Leetcode 2232: Minimize Result by Adding Parentheses to Expression
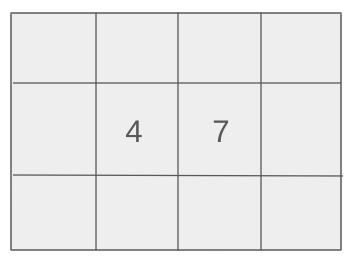
You are given a string expression in the form ‘+’, where and represent positive integers. Your task is to add a pair of parentheses to the expression such that the resulting expression evaluates to the smallest possible value. The parentheses must be placed around the ‘+’ operator. If there are multiple valid solutions yielding the same result, any of them can be returned.
Problem
Approach
Steps
Complexity
Input: The input consists of a string expression containing exactly one '+' symbol, with digits from '1' to '9'.
Example: expression = '345+56'
Constraints:
• 3 <= expression.length <= 10
• expression consists of digits from '1' to '9' and a single '+'
• expression starts and ends with digits
Output: Return the expression after adding a pair of parentheses such that the result of the expression is minimized.
Example: Output: '3(45+56)'
Constraints:
• The result fits within a signed 32-bit integer.
Goal: The goal is to minimize the expression by placing parentheses around the '+' operator. To achieve this, we need to consider the placement of parentheses that minimizes the product after the addition.
Steps:
• 1. Find the position of the '+' symbol in the expression.
• 2. Try different placements of the parentheses around the '+' and calculate the resulting value.
• 3. Return the expression that yields the smallest result.
Goal: The solution must handle up to 10 characters in the input expression.
Steps:
• expression contains exactly one '+'
• expression contains only digits from '1' to '9'
• expression length is between 3 and 10 characters
Assumptions:
• The expression is always a valid mathematical string with exactly one '+' symbol.
• The length of the expression is guaranteed to be between 3 and 10 characters.
• Input: Input: expression = '345+56'
• Explanation: In this case, we can place parentheses around '345' and '56', resulting in '3(45+56)', which evaluates to 3 * (45 + 56) = 3 * 101 = 303, which is the smallest possible value.
• Input: Input: expression = '123+45'
• Explanation: Here, placing parentheses around '12' and '45' results in '1(23+45)' which evaluates to 1 * (23 + 45) = 1 * 68 = 68, the smallest value.
• Input: Input: expression = '100+200'
• Explanation: For this case, the expression '100+200' becomes '(100+200)', which evaluates to 300, the only possible solution.
Approach: The approach is to explore various positions for the parentheses around the '+' symbol and calculate the resulting expressions. We then select the one that produces the smallest value.
Observations:
• The parentheses need to be placed around the '+' symbol to affect the addition operation.
• We can use brute force to check all possible valid parentheses placements and calculate the result to find the minimum.
Steps:
• 1. Identify the position of the '+' symbol in the expression.
• 2. Split the expression into two parts based on the '+' symbol.
• 3. Test different placements of parentheses around the '+' symbol.
• 4. Calculate the result for each placement and keep track of the smallest result.
• 5. Return the expression that produces the smallest result.
Empty Inputs:
• There are no empty inputs as the problem guarantees valid expressions.
Large Inputs:
• Since the length of the input is limited to 10 characters, handling large inputs is not an issue.
Special Values:
• The '+' symbol may be at different positions in the expression, but this doesn't affect the approach.
Constraints:
• The expression always contains exactly one '+' symbol.
string minimizeResult(string exp) {
int pos = -1;
int x = 0, y = 0, p = 0, q = 0;
for(int i = 0; i < exp.size(); i++) {
if(exp[i] == '+') pos = i;
if(pos == -1) {
y = y * 10 + exp[i] - '0';
} else if (exp[i] != '+'){
q = q * 10 + exp[i] - '0';
}
}
int tmp = q;
// cout << y << " " << pos << " " << q << "\n";
vector<int> res = {-1, -1}; int ans = INT_MAX;
for(int i = 0; i < pos; i++) {
x = x * 10 + (i > 0? exp[i - 1] - '0': 0);
y = y % (int)pow(10, pos - i);
for(int j = pos + 1; j < exp.size(); j++) {
p = p * 10 + (exp[j] - '0');
q= q % (int)pow(10, exp.size() - 1 - j);
// cout << x << " " << y << " " << p << " " << q << " " << ans << "\n";
if((x==0?1:x) * (y + p) * (q==0?1:q) < ans) {
ans = (x==0?1:x) * (y + p) * (q==0?1:q);
// cout << x << " " << y << " " << p << " " << q << " " << ans << "\n";
res = {i, j};
}
}
p = 0;
q = tmp;
}
// cout << res[0] << " " << res[1];
string ret = "";
int i = 0;
while(i < res[0]) ret += exp[i++];
ret += '(';
while(i <= res[1]) ret += exp[i++];
ret += ')';
while(i < exp.size()) ret += exp[i++];
return ret;
}
1 : Function Definition
string minimizeResult(string exp) {
Defines the function 'minimizeResult', which takes a string representing a mathematical expression and returns the minimized result after placing parentheses optimally.
2 : Variable Initialization
int pos = -1;
Initializes the variable 'pos' to -1, which will store the index of the '+' operator in the expression.
3 : Variable Initialization
int x = 0, y = 0, p = 0, q = 0;
Initializes variables 'x', 'y', 'p', and 'q' to 0. These variables will be used to break down and calculate parts of the expression.
4 : Looping Through Expression
for(int i = 0; i < exp.size(); i++) {
Starts a loop to iterate through each character of the expression string 'exp'.
5 : Condition Check
if(exp[i] == '+') pos = i;
Checks if the current character is a '+' operator and stores its position in 'pos'.
6 : Condition Check
if(pos == -1) {
Checks if the '+' operator hasn't been encountered yet.
7 : Mathematical Operations
y = y * 10 + exp[i] - '0';
If no '+' operator is found, the character is added to the variable 'y' by converting it to an integer.
8 : Condition Check
} else if (exp[i] != '+'){
If the character is not a '+', it is part of the second number after the '+' operator.
9 : Mathematical Operations
q = q * 10 + exp[i] - '0';
Adds the current character to the variable 'q', representing the second number in the expression.
10 : Variable Assignment
int tmp = q;
Stores the value of 'q' into 'tmp' to preserve its original value for later use.
11 : Variable Initialization
vector<int> res = {-1, -1}; int ans = INT_MAX;
Initializes a vector 'res' to store the optimal indices for placing parentheses, and initializes 'ans' to a large value (INT_MAX) to track the minimum result.
12 : Looping Through Expression
for(int i = 0; i < pos; i++) {
Starts a loop to iterate through the expression before the '+' operator.
13 : Mathematical Operations
x = x * 10 + (i > 0? exp[i - 1] - '0': 0);
Calculates the first part of the first number in the expression by adding digits before the '+' operator.
14 : Mathematical Operations
y = y % (int)pow(10, pos - i);
Calculates the remaining part of the second number after the '+' operator.
15 : Looping Through Expression
for(int j = pos + 1; j < exp.size(); j++) {
Starts a nested loop to iterate through the expression after the '+' operator.
16 : Mathematical Operations
p = p * 10 + (exp[j] - '0');
Adds the current character in the second part of the expression (after the '+') to the variable 'p'.
17 : Mathematical Operations
q= q % (int)pow(10, exp.size() - 1 - j);
Calculates the remaining part of 'q' after the current digit is processed.
18 : Condition Check
if((x==0?1:x) * (y + p) * (q==0?1:q) < ans) {
Checks if the current combination of 'x', 'y', 'p', and 'q' produces a smaller result.
19 : Variable Assignment
ans = (x==0?1:x) * (y + p) * (q==0?1:q);
Updates the minimum result 'ans' if the current combination yields a smaller value.
20 : Variable Assignment
res = {i, j};
Stores the indices of the optimal parentheses placement in 'res'.
21 : Returning Result
string ret = "";
Initializes an empty string 'ret' to store the result.
22 : Building Result String
int i = 0;
Initializes an index 'i' to start building the result string.
23 : Building Result String
while(i < res[0]) ret += exp[i++];
Adds the part before the first parenthesis to 'ret'.
24 : Building Result String
ret += '(';
Adds the opening parenthesis to 'ret'.
25 : Building Result String
while(i <= res[1]) ret += exp[i++];
Adds the part inside the parentheses to 'ret'.
26 : Building Result String
ret += ')';
Adds the closing parenthesis to 'ret'.
27 : Building Result String
while(i < exp.size()) ret += exp[i++];
Adds the remaining part of the expression to 'ret'.
28 : Returning Result
return ret;
Returns the final string with the parentheses placed optimally.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The algorithm performs linear passes over the expression to find the optimal placement of parentheses.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is linear due to storing intermediate results of expressions.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus