the range specified in the constraints.
Steps:
• Both num1 and num2 are guaranteed to be integers within the range -100 to 100.
Assumptions:
• The input will always consist of two valid integers.
• Input: Input: num1 = 8, num2 = -3
• Explanation: The sum of 8 and -3 is 5, so the output is 5.
• Input: Input: num1 = 20, num2 = 15
• Explanation: The sum of 20 and 15 is 35, so the output is 35.
• Input: Input: num1 = -50, num2 = -25
• Explanation: The sum of -50 and -25 is -75, so the output is -75.
Approach: A straightforward approach involves using the addition operator to compute the sum of the two integers.
Observations:
• The problem requires basic arithmetic.
• The constraints ensure the input values are manageable.
• A simple addition operation is sufficient to solve this problem.
Steps:
• 1. Receive the two integers as input.
• 2. Use the addition operator to calculate their sum.
• 3. Return the computed sum as the output.
Empty Inputs:
• Not applicable, as input is guaranteed to include two integers.
Large Inputs:
• Not applicable, as the input range is limited to -100 to 100.
Special Values:
• Input values at the edge of the range, e.g., -100 and 100.
Constraints:
• Ensure the solution works for both positive and negative integers.
int sum(int num1, int num2) {
return num1 + num2;
}
1 : Function Definition
int sum(int num1, int num2) {
Defines the function 'sum' that takes two integer parameters, 'num1' and 'num2', and will return their sum.
2 : Return Statement
return num1 + num2;
Returns the sum of 'num1' and 'num2' as the result of the function.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The addition operation has constant time complexity.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant, as no additional memory is used apart from the input and output.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus